电灯#
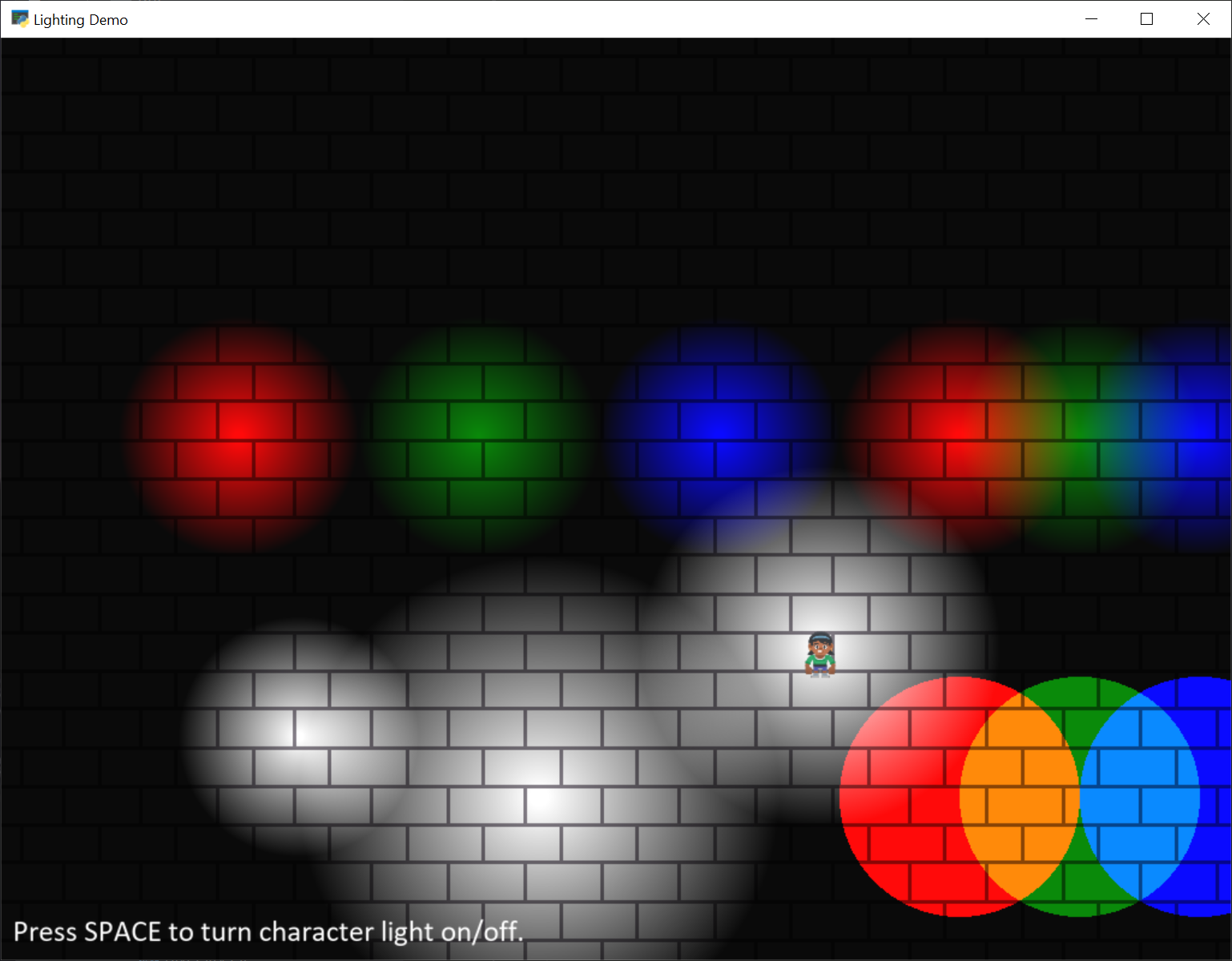
本教程需要一些文档。请随时提交公关来改进它!
light_demo.py#
1"""
2Show how to use lights.
3
4.. note:: This uses features from the upcoming version 2.4. The API for these
5 functions may still change. To use, you will need to install one of the
6 pre-release packages, or install via GitHub.
7
8Artwork from http://kenney.nl
9
10"""
11import arcade
12from arcade.experimental.lights import Light, LightLayer
13
14SCREEN_WIDTH = 1024
15SCREEN_HEIGHT = 768
16SCREEN_TITLE = "Lighting Demo"
17VIEWPORT_MARGIN = 200
18MOVEMENT_SPEED = 5
19
20# This is the color used for 'ambient light'. If you don't want any
21# ambient light, set it to black.
