第6步: Python#
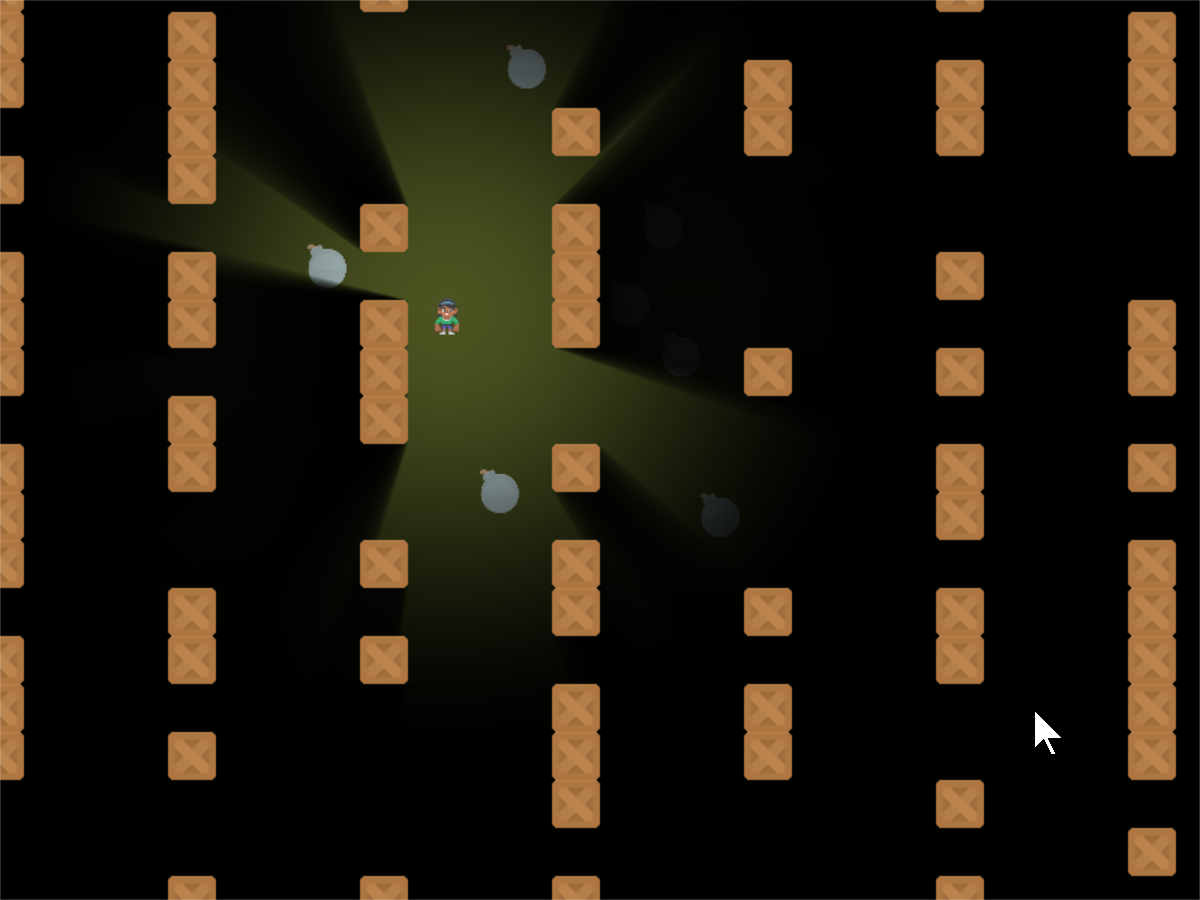
step_06.py#
1import random
2
3import arcade
4from arcade.experimental import Shadertoy
5
6# Do the math to figure out our screen dimensions
7SCREEN_WIDTH = 800
8SCREEN_HEIGHT = 600
9SCREEN_TITLE = "Ray-casting Demo"
10
11SPRITE_SCALING = 0.25
12
13# How fast the camera pans to the player. 1.0 is instant.
14CAMERA_SPEED = 0.1
15
16PLAYER_MOVEMENT_SPEED = 7
17BOMB_COUNT = 70
18PLAYING_FIELD_WIDTH = 1600
19PLAYING_FIELD_HEIGHT = 1600
20
21
22class MyGame(arcade.Window):
23
24 def __init__(self, width, height, title):
25 super().__init__(width, height, title)
26
27 # The shader toy and 'channels' we'll be using
28 self.shadertoy = None
29 self.channel0 = None
30 self.channel1 = None
31 self.load_shader()
32
33 # Sprites and sprite lists
34 self.player_sprite = None
35 self.wall_list = arcade.SpriteList()
36 self.player_list = arcade.SpriteList()
37 self.bomb_list = arcade.SpriteList()
38 self.physics_engine = None
39
40 self.generate_sprites()
41 self.background_color = arcade.color.ARMY_GREEN
42
43 def load_shader(self):
44 # Size of the window
45 window_size = self.get_size()
46
47 # Create the shader toy, passing in a path for the shader source
48 self.shadertoy = Shadertoy.create_from_file(window_size, "step_06.glsl")
49
50 # Create the channels 0 and 1 frame buffers.
