单人纸牌#
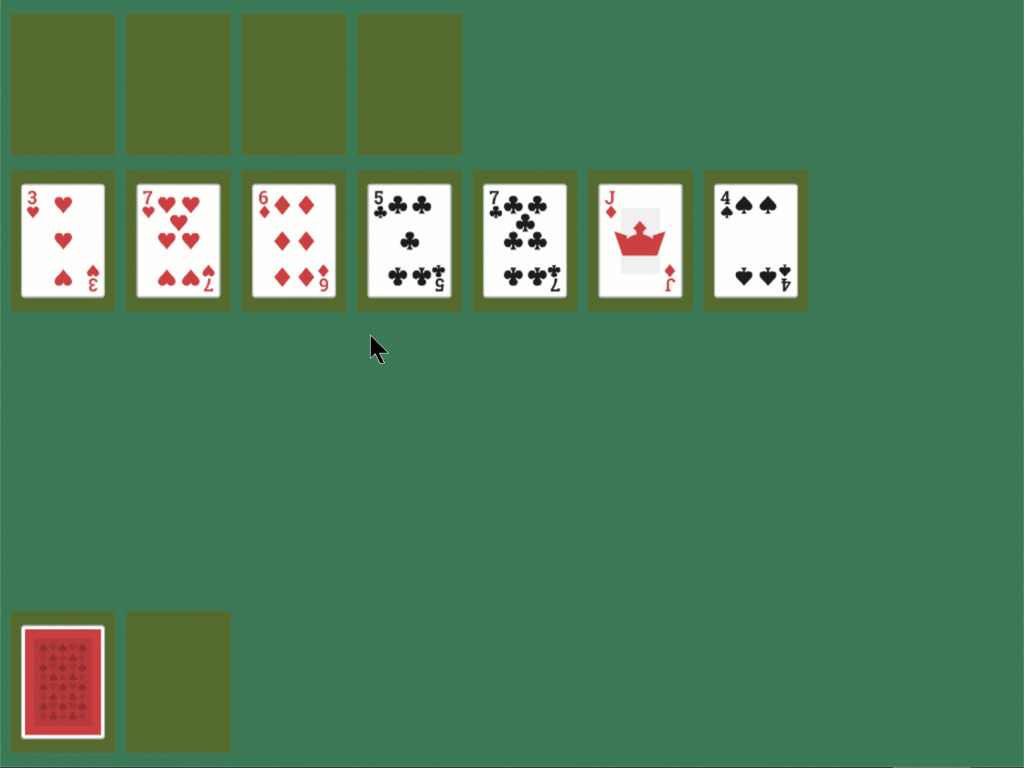
这份纸牌教程将带你了解创建纸牌游戏的基础知识,并做大量的拖放工作。
打开一扇窗#
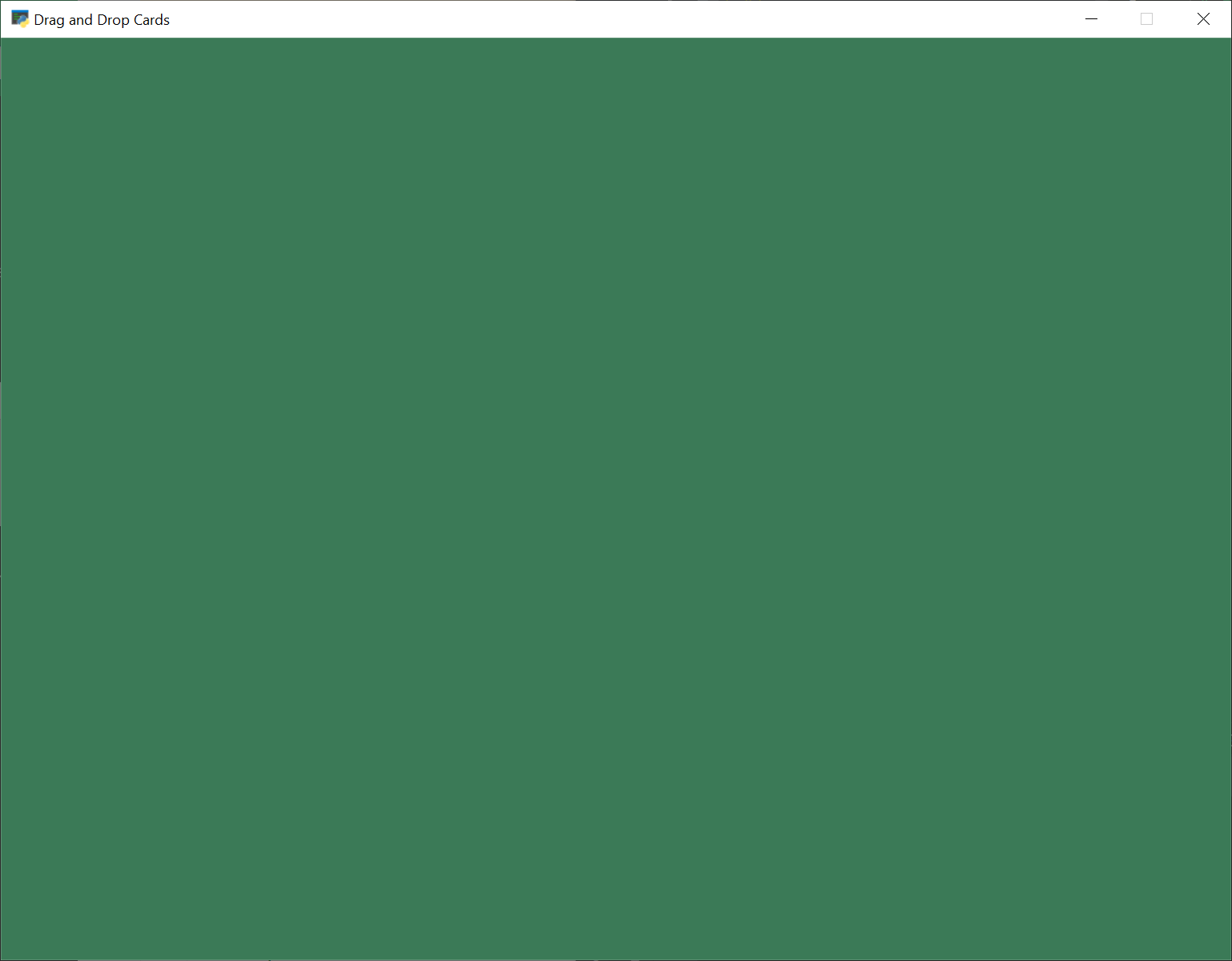
首先,让我们从一个使用Arcade打开空白窗口的程序开始。下面的清单还包含我们稍后将填充的方法的存根。
开始使用这段代码,并确保可以运行它。它应该会弹出一扇绿色的窗户。
1"""
2Solitaire clone.