22AMBIENT_COLOR = (10, 10, 10)
23
24class MyGame(arcade.Window):
25 """ Main Game Window """
26
27 def __init__(self, width, height, title):
28 """ Set up the class. """
29 super().__init__(width, height, title, resizable=True)
30
31 # Sprite lists
32 self.background_sprite_list = None
33 self.player_list = None
34 self.wall_list = None
35 self.player_sprite = None
36
37 # Physics engine
38 self.physics_engine = None
39
40 # camera for scrolling
41 self.camera = None
42
43 # --- Light related ---
44 # List of all the lights
45 self.light_layer = None
46 # Individual light we move with player, and turn on/off
47 self.player_light = None
48
49 def setup(self):
50 """ Create everything """
51
52 # Create sprite lists
53 self.background_sprite_list = arcade.SpriteList()
54 self.player_list = arcade.SpriteList()
55 self.wall_list = arcade.SpriteList()
56
57 # Create player sprite
58 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png", 0.4)
59 self.player_sprite.center_x = 64
60 self.player_sprite.center_y = 270
61 self.player_list.append(self.player_sprite)
62
63 # --- Light related ---
64 # Lights must shine on something. If there is no background sprite or color,
65 # you will just see black. Therefore, we use a loop to create a whole bunch of brick tiles to go in the
66 # background.
67 for x in range(-128, 2000, 128):
68 for y in range(-128, 1000, 128):
69 sprite = arcade.Sprite(":resources:images/tiles/brickTextureWhite.png")
70 sprite.position = x, y
71 self.background_sprite_list.append(sprite)
72
73 # Create a light layer, used to render things to, then post-process and
74 # add lights. This must match the screen size.
75 self.light_layer = LightLayer(SCREEN_WIDTH, SCREEN_HEIGHT)
76 # We can also set the background color that will be lit by lights,
77 # but in this instance we just want a black background
78 self.light_layer.set_background_color(arcade.color.BLACK)
79
80 # Here we create a bunch of lights.
81
82 # Create a small white light
83 x = 100
84 y = 200
85 radius = 100
86 mode = 'soft'
87 color = arcade.csscolor.WHITE
88 light = Light(x, y, radius, color, mode)
89 self.light_layer.add(light)
90
91 # Create an overlapping, large white light
92 x = 300
93 y = 150
94 radius = 200
95 color = arcade.csscolor.WHITE
96 mode = 'soft'
97 light = Light(x, y, radius, color, mode)
98 self.light_layer.add(light)
99
100 # Create three, non-overlapping RGB lights
101 x = 50
102 y = 450
103 radius = 100
104 mode = 'soft'
105 color = arcade.csscolor.RED
106 light = Light(x, y, radius, color, mode)
107 self.light_layer.add(light)
108
109 x = 250
110 y = 450
111 radius = 100
112 mode = 'soft'
113 color = arcade.csscolor.GREEN
114 light = Light(x, y, radius, color, mode)
115 self.light_layer.add(light)
116
117 x = 450
118 y = 450
119 radius = 100
120 mode = 'soft'
121 color = arcade.csscolor.BLUE
122 light = Light(x, y, radius, color, mode)
123 self.light_layer.add(light)
124
125 # Create three, overlapping RGB lights
126 x = 650
127 y = 450
128 radius = 100
129 mode = 'soft'
130 color = arcade.csscolor.RED
131 light = Light(x, y, radius, color, mode)
132 self.light_layer.add(light)
133
134 x = 750
135 y = 450
136 radius = 100
137 mode = 'soft'
138 color = arcade.csscolor.GREEN
139 light = Light(x, y, radius, color, mode)
140 self.light_layer.add(light)
141
142 x = 850
143 y = 450
144 radius = 100
145 mode = 'soft'
146 color = arcade.csscolor.BLUE
147 light = Light(x, y, radius, color, mode)
148 self.light_layer.add(light)
149
150 # Create three, overlapping RGB lights
151 # But 'hard' lights that don't fade out.
152 x = 650
153 y = 150
154 radius = 100
155 mode = 'hard'
156 color = arcade.csscolor.RED
157 light = Light(x, y, radius, color, mode)
158 self.light_layer.add(light)
159
160 x = 750
161 y = 150
162 radius = 100
163 mode = 'hard'
164 color = arcade.csscolor.GREEN
165 light = Light(x, y, radius, color, mode)
166 self.light_layer.add(light)
167
168 x = 850
169 y = 150
170 radius = 100
171 mode = 'hard'
172 color = arcade.csscolor.BLUE
173 light = Light(x, y, radius, color, mode)
174 self.light_layer.add(light)
175
176 # Create a light to follow the player around.
