收集在圆圈中移动的硬币#
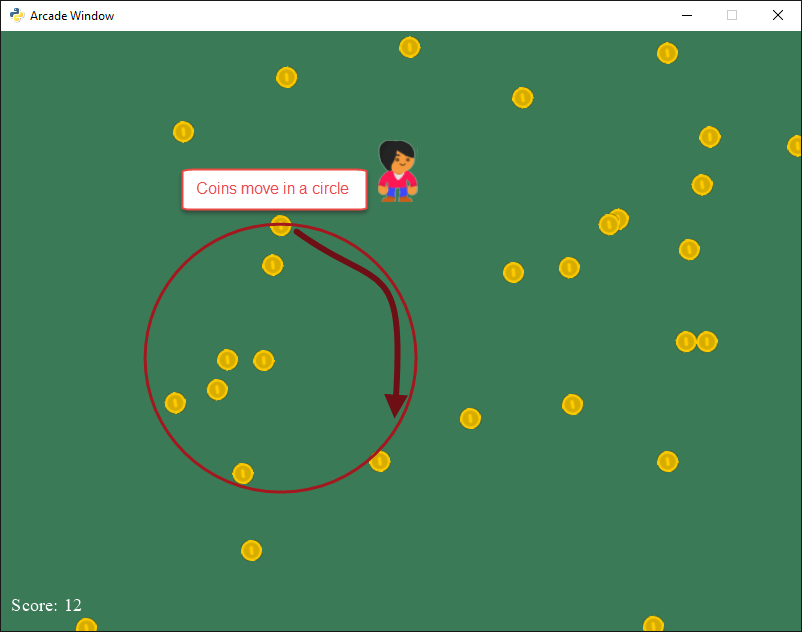
sprite_collect_coins_move_circle.py#
1"""
2Sprite Collect Coins Moving in Circles
3
4Simple program to show basic sprite usage.
5
6Artwork from https://kenney.nl
7
8If Python and Arcade are installed, this example can be run from the command line with:
9python -m arcade.examples.sprite_collect_coins_move_circle
10"""
11
12import random
13import arcade
14import math
15
16SPRITE_SCALING = 0.5
17
18SCREEN_WIDTH = 800
19SCREEN_HEIGHT = 600
20SCREEN_TITLE = "Sprite Collect Coins Moving in Circles Example"
21
22
23class Coin(arcade.Sprite):
24
25 def __init__(self, filename, scale):
26 """ Constructor. """
27 # Call the parent class (Sprite) constructor
28 super().__init__(filename, scale=scale)
29
30 # Current angle in radians
31 self.circle_angle = 0
32
33 # How far away from the center to orbit, in pixels
34 self.circle_radius = 0
35
36 # How fast to orbit, in radians per frame
37 self.circle_speed = 0.008
38
39 # Set the center of the point we will orbit around
40 self.circle_center_x = 0
41 self.circle_center_y = 0
42
43 def update(self):
44
45 """ Update the ball's position. """
46 # Calculate a new x, y
47 self.center_x = self.circle_radius * math.sin(self.circle_angle) \
48 + self.circle_center_x
49 self.center_y = self.circle_radius * math.cos(self.circle_angle) \
50 + self.circle_center_y
51
52 # Increase the angle in prep for the next round.
53 self.circle_angle += self.circle_speed
54
55
56class MyGame(arcade.Window):
57 """ Main application class. """
58
59 def __init__(self, width, height, title):
60
61 super().__init__(width, height, title)
62
63 # Sprite lists
64 self.all_sprites_list = None
65 self.coin_list = None
66
67 # Set up the player
68 self.score = 0
69 self.player_sprite = None
70
71 def start_new_game(self):
72 """ Set up the game and initialize the variables. """
73
74 # Sprite lists
75 self.all_sprites_list = arcade.SpriteList()
76 self.coin_list = arcade.SpriteList()
77
78 # Set up the player
79 self.score = 0
80 # Character image from kenney.nl
81 self.player_sprite = arcade.Sprite(
82 ":resources:images/animated_characters/female_person/femalePerson_idle.png",
83 scale=SPRITE_SCALING
84 )
85 self.player_sprite.center_x = 50
86 self.player_sprite.center_y = 70
87 self.all_sprites_list.append(self.player_sprite)
88
89 for i in range(50):
90
91 # Create the coin instance
92 # Coin image from kenney.nl
93 coin = Coin(":resources:images/items/coinGold.png", scale=SPRITE_SCALING / 3)
94
95 # Position the center of the circle the coin will orbit
96 coin.circle_center_x = random.randrange(SCREEN_WIDTH)
97 coin.circle_center_y = random.randrange(SCREEN_HEIGHT)
98
99 # Random radius from 10 to 200
100 coin.circle_radius = random.randrange(10, 200)
101
102 # Random start angle from 0 to 2pi
103 coin.circle_angle = random.random() * 2 * math.pi
104
105 # Add the coin to the lists
106 self.all_sprites_list.append(coin)
107 self.coin_list.append(coin)
108
109 # Don't show the mouse cursor
110 self.set_mouse_visible(False)
111
112 # Set the background color
113 self.background_color = arcade.color.AMAZON
114
115 def on_draw(self):
116
117 # This command has to happen before we start drawing
118 self.clear()
119
120 # Draw all the sprites.
121 self.all_sprites_list.draw()
122
123 # Put the text on the screen.
124 output = "Score: " + str(self.score)
125 arcade.draw_text(output, 10, 20, arcade.color.WHITE, 14)
126
127 def on_mouse_motion(self, x, y, dx, dy):
128 self.player_sprite.center_x = x
129 self.player_sprite.center_y = y
130
131 def on_update(self, delta_time):
132 """ Movement and game logic """
133
134 # Call update on all sprites (The sprites don't do much in this
135 # example though.)
136 self.all_sprites_list.update()
137
138 # Generate a list of all sprites that collided with the player.
139 hit_list = arcade.check_for_collision_with_list(self.player_sprite,
140 self.coin_list)
141
142 # Loop through each colliding sprite, remove it, and add to the score.
143 for coin in hit_list:
144 self.score += 1
145 coin.remove_from_sprite_lists()
146
147
148def main():
149 window = MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
150 window.start_new_game()
151 arcade.run()
152
153
154if __name__ == "__main__":
155 main()