3"""
4import arcade
5
6# Screen title and size
7SCREEN_WIDTH = 1024
8SCREEN_HEIGHT = 768
9SCREEN_TITLE = "Drag and Drop Cards"
10
11
12class MyGame(arcade.Window):
13 """ Main application class. """
14
15 def __init__(self):
16 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
17
18 self.background_color = arcade.color.AMAZON
19
20 def setup(self):
21 """ Set up the game here. Call this function to restart the game. """
22 pass
23
24 def on_draw(self):
25 """ Render the screen. """
26 # Clear the screen
27 self.clear()
28
29 def on_mouse_press(self, x, y, button, key_modifiers):
30 """ Called when the user presses a mouse button. """
31 pass
32
33 def on_mouse_release(self, x: float, y: float, button: int,
34 modifiers: int):
35 """ Called when the user presses a mouse button. """
36 pass
37
38 def on_mouse_motion(self, x: float, y: float, dx: float, dy: float):
39 """ User moves mouse """
40 pass
41
42
43def main():
44 """ Main function """
45 window = MyGame()
46 window.setup()
47 arcade.run()
48
49
50if __name__ == "__main__":
51 main()
创建卡片精灵#
我们的下一步是创建一组精灵,每张牌一个。
常量#
首先,我们将创建一些常量,用于定位卡片,并跟踪哪张卡片是哪张。
我们可以只硬编码数字,但我喜欢把事情算出来。“垫子”最终将是一个正方形,比每一张卡片略大,用来追踪我们可以把卡片放在哪里。(我们可以在上面放一堆卡片的垫子。)
1# Constants for sizing
2CARD_SCALE = 0.6
3
4# How big are the cards?
5CARD_WIDTH = 140 * CARD_SCALE
6CARD_HEIGHT = 190 * CARD_SCALE
7
8# How big is the mat we'll place the card on?
9MAT_PERCENT_OVERSIZE = 1.25
10MAT_HEIGHT = int(CARD_HEIGHT * MAT_PERCENT_OVERSIZE)
11MAT_WIDTH = int(CARD_WIDTH * MAT_PERCENT_OVERSIZE)
12
13# How much space do we leave as a gap between the mats?
14# Done as a percent of the mat size.
15VERTICAL_MARGIN_PERCENT = 0.10
16HORIZONTAL_MARGIN_PERCENT = 0.10
17
18# The Y of the bottom row (2 piles)
19BOTTOM_Y = MAT_HEIGHT / 2 + MAT_HEIGHT * VERTICAL_MARGIN_PERCENT
20
21# The X of where to start putting things on the left side
22START_X = MAT_WIDTH / 2 + MAT_WIDTH * HORIZONTAL_MARGIN_PERCENT
23
24# Card constants
25CARD_VALUES = ["A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"]
26CARD_SUITS = ["Clubs", "Hearts", "Spades", "Diamonds"]
卡片类别#
接下来,我们将创建一个Card类。Card类是的子类 arcade.Sprite
。它将具有卡片的花色和价值的属性,并基于此自动加载卡片的图像。
我们将使用整个图像作为点击框,所以我们不需要进行耗时的点击框计算。因此,我们将其关闭。否则,装载精灵将需要很长时间。
1class Card(arcade.Sprite):
2 """ Card sprite """
3
4 def __init__(self, suit, value, scale=1):
5 """ Card constructor """
6
7 # Attributes for suit and value
8 self.suit = suit
9 self.value = value
10
11 # Image to use for the sprite when face up
12 self.image_file_name = f":resources:images/cards/card{self.suit}{self.value}.png"
13
14 # Call the parent
15 super().__init__(self.image_file_name, scale, hit_box_algorithm="None")
创建卡片#
我们将首先为 SpriteList
这将是游戏中所有的牌。
1 def __init__(self):
2 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
3
4 # Sprite list with all the cards, no matter what pile they are in.
5 self.card_list = None
6
7 self.background_color = arcade.color.AMAZON
在……里面 setup
我们将创建列表和卡片。我们不在这里做这个 __init__
因为通过将创建分离到它自己的方法中,我们可以通过调用 setup
。
1 def setup(self):
2 """ Set up the game here. Call this function to restart the game. """
3
4 # Sprite list with all the cards, no matter what pile they are in.
5 self.card_list = arcade.SpriteList()
6
7 # Create every card
8 for card_suit in CARD_SUITS:
9 for card_value in CARD_VALUES:
10 card = Card(card_suit, card_value, CARD_SCALE)
11 card.position = START_X, BOTTOM_Y
12 self.card_list.append(card)
绘图卡#
最后,抽出卡片:
1 def on_draw(self):
2 """ Render the screen. """
3 # Clear the screen
4 self.clear()
5
6 # Draw the cards
7 self.card_list.draw()
最后,您应该会看到所有的卡片堆叠在左下角:
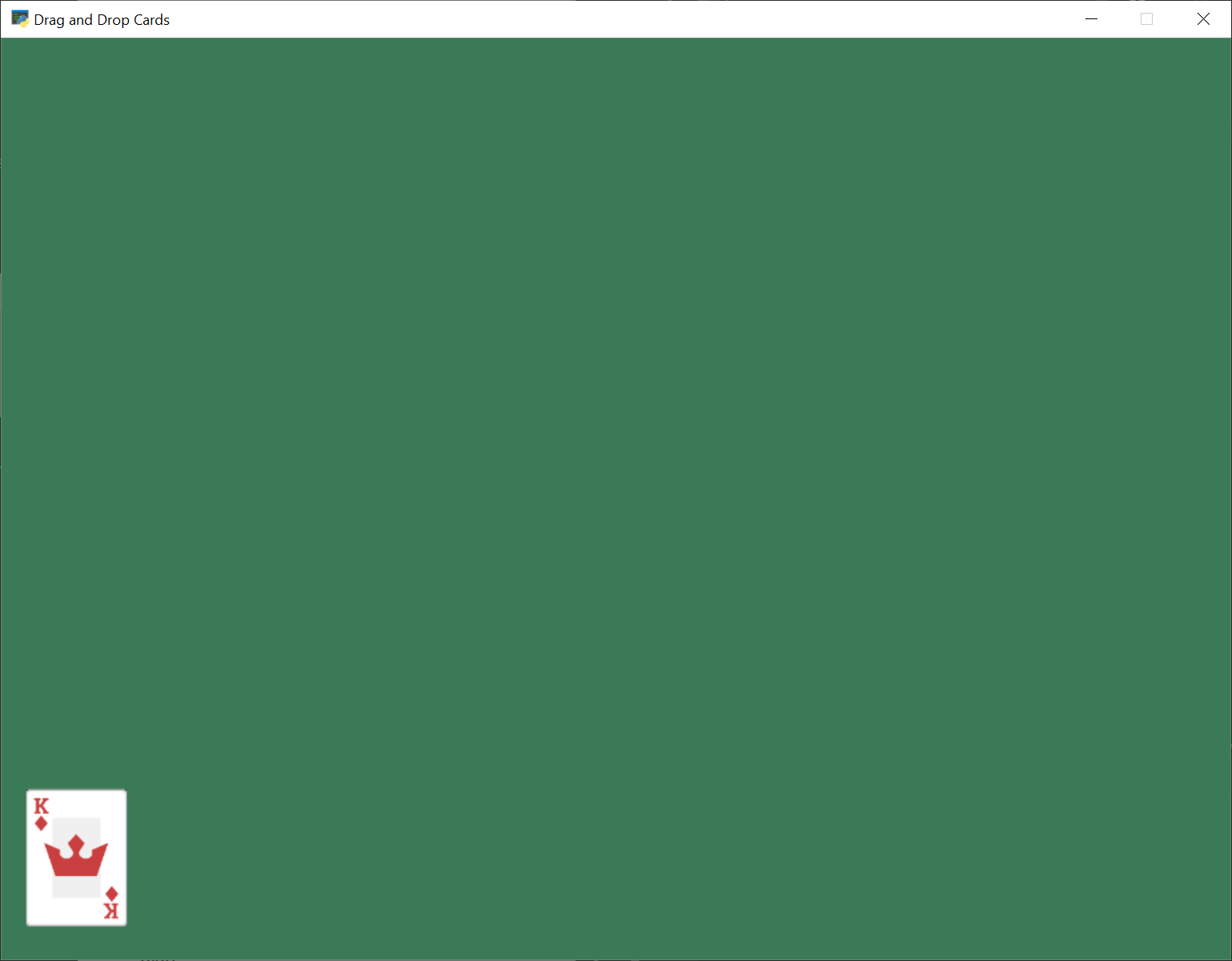
Solitaire_02.py完整列表 ← Full listing of where we are right now
单人纸牌_02.py diff ← What we changed to get here
实现拖放#
接下来,让我们添加拾取、拖放卡片的功能。
跟踪卡片#
首先,让我们添加一些属性来跟踪我们正在移动的牌。因为我们可以移动多张牌,所以我们会将其保留为列表。如果用户将卡放在非法位置,我们将需要将卡重置到其原始位置。所以我们也会追踪这一点。
创建属性:
1 def __init__(self):
2 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
3
4 # Sprite list with all the cards, no matter what pile they are in.