51 # Make the buffer the size of the window, with 4 channels (RGBA)
52 self.channel0 = self.shadertoy.ctx.framebuffer(
53 color_attachments=[self.shadertoy.ctx.texture(window_size, components=4)]
54 )
55 self.channel1 = self.shadertoy.ctx.framebuffer(
56 color_attachments=[self.shadertoy.ctx.texture(window_size, components=4)]
57 )
58
59 # Assign the frame buffers to the channels
60 self.shadertoy.channel_0 = self.channel0.color_attachments[0]
61 self.shadertoy.channel_1 = self.channel1.color_attachments[0]
62
63 def generate_sprites(self):
64 # -- Set up several columns of walls
65 for x in range(0, PLAYING_FIELD_WIDTH, 128):
66 for y in range(0, PLAYING_FIELD_HEIGHT, int(128 * SPRITE_SCALING)):
67 # Randomly skip a box so the player can find a way through
68 if random.randrange(2) > 0:
69 wall = arcade.Sprite(":resources:images/tiles/boxCrate_double.png", SPRITE_SCALING)
70 wall.center_x = x
71 wall.center_y = y
72 self.wall_list.append(wall)
73
74 # -- Set some hidden bombs in the area
75 for i in range(BOMB_COUNT):
76 bomb = arcade.Sprite(":resources:images/tiles/bomb.png", 0.25)
77 placed = False
78 while not placed:
79 bomb.center_x = random.randrange(PLAYING_FIELD_WIDTH)
80 bomb.center_y = random.randrange(PLAYING_FIELD_HEIGHT)
81 if not arcade.check_for_collision_with_list(bomb, self.wall_list):
82 placed = True
83 self.bomb_list.append(bomb)
84
85 # Create the player
86 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
87 scale=SPRITE_SCALING)
88 self.player_sprite.center_x = 256
89 self.player_sprite.center_y = 512
90 self.player_list.append(self.player_sprite)
91
92 # Physics engine, so we don't run into walls
93 self.physics_engine = arcade.PhysicsEngineSimple(self.player_sprite, self.wall_list)
94
95 def on_draw(self):
96 # Select the channel 0 frame buffer to draw on
97 self.channel0.use()
98 self.channel0.clear()
99 # Draw the walls
100 self.wall_list.draw()
101
102 self.channel1.use()
103 self.channel1.clear()
104 # Draw the bombs
105 self.bomb_list.draw()
106
107 # Select this window to draw on
108 self.use()
109 # Clear to background color
110 self.clear()
111 # Run the shader and render to the window
112 self.shadertoy.program['lightPosition'] = self.player_sprite.position
113 self.shadertoy.program['lightSize'] = 300
114 self.shadertoy.render()
115
116 # Draw the walls
117 self.wall_list.draw()
118
119 # Draw the player
120 self.player_list.draw()
121
122 def on_key_press(self, key, modifiers):
123 """Called whenever a key is pressed. """
124
125 if key == arcade.key.UP:
126 self.player_sprite.change_y = PLAYER_MOVEMENT_SPEED
127 elif key == arcade.key.DOWN:
128 self.player_sprite.change_y = -PLAYER_MOVEMENT_SPEED
129 elif key == arcade.key.LEFT:
130 self.player_sprite.change_x = -PLAYER_MOVEMENT_SPEED
131 elif key == arcade.key.RIGHT:
132 self.player_sprite.change_x = PLAYER_MOVEMENT_SPEED
133
134 def on_key_release(self, key, modifiers):
135 """Called when the user releases a key. """
136
137 if key == arcade.key.UP or key == arcade.key.DOWN:
138 self.player_sprite.change_y = 0
139 elif key == arcade.key.LEFT or key == arcade.key.RIGHT:
140 self.player_sprite.change_x = 0
141
142 def on_update(self, delta_time):
143 """ Movement and game logic """
144
145 # Call update on all sprites (The sprites don't do much in this
146 # example though.)
147 self.physics_engine.update()
148
149
150if __name__ == "__main__":
151 MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
152 arcade.run()