随意放置硬币,但要远离墙壁和其他硬币#
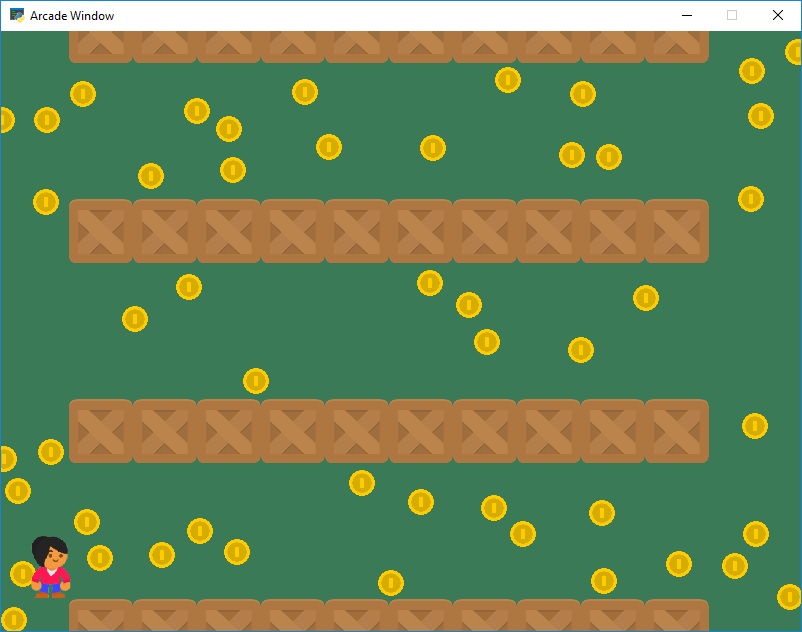
sprite_no_coins_on_walls.py#
1"""
2No coins on the walls
3
4Simple program to show basic sprite usage. Specifically, create coin sprites that
5aren't on top of any walls, and don't have coins on top of each other.
6
7Artwork from https://kenney.nl
8
9If Python and Arcade are installed, this example can be run from the command line with:
10python -m arcade.examples.sprite_no_coins_on_walls
11"""
12import arcade
13import random
14
15SPRITE_SCALING = 0.5
16SPRITE_SCALING_COIN = 0.2
17
18SCREEN_WIDTH = 800
19SCREEN_HEIGHT = 600
20SCREEN_TITLE = "Sprite No Coins on Walls Example"
21
22NUMBER_OF_COINS = 50
23
24MOVEMENT_SPEED = 5
25
26
27class MyGame(arcade.Window):
28 """ Main application class. """
29
30 def __init__(self, width, height, title):
31 """
32 Initializer
33 """
34 super().__init__(width, height, title)
35
36 # Sprite lists
37 self.player_list = None
38 self.coin_list = None
39
40 # Set up the player
41 self.player_sprite = None
42 self.wall_list = None
43 self.physics_engine = None
44
45 def setup(self):
46 """ Set up the game and initialize the variables. """
47
48 # Sprite lists
49 self.player_list = arcade.SpriteList()
50 self.wall_list = arcade.SpriteList()
51 self.coin_list = arcade.SpriteList()
52
53 # Set up the player
54 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
55 scale=SPRITE_SCALING)
56 self.player_sprite.center_x = 50
57 self.player_sprite.center_y = 64
58 self.player_list.append(self.player_sprite)
59
60 # -- Set up the walls
61 # Create a series of horizontal walls
62 for y in range(0, 800, 200):
63 for x in range(100, 700, 64):
64 wall = arcade.Sprite(":resources:images/tiles/boxCrate_double.png", scale=SPRITE_SCALING)
65 wall.center_x = x
66 wall.center_y = y
67 self.wall_list.append(wall)
68
69 # -- Randomly place coins where there are no walls
70 # Create the coins
71 for i in range(NUMBER_OF_COINS):
72
73 # Create the coin instance
74 # Coin image from kenney.nl
75 coin = arcade.Sprite(":resources:images/items/coinGold.png", scale=SPRITE_SCALING_COIN)
76
77 # --- IMPORTANT PART ---
78
79 # Boolean variable if we successfully placed the coin
80 coin_placed_successfully = False
81
82 # Keep trying until success
83 while not coin_placed_successfully:
84 # Position the coin
85 coin.center_x = random.randrange(SCREEN_WIDTH)
86 coin.center_y = random.randrange(SCREEN_HEIGHT)
87
88 # See if the coin is hitting a wall
89 wall_hit_list = arcade.check_for_collision_with_list(coin, self.wall_list)
90
91 # See if the coin is hitting another coin
92 coin_hit_list = arcade.check_for_collision_with_list(coin, self.coin_list)
93
94 if len(wall_hit_list) == 0 and len(coin_hit_list) == 0:
95 # It is!
96 coin_placed_successfully = True
97
98 # Add the coin to the lists
99 self.coin_list.append(coin)
100
101 # --- END OF IMPORTANT PART ---
102
103 self.physics_engine = arcade.PhysicsEngineSimple(self.player_sprite, self.wall_list)
104
105 # Set the background color
106 self.background_color = arcade.color.AMAZON
107
108 def on_draw(self):
109 """ Render the screen. """
110
111 # This command has to happen before we start drawing
112 self.clear()
113
114 # Draw all the sprites.
115 self.wall_list.draw()
116 self.coin_list.draw()
117 self.player_list.draw()
118
119 def on_key_press(self, key, modifiers):
120 """ Called whenever a key is pressed. """
121
122 if key == arcade.key.UP:
123 self.player_sprite.change_y = MOVEMENT_SPEED
124 elif key == arcade.key.DOWN:
125 self.player_sprite.change_y = -MOVEMENT_SPEED
126 elif key == arcade.key.LEFT:
127 self.player_sprite.change_x = -MOVEMENT_SPEED
128 elif key == arcade.key.RIGHT:
129 self.player_sprite.change_x = MOVEMENT_SPEED
130
131 def on_key_release(self, key, modifiers):
132 """ Called when the user releases a key. """
133
134 if key == arcade.key.UP or key == arcade.key.DOWN:
135 self.player_sprite.change_y = 0
136 elif key == arcade.key.LEFT or key == arcade.key.RIGHT:
137 self.player_sprite.change_x = 0
138
139 def on_update(self, delta_time):
140 """ Movement and game logic """
141
142 # Call update on all sprites (The sprites don't do much in this
143 # example though.)
144 self.physics_engine.update()
145
146
147def main():
148 """ Main function """
149 window = MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
150 window.setup()
151 arcade.run()
152
153
154if __name__ == "__main__":
155 main()