5 self.card_list = None
6
7 self.background_color = arcade.color.AMAZON
8
9 # List of cards we are dragging with the mouse
10 self.held_cards = None
11
12 # Original location of cards we are dragging with the mouse in case
13 # they have to go back.
14 self.held_cards_original_position = None
设置初始值(空列表):
1 def setup(self):
2 """ Set up the game here. Call this function to restart the game. """
3
4 # List of cards we are dragging with the mouse
5 self.held_cards = []
6
7 # Original location of cards we are dragging with the mouse in case
8 # they have to go back.
9 self.held_cards_original_position = []
10
11 # Sprite list with all the cards, no matter what pile they are in.
12 self.card_list = arcade.SpriteList()
13
14 # Create every card
15 for card_suit in CARD_SUITS:
16 for card_value in CARD_VALUES:
17 card = Card(card_suit, card_value, CARD_SCALE)
18 card.position = START_X, BOTTOM_Y
19 self.card_list.append(card)
将卡片拉到抽签顺序的顶部#
当我们点击这张牌时,我们希望它是最后一张抽出的牌,这样它就会出现在所有其他牌的顶部。否则,我们可能会将一张牌拖到另一张牌下面,这看起来会很奇怪。
1 def pull_to_top(self, card: arcade.Sprite):
2 """ Pull card to top of rendering order (last to render, looks on-top) """
3
4 # Remove, and append to the end
5 self.card_list.remove(card)
6 self.card_list.append(card)
鼠标已移动#
如果用户移动鼠标,我们就会用它来移动手中的任何卡片。
1 def on_mouse_motion(self, x: float, y: float, dx: float, dy: float):
2 """ User moves mouse """
3
4 # If we are holding cards, move them with the mouse
5 for card in self.held_cards:
6 card.center_x += dx
7 card.center_y += dy
老鼠被释放#
当用户释放鼠标按钮时,我们将清除持有的卡列表。
1 def on_mouse_release(self, x: float, y: float, button: int,
2 modifiers: int):
3 """ Called when the user presses a mouse button. """
4
5 # If we don't have any cards, who cares
6 if len(self.held_cards) == 0:
7 return
8
9 # We are no longer holding cards
10 self.held_cards = []
测试程序#
现在您应该能够在屏幕上拾起和移动卡片了。试试看!
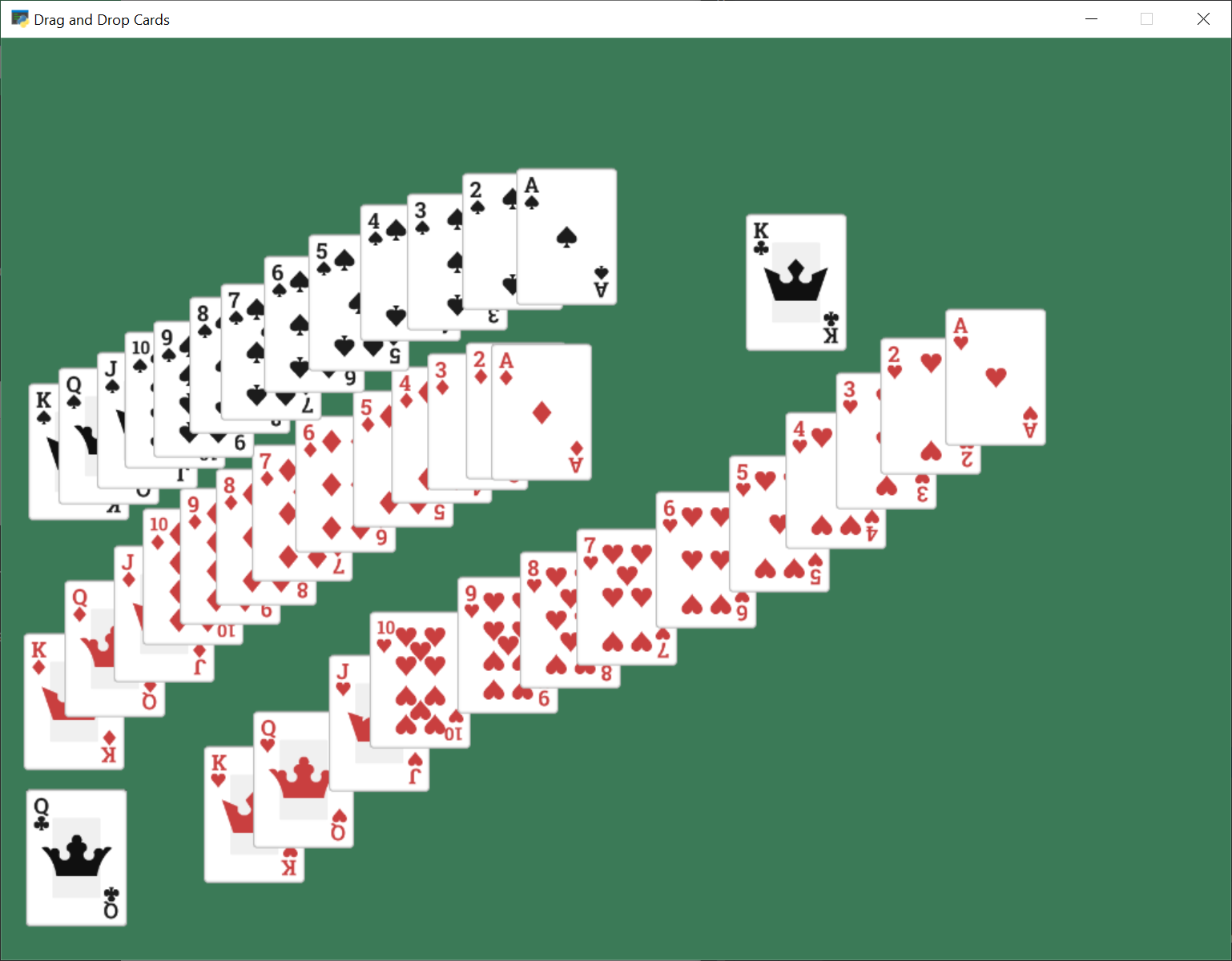
Solitaire_03.py完整列表 ← Full listing of where we are right now
单人纸牌_03.py diff ← What we changed to get here
抽拉桩垫#
接下来,我们将创建精灵,这些精灵将充当游戏中纸牌堆放置位置的向导。我们会将它们创建为精灵,这样我们就可以使用碰撞检测来确定我们是否在它们上面掉了一张牌。
创建常量#
首先,我们将为中间行的七个堆创建常量,为顶行的四个堆创建常量。我们还将创建一个常数,以确定每一堆应该有多远。
同样,我们可以硬编码数字,但我喜欢计算它们,这样我就可以很容易地更改比例。
1# The Y of the top row (4 piles)
2TOP_Y = SCREEN_HEIGHT - MAT_HEIGHT / 2 - MAT_HEIGHT * VERTICAL_MARGIN_PERCENT
3
4# The Y of the middle row (7 piles)
5MIDDLE_Y = TOP_Y - MAT_HEIGHT - MAT_HEIGHT * VERTICAL_MARGIN_PERCENT
6
7# How far apart each pile goes
8X_SPACING = MAT_WIDTH + MAT_WIDTH * HORIZONTAL_MARGIN_PERCENT
创建垫Sprite#
为垫子精灵列表创建一个属性:
1 def __init__(self):
2 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
3
4 # Sprite list with all the cards, no matter what pile they are in.
