精灵绕点旋转#
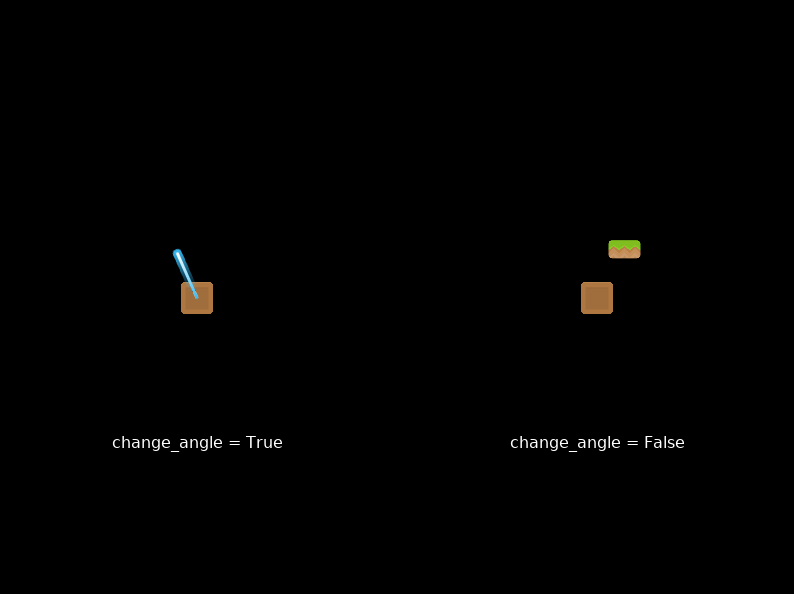
此非交互示例演示了绕点旋转精灵的两个应用程序:
在左边,一束光束绕着圆圈中的一点旋转
在右侧,平台围绕一个点旋转,同时保持平坦
虽然这一行为的灵感来自平台,但它可能对其他类型的游戏有用。
此示例中使用的基类提供了比 收集在圆圈中移动的硬币 。
有关演示如何在用户输入中混合使用点旋转的相关示例,请参见 按键盘移动,向鼠标射击 。
sprite_rotate_around_point.py#
1"""
2Rotating Sprites Around Points
3
4Two minimal examples demonstrating how to rotate sprites around points
5and how you might apply them in a game.
6
7Artwork from https://kenney.nl
8
9If Python and Arcade are installed, this example can be run from the command line with:
10python -m arcade.examples.sprite_rotate_around_point
11"""
12import arcade
13from arcade.math import rotate_point
14
15SCREEN_WIDTH = 800
16SCREEN_HEIGHT = 600
17QUARTER_WIDTH = SCREEN_WIDTH // 4
18HALF_HEIGHT = SCREEN_HEIGHT // 2
19
20
21SCREEN_TITLE = "Rotating Sprites Around Points"
22
23
24class RotatingSprite(arcade.Sprite):
25 """
26 This sprite subclass implements a generic rotate_around_point method.
27 """
28
29 def rotate_around_point(self, point, degrees, change_angle=True):
30 """
31 Rotate the sprite around a point by the set amount of degrees
32
33 You could remove the change_angle keyword and/or angle change
34 if you know that sprites will always or never change angle.
35
36 :param point: The point that the sprite will rotate about
37 :param degrees: How many degrees to rotate the sprite
38 :param change_angle: Whether the sprite's angle should also be adjusted.
39 """
40
41 # If change_angle is true, change the sprite's angle
42 if change_angle:
43 self.angle += degrees
44
45 # Move the sprite along a circle centered on the point by degrees
46 self.position = rotate_point(
47 self.center_x, self.center_y,
48 point[0], point[1], degrees)
49
50
51class ExampleWindow(arcade.Window):
52
53 def __init__(self):
54 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
55
56 self.sprites = arcade.SpriteList()
57
58 # This example is based off spinning lines of fire from Mario games
59 # See https://www.mariowiki.com/Fire_Bar for more information
60 self.left_rotating_laser_sprite = RotatingSprite(
61 ":resources:images/space_shooter/laserBlue01.png",
62 center_x=QUARTER_WIDTH + 26, center_y=HALF_HEIGHT)
63
64 self.laser_base_sprite = arcade.Sprite(
65 ":resources:images/tiles/boxCrate.png", scale=0.25,
66 center_x=QUARTER_WIDTH, center_y=HALF_HEIGHT)
67
68 self.laser_text = arcade.Text(
69 "change_angle = True",
70 QUARTER_WIDTH, SCREEN_HEIGHT // 2 - 150,
71 anchor_x='center')
72
73 # This example demonstrates how to make platforms rotate around a point
74 self.right_rotating_platform_sprite = RotatingSprite(
75 ":resources:images/tiles/grassHalf.png", scale=0.25,
76 center_x=3 * QUARTER_WIDTH + 50, center_y=HALF_HEIGHT)
77
78 self.platform_base_sprite = arcade.Sprite(
79 ":resources:images/tiles/boxCrate.png", scale=0.25,
80 center_x=3 * QUARTER_WIDTH, center_y=HALF_HEIGHT)
81
82 self.platform_text = arcade.Text(
83 "change_angle = False",
84 3 * QUARTER_WIDTH, HALF_HEIGHT - 150,
85 anchor_x='center')
86
87 self.sprites.extend([
88 self.laser_base_sprite,
89 self.left_rotating_laser_sprite,
90 self.platform_base_sprite,
91 self.right_rotating_platform_sprite])
92
93 def on_update(self, delta_time: float):
94 # Rotate the laser sprite and change its angle
95 self.left_rotating_laser_sprite.rotate_around_point(
96 self.laser_base_sprite.position,
97 120 * delta_time)
98
99 # Rotate the platform sprite but don't change its angle
100 self.right_rotating_platform_sprite.rotate_around_point(
101 self.platform_base_sprite.position,
102 60 * delta_time, False)
103
104 def on_draw(self):
105 # Draw the sprite list.
106 self.clear()
107 self.sprites.draw()
108
109 self.laser_text.draw()
110 self.platform_text.draw()
111
112
113def main():
114 window = ExampleWindow()
115 window.run()
116
117
118if __name__ == '__main__':
119 main()