与墙一起移动#
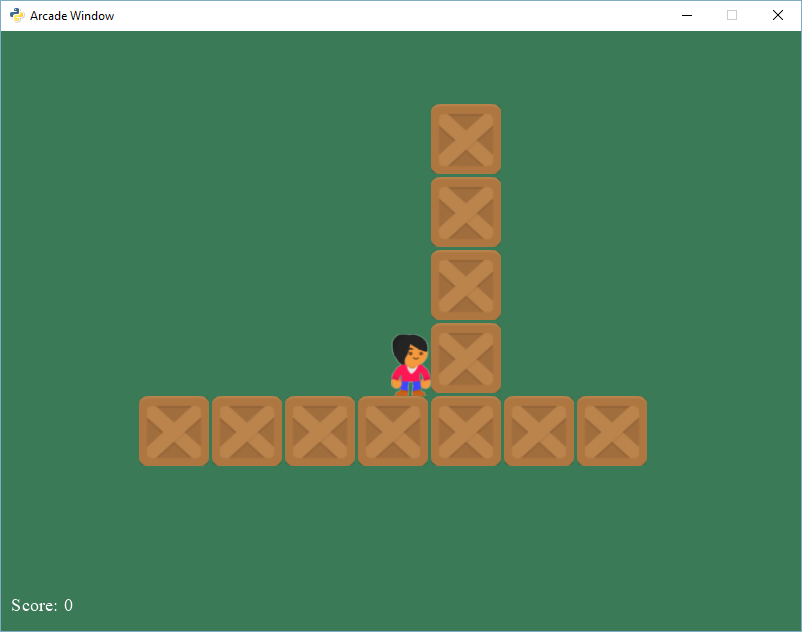
sprite_move_walls.py#
1"""
2Sprite Move With Walls
3
4Simple program to show basic sprite usage.
5
6Artwork from https://kenney.nl
7
8If Python and Arcade are installed, this example can be run from the command line with:
9python -m arcade.examples.sprite_move_walls
10"""
11
12import arcade
13
14SPRITE_SCALING = 0.5
15
16SCREEN_WIDTH = 800
17SCREEN_HEIGHT = 600
18SCREEN_TITLE = "Sprite Move with Walls Example"
19
20MOVEMENT_SPEED = 5
21
22
23class MyGame(arcade.Window):
24 """ Main application class. """
25
26 def __init__(self, width, height, title):
27 """
28 Initializer
29 """
30 super().__init__(width, height, title)
31
32 # Sprite lists
33 self.coin_list = None
34 self.wall_list = None
35 self.player_list = None
36
37 # Set up the player
38 self.player_sprite = None
39 self.physics_engine = None
40
41 def setup(self):
42 """ Set up the game and initialize the variables. """
43
44 # Sprite lists
45 self.player_list = arcade.SpriteList()
46 self.wall_list = arcade.SpriteList()
47
48 # Set up the player
49 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
50 scale=SPRITE_SCALING)
51 self.player_sprite.center_x = 50
52 self.player_sprite.center_y = 64
53 self.player_list.append(self.player_sprite)
54
55 # -- Set up the walls
56 # Create a row of boxes
57 for x in range(173, 650, 64):
58 wall = arcade.Sprite(":resources:images/tiles/boxCrate_double.png",
59 scale=SPRITE_SCALING)
60 wall.center_x = x
61 wall.center_y = 200
62 self.wall_list.append(wall)
63
64 # Create a column of boxes
65 for y in range(273, 500, 64):
66 wall = arcade.Sprite(":resources:images/tiles/boxCrate_double.png",
67 scale=SPRITE_SCALING)
68 wall.center_x = 465
69 wall.center_y = y
70 self.wall_list.append(wall)
71
72 self.physics_engine = arcade.PhysicsEngineSimple(self.player_sprite,
73 self.wall_list)
74
75 # Set the background color
76 self.background_color = arcade.color.AMAZON
77
78 def on_draw(self):
79 """
80 Render the screen.
81 """
82
83 # This command has to happen before we start drawing
84 self.clear()
85
86 # Draw all the sprites.
87 self.wall_list.draw()
88 self.player_list.draw()
89
90 def on_key_press(self, key, modifiers):
91 """Called whenever a key is pressed. """
92
93 if key == arcade.key.UP:
94 self.player_sprite.change_y = MOVEMENT_SPEED
95 elif key == arcade.key.DOWN:
96 self.player_sprite.change_y = -MOVEMENT_SPEED
97 elif key == arcade.key.LEFT:
98 self.player_sprite.change_x = -MOVEMENT_SPEED
99 elif key == arcade.key.RIGHT:
100 self.player_sprite.change_x = MOVEMENT_SPEED
101
102 def on_key_release(self, key, modifiers):
103 """Called when the user releases a key. """
104
105 if key == arcade.key.UP or key == arcade.key.DOWN:
106 self.player_sprite.change_y = 0
107 elif key == arcade.key.LEFT or key == arcade.key.RIGHT:
108 self.player_sprite.change_x = 0
109
110 def on_update(self, delta_time):
111 """ Movement and game logic """
112
113 # Call update on all sprites (The sprites don't do much in this
114 # example though.)
115 self.physics_engine.update()
116
117
118def main():
119 """ Main function """
120 window = MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
121 window.setup()
122 arcade.run()
123
124
125if __name__ == "__main__":
126 main()