5 self.card_list = None
6
7 self.background_color = arcade.color.AMAZON
8
9 # List of cards we are dragging with the mouse
10 self.held_cards = None
11
12 # Original location of cards we are dragging with the mouse in case
13 # they have to go back.
14 self.held_cards_original_position = None
15
16 # Sprite list with all the mats tha cards lay on.
17 self.pile_mat_list = None
然后在中创建垫精灵 setup
方法
1 def setup(self):
2 """ Set up the game here. Call this function to restart the game. """
3
4 # List of cards we are dragging with the mouse
5 self.held_cards = []
6
7 # Original location of cards we are dragging with the mouse in case
8 # they have to go back.
9 self.held_cards_original_position = []
10
11 # --- Create the mats the cards go on.
12
13 # Sprite list with all the mats tha cards lay on.
14 self.pile_mat_list: arcade.SpriteList = arcade.SpriteList()
15
16 # Create the mats for the bottom face down and face up piles
17 pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
18 pile.position = START_X, BOTTOM_Y
19 self.pile_mat_list.append(pile)
20
21 pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
22 pile.position = START_X + X_SPACING, BOTTOM_Y
23 self.pile_mat_list.append(pile)
24
25 # Create the seven middle piles
26 for i in range(7):
27 pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
28 pile.position = START_X + i * X_SPACING, MIDDLE_Y
29 self.pile_mat_list.append(pile)
30
31 # Create the top "play" piles
32 for i in range(4):
33 pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
34 pile.position = START_X + i * X_SPACING, TOP_Y
35 self.pile_mat_list.append(pile)
36
37 # Sprite list with all the cards, no matter what pile they are in.
38 self.card_list = arcade.SpriteList()
39
40 # Create every card
41 for card_suit in CARD_SUITS:
42 for card_value in CARD_VALUES:
43 card = Card(card_suit, card_value, CARD_SCALE)
44 card.position = START_X, BOTTOM_Y
45 self.card_list.append(card)
画垫Sprite#
最后,如果我们不画这些垫子,它们就不会显示:
1 def on_draw(self):
2 """ Render the screen. """
3 # Clear the screen
4 self.clear()
5
6 # Draw the mats the cards go on to
7 self.pile_mat_list.draw()
8
9 # Draw the cards
10 self.card_list.draw()
测试程序#
运行该程序,查看垫子是否出现:
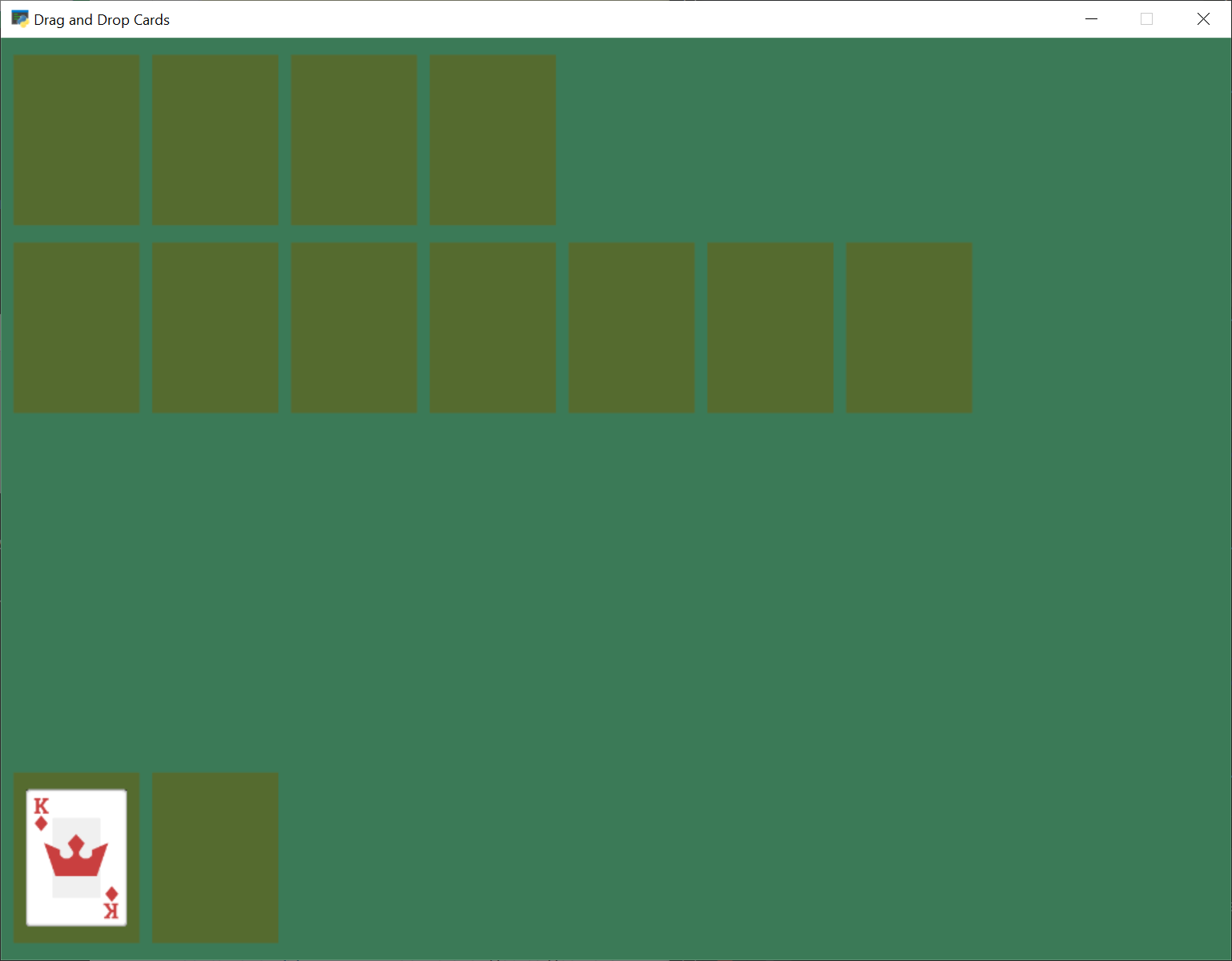
Solitaire_04.py完整列表 ← Full listing of where we are right now
单人纸牌_04.py diff ← What we changed to get here
将卡片卡到桩上#
现在,你可以把卡片拖到任何地方。它们不一定要堆成一堆。让我们添加将卡“咬合”到一堆上的代码。如果我们不落在一堆上,我们就重置到原来的位置。
1 def on_mouse_release(self, x: float, y: float, button: int,
2 modifiers: int):
3 """ Called when the user presses a mouse button. """
4
5 # If we don't have any cards, who cares
6 if len(self.held_cards) == 0:
7 return
8
9 # Find the closest pile, in case we are in contact with more than one
10 pile, distance = arcade.get_closest_sprite(self.held_cards[0], self.pile_mat_list)
11 reset_position = True
12
13 # See if we are in contact with the closest pile
14 if arcade.check_for_collision(self.held_cards[0], pile):
15
16 # For each held card, move it to the pile we dropped on
17 for i, dropped_card in enumerate(self.held_cards):
18 # Move cards to proper position
19 dropped_card.position = pile.center_x, pile.center_y
20
21 # Success, don't reset position of cards
22 reset_position = False
23
24 # Release on top play pile? And only one card held?