177 # We'll position it later, when the player moves.
178 # We'll only add it to the light layer when the player turns the light
179 # on. We start with the light off.
180 radius = 150
181 mode = 'soft'
182 color = arcade.csscolor.WHITE
183 self.player_light = Light(0, 0, radius, color, mode)
184
185 # Create the physics engine
186 self.physics_engine = arcade.PhysicsEngineSimple(self.player_sprite, self.wall_list)
187
188 # setup camera
189 self.camera = arcade.camera.Camera2D()
190
191 def on_draw(self):
192 """ Draw everything. """
193 self.clear()
194
195 # --- Light related ---
196 # Everything that should be affected by lights gets rendered inside this
197 # 'with' statement. Nothing is rendered to the screen yet, just the light
198 # layer.
199 with self.light_layer:
200 self.background_sprite_list.draw()
201 self.player_list.draw()
202
203 # Draw the light layer to the screen.
204 # This fills the entire screen with the lit version
205 # of what we drew into the light layer above.
206 self.light_layer.draw(ambient_color=AMBIENT_COLOR)
207
208 # Now draw anything that should NOT be affected by lighting.
209 arcade.draw_text("Press SPACE to turn character light on/off.",
210 10 + self.camera.left, 10 + self.camera.bottom,
211 arcade.color.WHITE, 20)
212
213 def on_resize(self, width, height):
214 """ User resizes the screen. """
215
216 # --- Light related ---
217 # We need to resize the light layer to
218 self.light_layer.resize(width, height)
219
220 # Scroll the screen so the user is visible
221 self.scroll_screen()
222
223 def on_key_press(self, key, _):
224 """Called whenever a key is pressed. """
225
226 if key == arcade.key.UP:
227 self.player_sprite.change_y = MOVEMENT_SPEED
228 elif key == arcade.key.DOWN:
229 self.player_sprite.change_y = -MOVEMENT_SPEED
230 elif key == arcade.key.LEFT:
231 self.player_sprite.change_x = -MOVEMENT_SPEED
232 elif key == arcade.key.RIGHT:
233 self.player_sprite.change_x = MOVEMENT_SPEED
234 elif key == arcade.key.SPACE:
235 # --- Light related ---
236 # We can add/remove lights from the light layer. If they aren't
237 # in the light layer, the light is off.
238 if self.player_light in self.light_layer:
239 self.light_layer.remove(self.player_light)
240 else:
241 self.light_layer.add(self.player_light)
242
243 def on_key_release(self, key, _):
244 """Called when the user releases a key. """
245
246 if key == arcade.key.UP or key == arcade.key.DOWN:
247 self.player_sprite.change_y = 0
248 elif key == arcade.key.LEFT or key == arcade.key.RIGHT:
249 self.player_sprite.change_x = 0
250
251 def scroll_screen(self):
252 """ Manage Scrolling """
253
254 # Scroll left
255 left_boundary = self.camera.left + VIEWPORT_MARGIN
256 if self.player_sprite.left < left_boundary:
257 self.camera.left -= left_boundary - self.player_sprite.left
258
259 # Scroll right
260 right_boundary = self.camera.right - VIEWPORT_MARGIN
261 if self.player_sprite.right > right_boundary:
262 self.camera.right += self.player_sprite.right - right_boundary
263
264 # Scroll up
265 top_boundary = self.camera.top - VIEWPORT_MARGIN
266 if self.player_sprite.top > top_boundary:
267 self.camera.top += self.player_sprite.top - top_boundary
268
269 # Scroll down
270 bottom_boundary = self.camera.bottom + VIEWPORT_MARGIN
271 if self.player_sprite.bottom < bottom_boundary:
272 self.camera.bottom -= bottom_boundary - self.player_sprite.bottom
273
274 # Make sure our boundaries are integer values. While the viewport does
275 # support floating point numbers, for this application we want every pixel
276 # in the view port to map directly onto a pixel on the screen. We don't want
277 # any rounding errors.
278 self.camera.left = int(self.camera.left)
279 self.camera.bottom = int(self.camera.bottom)
280
281 self.camera.use()
282
283 def on_update(self, delta_time):
284 """ Movement and game logic """
285
286 # Call update on all sprites (The sprites don't do much in this
287 # example though.)
288 self.physics_engine.update()
289
290 # --- Light related ---
291 # We can easily move the light by setting the position,
292 # or by center_x, center_y.
293 self.player_light.position = self.player_sprite.position
294
295 # Scroll the screen so we can see the player
296 self.scroll_screen()
297
298
299if __name__ == "__main__":
300 window = MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
301 window.setup()
302 arcade.run()