精灵属性#
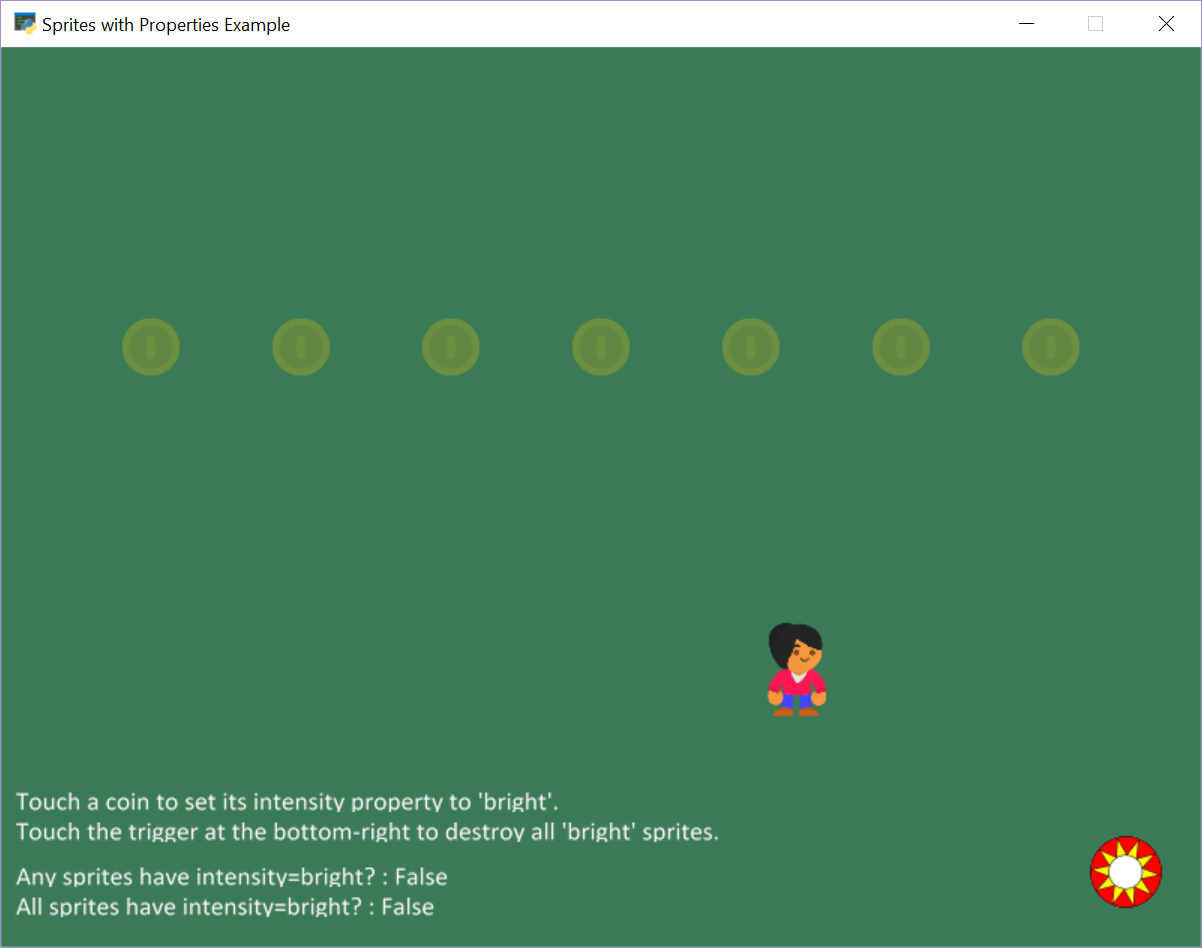
sprite_properties.py#
1"""
2Sprites with Properties Example
3
4Simple program to show how to store properties on sprites.