25 if reset_position:
26 # Where-ever we were dropped, it wasn't valid. Reset the each card's position
27 # to its original spot.
28 for pile_index, card in enumerate(self.held_cards):
29 card.position = self.held_cards_original_position[pile_index]
30
31 # We are no longer holding cards
32 self.held_cards = []
Solitaire_05.py完整列表 ← Full listing of where we are right now
单人纸牌_05.py diff ← What we changed to get here
洗牌#
把所有的牌都整理好是很无聊的。让我们把他们拖入 setup
方法:
1 # Shuffle the cards
2 for pos1 in range(len(self.card_list)):
3 pos2 = random.randrange(len(self.card_list))
4 self.card_list.swap(pos1, pos2)
别忘了 import random
在顶端。
运行你的程序,并确保你可以移动卡片。
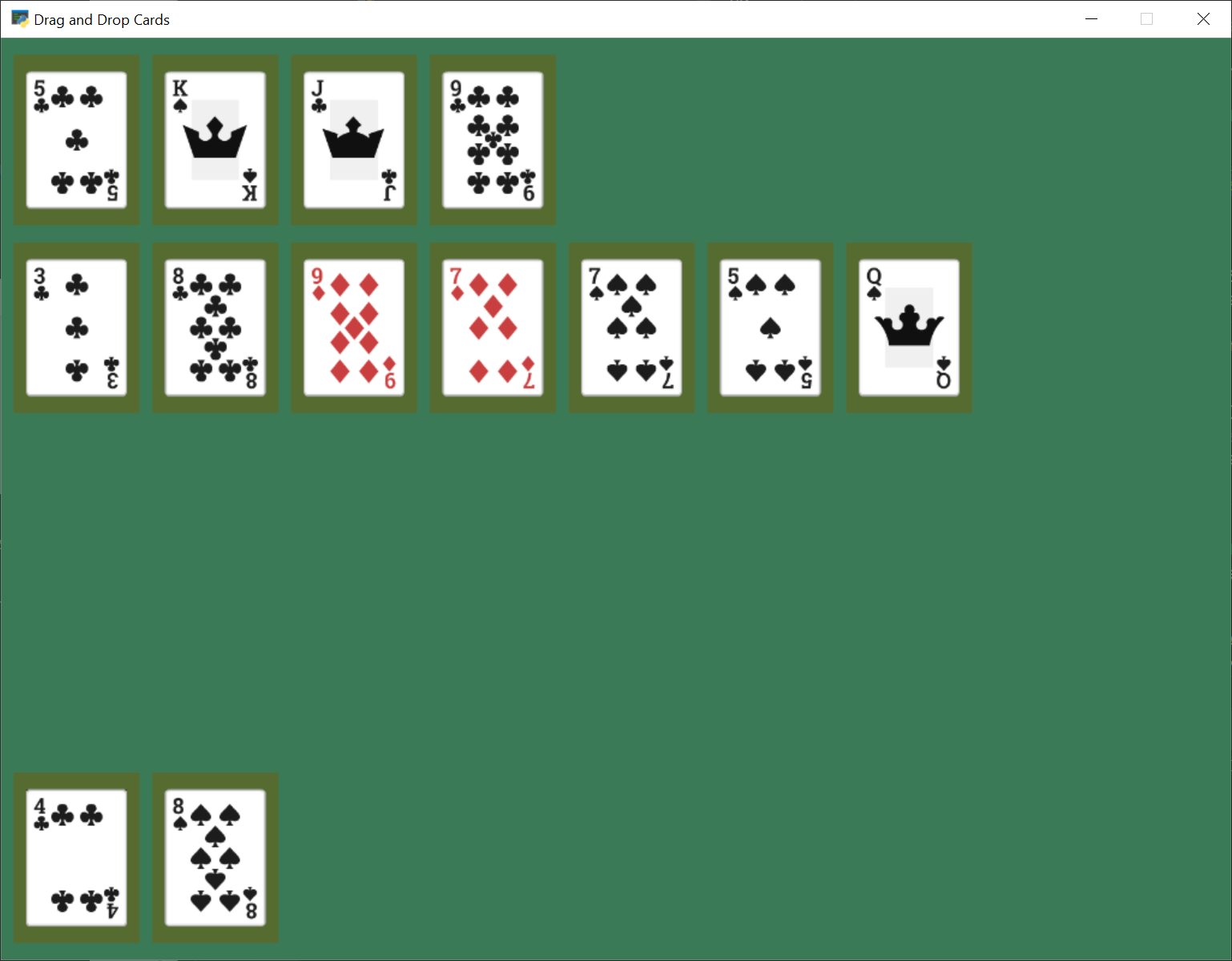
Solitaire_06.py完整列表 ← Full listing of where we are right now
单人纸牌_06.py diff ← What we changed to get here
轨道卡桩#
现在我们正在把牌转过来。但要弄清楚哪一堆卡里是哪张卡并不容易。我们可以按位置检查,但之后我们就开始扇出卡片,这将是非常困难的。
因此,我们将为每一堆卡片保留一个单独的列表。当我们移动一张卡时,我们需要移动位置,并切换它在哪个列表中。
添加新常量#
首先,让我们为每个堆添加一些常量:
1# If we fan out cards stacked on each other, how far apart to fan them?
2CARD_VERTICAL_OFFSET = CARD_HEIGHT * CARD_SCALE * 0.3
3
4# Constants that represent "what pile is what" for the game
5PILE_COUNT = 13
6BOTTOM_FACE_DOWN_PILE = 0
7BOTTOM_FACE_UP_PILE = 1
8PLAY_PILE_1 = 2
9PLAY_PILE_2 = 3
10PLAY_PILE_3 = 4
11PLAY_PILE_4 = 5
12PLAY_PILE_5 = 6
13PLAY_PILE_6 = 7
14PLAY_PILE_7 = 8
15TOP_PILE_1 = 9
16TOP_PILE_2 = 10
17TOP_PILE_3 = 11
18TOP_PILE_4 = 12
创建堆叠列表#
然后在我们的 __init__
添加一个变量以跟踪桩:
1 # Create a list of lists, each holds a pile of cards.
2 self.piles = None
在 setup
方法,则为每个堆创建一个列表。然后,将所有卡片添加到正面朝下的交易堆中。(稍后,我们将添加对面朝下卡的支持。是的,现在所有正面朝下的牌都是向上的。)
1 # Create a list of lists, each holds a pile of cards.
2 self.piles = [[] for _ in range(PILE_COUNT)]
3
4 # Put all the cards in the bottom face-down pile
5 for card in self.card_list:
6 self.piles[BOTTOM_FACE_DOWN_PILE].append(card)
卡片堆放管理办法#
接下来,我们需要一些我们将在其他地方使用的方便方法。
首先,给定一张卡片,返回该卡片属于哪一堆的索引:
1 def get_pile_for_card(self, card):
2 """ What pile is this card in? """
3 for index, pile in enumerate(self.piles):
4 if card in pile:
5 return index
接下来,从一堆卡片中取出一张卡片。
1 def remove_card_from_pile(self, card):
2 """ Remove card from whatever pile it was in. """
3 for pile in self.piles:
4 if card in pile:
5 pile.remove(card)
6 break
最后,将卡片从一堆卡片移到另一堆卡片。
1 def move_card_to_new_pile(self, card, pile_index):
2 """ Move the card to a new pile """
3 self.remove_card_from_pile(card)
4 self.piles[pile_index].append(card)
丢掉卡片#
接下来,我们需要修改松开鼠标时发生的事情。
首先,看看我们能不能把它放到原来的那一堆。如果是,只需将卡重置回其原始位置即可。
1 def on_mouse_release(self, x: float, y: float, button: int,
2 modifiers: int):
3 """ Called when the user presses a mouse button. """
4
5 # If we don't have any cards, who cares
6 if len(self.held_cards) == 0:
7 return
8
9 # Find the closest pile, in case we are in contact with more than one
10 pile, distance = arcade.get_closest_sprite(self.held_cards[0], self.pile_mat_list)
11 reset_position = True
12
13 # See if we are in contact with the closest pile
14 if arcade.check_for_collision(self.held_cards[0], pile):
15
16 # What pile is it?
17 pile_index = self.pile_mat_list.index(pile)
18
19 # Is it the same pile we came from?
20 if pile_index == self.get_pile_for_card(self.held_cards[0]):
21 # If so, who cares. We'll just reset our position.
22 pass
如果它在一个中间的游戏堆上呢?呃,这有点复杂。如果垫子是空的,我们需要把它放在垫子的中间。如果垫子上有卡片,我们需要对卡片进行偏移,这样我们才能看到卡片的分布。
虽然我们现在一次只能拿起一张卡,但一旦我们支持多张卡携带,我们就需要支持丢弃多张卡。
1 # Is it on a middle play pile?
2 elif PLAY_PILE_1 <= pile_index <= PLAY_PILE_7:
3 # Are there already cards there?
4 if len(self.piles[pile_index]) > 0:
5 # Move cards to proper position
6 top_card = self.piles[pile_index][-1]
7 for i, dropped_card in enumerate(self.held_cards):
8 dropped_card.position = top_card.center_x, \
9 top_card.center_y - CARD_VERTICAL_OFFSET * (i + 1)
10 else:
11 # Are there no cards in the middle play pile?
12 for i, dropped_card in enumerate(self.held_cards):
13 # Move cards to proper position
14 dropped_card.position = pile.center_x, \
15 pile.center_y - CARD_VERTICAL_OFFSET * i
16
17 for card in self.held_cards:
18 # Cards are in the right position, but we need to move them to the right list
19 self.move_card_to_new_pile(card, pile_index)
20
21 # Success, don't reset position of cards
22 reset_position = False
如果它是在顶级播放堆中发布的,会怎么样?确保我们手中只有一张卡。我们不想把一堆东西放在上面。然后把卡片移到那堆卡片上。
1 # Release on top play pile? And only one card held?
2 elif TOP_PILE_1 <= pile_index <= TOP_PILE_4 and len(self.held_cards) == 1:
3 # Move position of card to pile
4 self.held_cards[0].position = pile.position
5 # Move card to card list
6 for card in self.held_cards:
7 self.move_card_to_new_pile(card, pile_index)
8
9 reset_position = False
如果移动无效,我们需要将所有持有的卡重新设置到初始位置。
1 if reset_position:
2 # Where-ever we were dropped, it wasn't valid. Reset the each card's position
3 # to its original spot.
4 for pile_index, card in enumerate(self.held_cards):
5 card.position = self.held_cards_original_position[pile_index]
6
7 # We are no longer holding cards
8 self.held_cards = []
测试#
测试你的程序,看看卡片是否被正确地展开。
备注
该代码并没有强制执行任何游戏规则。你可以按任何顺序堆叠纸牌。此外,对于长长的卡片堆叠,你仍然必须将卡片掉在垫子上。当一叠卡片向下延伸超过垫子时,这是违反直觉的。
我们将这些问题的解决方案留给读者作为练习。

Solitaire_07.py完整列表 ← Full listing of where we are right now
单人纸牌_07.py diff ← What we changed to get here
拾取卡片堆叠#
我们怎么才能拿到一叠卡片呢?当鼠标被按下时,我们需要找出卡在哪一堆中。
接下来,看看我们点击的卡片在堆中的什么位置。如果稍后有任何卡片放在这堆卡片上,我们也想拿起那些卡片。将它们添加到列表中。
1 def on_mouse_press(self, x, y, button, key_modifiers):
2 """ Called when the user presses a mouse button. """
3
4 # Get list of cards we've clicked on
5 cards = arcade.get_sprites_at_point((x, y), self.card_list)
6
7 # Have we clicked on a card?