5
6Artwork from https://kenney.nl
7
8If Python and Arcade are installed, this example can be run from the command line with:
9python -m arcade.examples.sprite_properties
10"""
11import arcade
12
13# --- Constants ---
14SPRITE_SCALING_PLAYER = 0.5
15
16SCREEN_WIDTH = 800
17SCREEN_HEIGHT = 600
18SCREEN_TITLE = "Sprites with Properties Example"
19
20
21class MyGame(arcade.Window):
22 """ Our custom Window Class"""
23
24 def __init__(self):
25 """ Initializer """
26 # Call the parent class initializer
27 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
28
29 # Variables that will hold sprite lists
30 self.player_list = None
31 self.coin_list = None
32
33 # Set up the player info
34 self.player_sprite = None
35
36 # Set up sprite that will serve as trigger
37 self.trigger_sprite = None
38
39 # Don't show the mouse cursor
40 self.set_mouse_visible(False)
41
42 self.background_color = arcade.color.AMAZON
43
44 def setup(self):
45 """ Set up the game and initialize the variables. """
46
47 # Sprite lists
48 self.player_list = arcade.SpriteList()
49 self.coin_list = arcade.SpriteList()
50
51 # Set up the player
52 # Character image from kenney.nl
53 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
54 scale=SPRITE_SCALING_PLAYER)
55 self.player_sprite.center_x = 50
56 self.player_sprite.center_y = 150
57 self.player_list.append(self.player_sprite)
58
59 # Create the sprites
60 for x in range(100, 800, 100):
61 coin = arcade.Sprite(":resources:images/items/coinGold.png", scale=0.3, center_x=x, center_y=400)
62 coin.intensity = 'dim'
63 coin.alpha = 64
64 self.coin_list.append(coin)
65
66 # Create trigger
67 self.trigger_sprite = arcade.Sprite(":resources:images/pinball/bumper.png", scale=0.5,
68 center_x=750, center_y=50)
69
70 def on_draw(self):
71 """ Draw everything """
72 self.clear()
73 self.coin_list.draw()
74 self.trigger_sprite.draw()
75 self.player_list.draw()
76
77 # Put the instructions on the screen.
78 instructions1 = "Touch a coin to set its intensity property to 'bright'."
79 arcade.draw_text(instructions1, 10, 90, arcade.color.WHITE, 14)
80 instructions2 = "Touch the trigger at the bottom-right to destroy all 'bright' sprites."
81 arcade.draw_text(instructions2, 10, 70, arcade.color.WHITE, 14)
82
83 # Query the property on the coins and show results.
84 coins_are_bright = [coin.intensity == 'bright' for coin in self.coin_list]
85 output_any = f"Any sprites have intensity=bright? : {any(coins_are_bright)}"
86 arcade.draw_text(output_any, 10, 40, arcade.color.WHITE, 14)
87 output_all = f"All sprites have intensity=bright? : {all(coins_are_bright)}"
88 arcade.draw_text(output_all, 10, 20, arcade.color.WHITE, 14)
89
90 def on_mouse_motion(self, x, y, dx, dy):
91 """ Handle Mouse Motion """
92
93 # Move the center of the player sprite to match the mouse x, y
94 self.player_sprite.center_x = x
95 self.player_sprite.center_y = y
96
97 def on_update(self, delta_time):
98 """ Movement and game logic """
99
100 # Call update on all sprites (The sprites don't do much in this
101 # example though.)
102 self.coin_list.update()
103
104 # Generate a list of all sprites that collided with the player.
105 coins_hit_list = arcade.check_for_collision_with_list(self.player_sprite, self.coin_list)
106
107 # Loop through each colliding sprite to set intensity=bright
108 for coin in coins_hit_list:
109 coin.intensity = 'bright'
110 coin.alpha = 255
111
112 hit_trigger = arcade.check_for_collision(self.player_sprite, self.trigger_sprite)
113 if hit_trigger:
114 intense_sprites = [sprite for sprite in self.coin_list if sprite.intensity == 'bright']
115 for coin in intense_sprites:
116 coin.remove_from_sprite_lists()
117
118
119def main():
120 """ Main function """
121 window = MyGame()
122 window.setup()
123 arcade.run()
124
125
126if __name__ == "__main__":
127 main()