8 if len(cards) > 0:
9
10 # Might be a stack of cards, get the top one
11 primary_card = cards[-1]
12 # Figure out what pile the card is in
13 pile_index = self.get_pile_for_card(primary_card)
14
15 # All other cases, grab the face-up card we are clicking on
16 self.held_cards = [primary_card]
17 # Save the position
18 self.held_cards_original_position = [self.held_cards[0].position]
19 # Put on top in drawing order
20 self.pull_to_top(self.held_cards[0])
21
22 # Is this a stack of cards? If so, grab the other cards too
23 card_index = self.piles[pile_index].index(primary_card)
24 for i in range(card_index + 1, len(self.piles[pile_index])):
25 card = self.piles[pile_index][i]
26 self.held_cards.append(card)
27 self.held_cards_original_position.append(card.position)
28 self.pull_to_top(card)
在此之后,您应该能够用鼠标从中间一堆卡片中拿起一叠卡片,并将它们四处移动。
Solitaire_08.py完整列表 ← Full listing of where we are right now
单人纸牌_08.py diff ← What we changed to get here
发牌卡#
我们可以将卡片分成七个中间的堆,方法是向 setup
方法。我们需要更改每张卡所属的列表以及它的位置。
1 # - Pull from that pile into the middle piles, all face-down
2 # Loop for each pile
3 for pile_no in range(PLAY_PILE_1, PLAY_PILE_7 + 1):
4 # Deal proper number of cards for that pile
5 for j in range(pile_no - PLAY_PILE_1 + 1):
6 # Pop the card off the deck we are dealing from
7 card = self.piles[BOTTOM_FACE_DOWN_PILE].pop()
8 # Put in the proper pile
9 self.piles[pile_no].append(card)
10 # Move card to same position as pile we just put it in
11 card.position = self.pile_mat_list[pile_no].position
12 # Put on top in draw order
13 self.pull_to_top(card)
Solitaire_09.py完整列表 ← Full listing of where we are right now
单人纸牌_09.py diff ← What we changed to get here
正面朝下的卡片#
我们不会在所有纸牌都朝上的情况下玩单人纸牌,所以让我们在游戏中增加正面朝下的支持。
新常量#
首先为正面朝下时使用的图像定义一个常量。
1# Face down image
2FACE_DOWN_IMAGE = ":resources:images/cards/cardBack_red2.png"
对Card类的更新#
下一步,默认 Card
全班都要正面朝上。另外,让我们添加一些方法来向上或向下翻转牌。
1class Card(arcade.Sprite):
2 """ Card sprite """
3
4 def __init__(self, suit, value, scale=1):
5 """ Card constructor """
6
7 # Attributes for suit and value
8 self.suit = suit
9 self.value = value
10
11 # Image to use for the sprite when face up
12 self.image_file_name = f":resources:images/cards/card{self.suit}{self.value}.png"
13 self.is_face_up = False
14 super().__init__(FACE_DOWN_IMAGE, scale, hit_box_algorithm="None")
15
16 def face_down(self):
17 """ Turn card face-down """
18 self.texture = arcade.load_texture(FACE_DOWN_IMAGE)
19 self.is_face_up = False
20
21 def face_up(self):
22 """ Turn card face-up """
23 self.texture = arcade.load_texture(self.image_file_name)
24 self.is_face_up = True
25
26 @property
27 def is_face_down(self):
28 """ Is this card face down? """
29 return not self.is_face_up
在中七堆上翻起纸牌#
现在每张牌都是正面朝下的。让我们更新一下 setup
方法使中间七堆最上面的卡片正面朝上。
1 # Flip up the top cards
2 for i in range(PLAY_PILE_1, PLAY_PILE_7 + 1):
3 self.piles[i][-1].face_up()
点击时弹出卡片#
当我们点击一张正面朝下的卡片时,让我们把它翻过来,而不是拿起它:
1 def on_mouse_press(self, x, y, button, key_modifiers):
2 """ Called when the user presses a mouse button. """
3
4 # Get list of cards we've clicked on
5 cards = arcade.get_sprites_at_point((x, y), self.card_list)
6
7 # Have we clicked on a card?
8 if len(cards) > 0:
9
10 # Might be a stack of cards, get the top one
11 primary_card = cards[-1]
12 assert isinstance(primary_card, Card)
13
14 # Figure out what pile the card is in
15 pile_index = self.get_pile_for_card(primary_card)
16
17 if primary_card.is_face_down:
18 # Is the card face down? In one of those middle 7 piles? Then flip up
19 primary_card.face_up()
20 else:
21 # All other cases, grab the face-up card we are clicking on
22 self.held_cards = [primary_card]
23 # Save the position
24 self.held_cards_original_position = [self.held_cards[0].position]
25 # Put on top in drawing order
26 self.pull_to_top(self.held_cards[0])
27
28 # Is this a stack of cards? If so, grab the other cards too
29 card_index = self.piles[pile_index].index(primary_card)
30 for i in range(card_index + 1, len(self.piles[pile_index])):
31 card = self.piles[pile_index][i]
32 self.held_cards.append(card)
33 self.held_cards_original_position.append(card.position)
34 self.pull_to_top(card)
测试#
试试看你的程序。当你移动纸牌时,你应该也能看到正面朝下的纸牌,并且能够将它们翻过来。
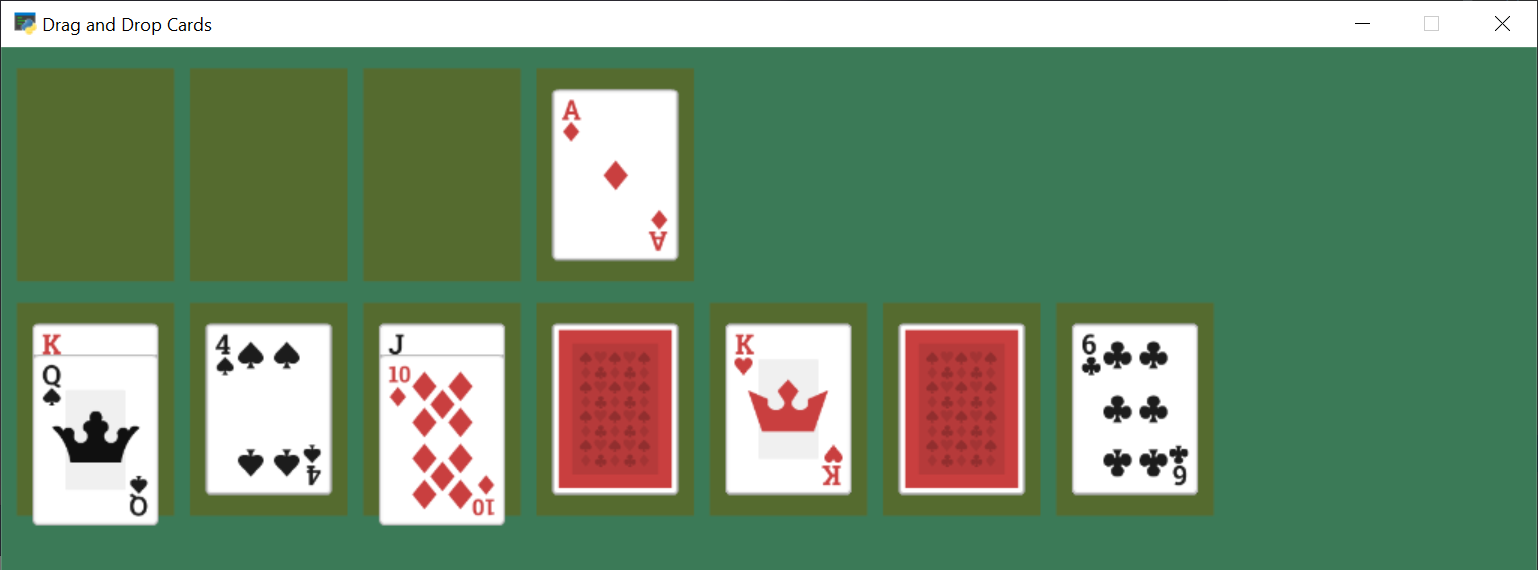
Solitaire_10.py完整列表 ← Full listing of where we are right now
Solitaire_10.py diff ← What we changed to get here
重启游戏#
我们可以添加重新启动游戏的能力,任何类型的游戏我们都可以按下‘R’键:
1 def on_key_press(self, symbol: int, modifiers: int):
2 """ User presses key """
3 if symbol == arcade.key.R:
4 # Restart
5 self.setup()
从抽签堆翻三个字#
我们屏幕底部的抽签堆还不能正常工作。当我们点击它时,我们需要它将三张牌翻到右下角。此外,如果已经检查了堆中的所有卡片,我们需要重置堆,以便可以再次检查。
1 def on_mouse_press(self, x, y, button, key_modifiers):
2 """ Called when the user presses a mouse button. """
3
4 # Get list of cards we've clicked on
5 cards = arcade.get_sprites_at_point((x, y), self.card_list)
6
7 # Have we clicked on a card?
8 if len(cards) > 0:
9
10 # Might be a stack of cards, get the top one
11 primary_card = cards[-1]
12 assert isinstance(primary_card, Card)
13
14 # Figure out what pile the card is in
15 pile_index = self.get_pile_for_card(primary_card)
16
17 # Are we clicking on the bottom deck, to flip three cards?
18 if pile_index == BOTTOM_FACE_DOWN_PILE:
19 # Flip three cards
20 for i in range(3):
21 # If we ran out of cards, stop
22 if len(self.piles[BOTTOM_FACE_DOWN_PILE]) == 0:
23 break
24 # Get top card
25 card = self.piles[BOTTOM_FACE_DOWN_PILE][-1]
26 # Flip face up
27 card.face_up()
28 # Move card position to bottom-right face up pile
29 card.position = self.pile_mat_list[BOTTOM_FACE_UP_PILE].position
30 # Remove card from face down pile
31 self.piles[BOTTOM_FACE_DOWN_PILE].remove(card)
32 # Move card to face up list
33 self.piles[BOTTOM_FACE_UP_PILE].append(card)
34 # Put on top draw-order wise
35 self.pull_to_top(card)
36
37 elif primary_card.is_face_down:
38 # Is the card face down? In one of those middle 7 piles? Then flip up
39 primary_card.face_up()
40 else:
41 # All other cases, grab the face-up card we are clicking on
42 self.held_cards = [primary_card]
43 # Save the position
44 self.held_cards_original_position = [self.held_cards[0].position]
45 # Put on top in drawing order
46 self.pull_to_top(self.held_cards[0])
47
48 # Is this a stack of cards? If so, grab the other cards too
49 card_index = self.piles[pile_index].index(primary_card)
50 for i in range(card_index + 1, len(self.piles[pile_index])):
51 card = self.piles[pile_index][i]
52 self.held_cards.append(card)
53 self.held_cards_original_position.append(card.position)
54 self.pull_to_top(card)
55
56 else:
57
58 # Click on a mat instead of a card?
59 mats = arcade.get_sprites_at_point((x, y), self.pile_mat_list)
60
61 if len(mats) > 0:
62 mat = mats[0]
63 mat_index = self.pile_mat_list.index(mat)
64
65 # Is it our turned over flip mat? and no cards on it?
66 if mat_index == BOTTOM_FACE_DOWN_PILE and len(self.piles[BOTTOM_FACE_DOWN_PILE]) == 0:
67 # Flip the deck back over so we can restart
68 temp_list = self.piles[BOTTOM_FACE_UP_PILE].copy()
69 for card in reversed(temp_list):
70 card.face_down()
71 self.piles[BOTTOM_FACE_UP_PILE].remove(card)
72 self.piles[BOTTOM_FACE_DOWN_PILE].append(card)
73 card.position = self.pile_mat_list[BOTTOM_FACE_DOWN_PILE].position
测试#
现在我们有了一个基本的工作单人纸牌游戏!试试看!
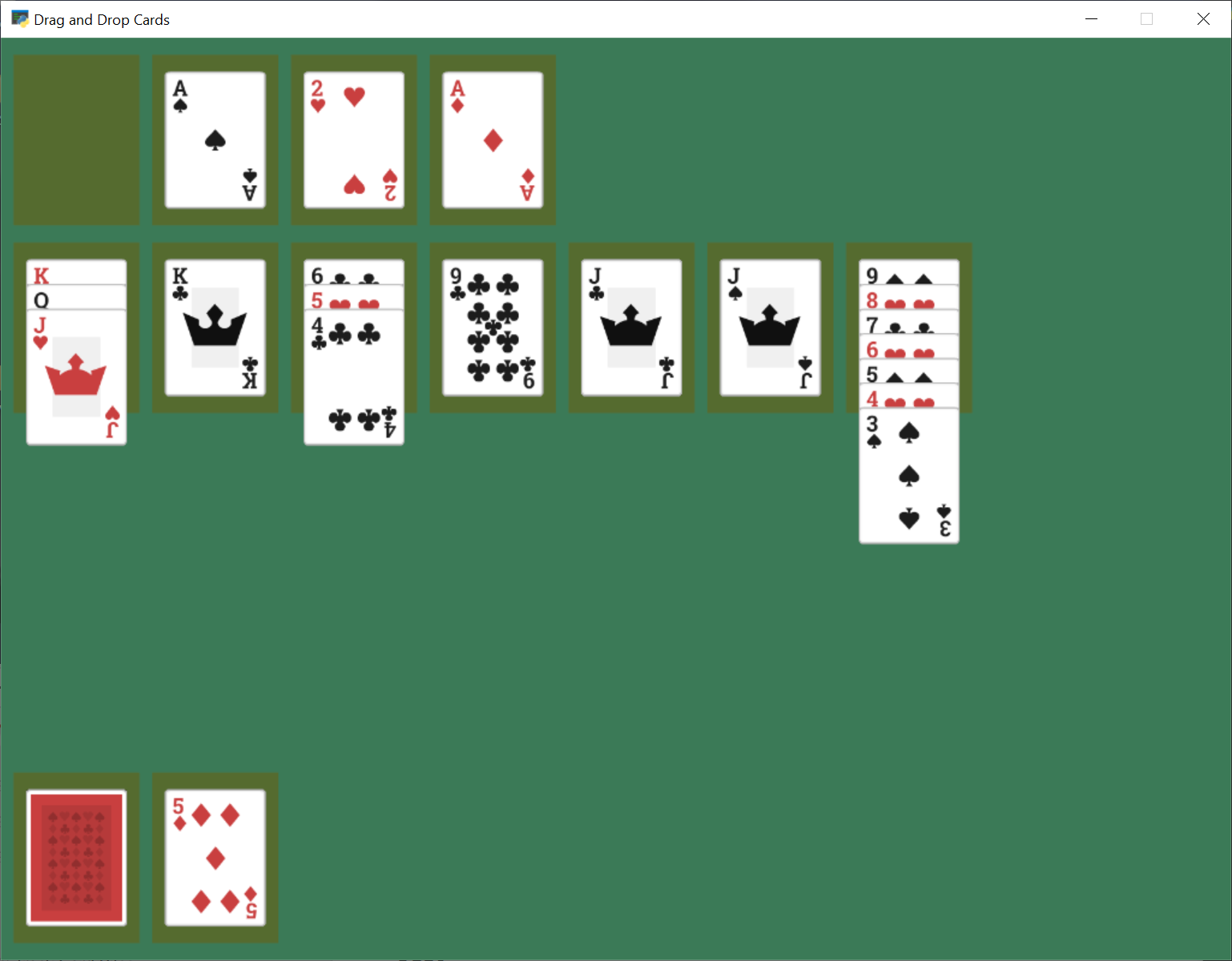
Solitaire_11.py完整列表 ← Full listing of where we are right now
单人纸牌_11.py diff ← What we changed to get here
结论#
这个游戏还有更多的东西可以添加,比如执行规则,添加动画来将掉落的卡片滑动到它的位置,声音,更好的图形,等等。或者,这可以改编成一种不同的纸牌游戏。
希望这足以让你开始自己的游戏。