摄像机抖动#
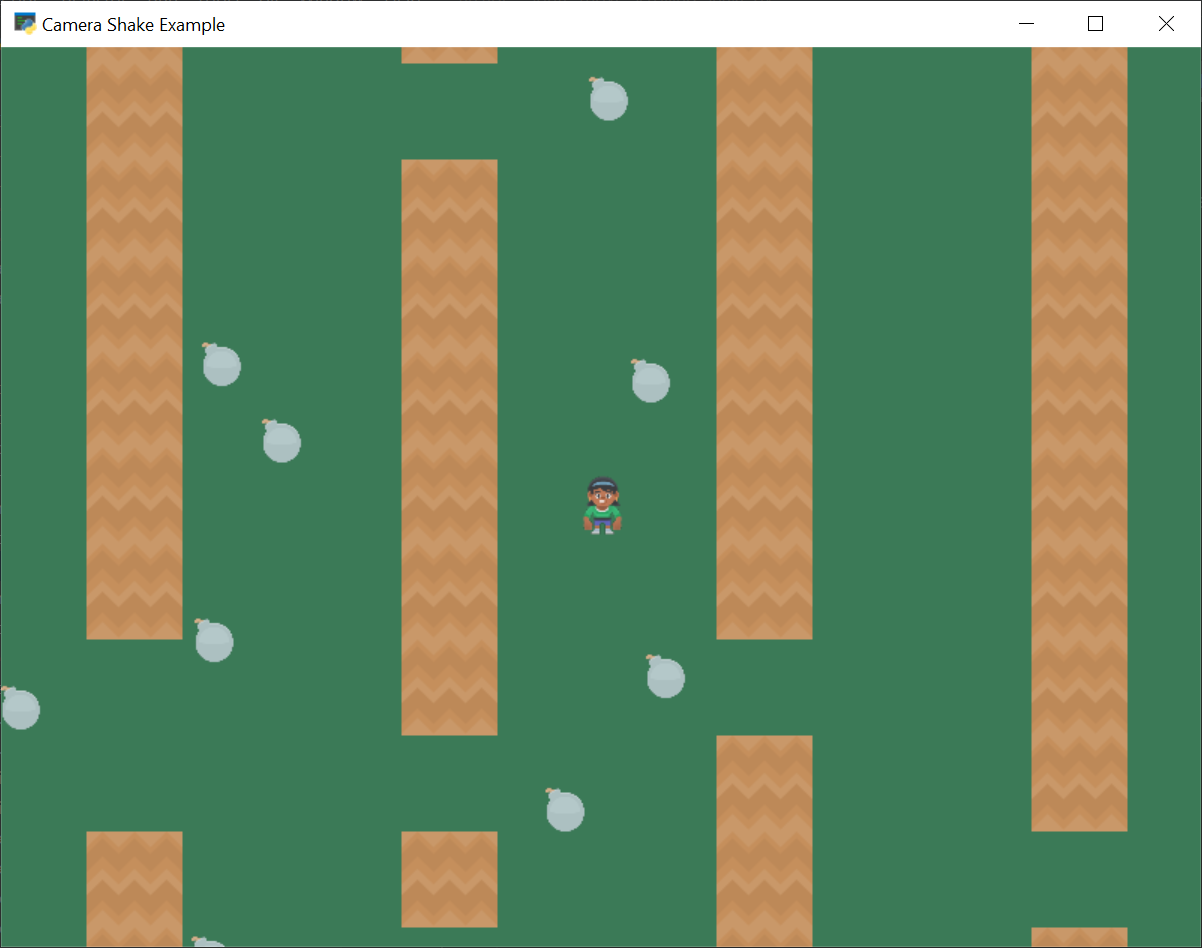
您可以使相机抖动,就像这个示例在玩家遇到炸弹时所做的那样。请参见下面突出显示的行。
sprite_move_scrolling_shake.py#
1"""
2Scroll around a large screen.
3
4Artwork from https://kenney.nl
5
6If Python and Arcade are installed, this example can be run from the command line with:
7python -m arcade.examples.sprite_move_scrolling_shake
8"""
9
10import random
11# import math
12import arcade
13
14SPRITE_SCALING = 0.5
15
16DEFAULT_SCREEN_WIDTH = 800
17DEFAULT_SCREEN_HEIGHT = 600
18SCREEN_TITLE = "Camera Shake Example"
19
20# How many pixels to keep as a minimum margin between the character
21# and the edge of the screen.
22VIEWPORT_MARGIN = 220
23
24# How fast the camera pans to the player. 1.0 is instant.
25CAMERA_SPEED = 0.1
26
27# How fast the character moves
28PLAYER_MOVEMENT_SPEED = 7
29
30BOMB_COUNT = 50
31PLAYING_FIELD_WIDTH = 1600
32PLAYING_FIELD_HEIGHT = 1600
33
34
35class MyGame(arcade.Window):
36 """ Main application class. """
37
38 def __init__(self, width, height, title):
39 """
40 Initializer
41 """
42 super().__init__(width, height, title, resizable=True)
43
44 # Sprite lists
45 self.player_list = None
46 self.wall_list = None
47 self.bomb_list = None
48
49 # Set up the player
50 self.player_sprite = None
51
52 # Physics engine so we don't run into walls.
53 self.physics_engine = None
54
55 # Create the cameras. One for the GUI, one for the sprites.
56 # We scroll the 'sprite world' but not the GUI.
57 self.camera_sprites = arcade.camera.Camera2D()
58 self.camera_gui = arcade.camera.Camera2D()
59
60 self.camera_shake = arcade.camera.grips.ScreenShake2D(self.camera_sprites.view_data,
61 max_amplitude=15.0,
62 acceleration_duration=0.1,
63 falloff_time=0.5,
64 shake_frequency=10.0)
65
66 self.explosion_sound = arcade.load_sound(":resources:sounds/explosion1.wav")
67
68 def setup(self):
69 """ Set up the game and initialize the variables. """
70
71 # Sprite lists
72 self.player_list = arcade.SpriteList()
73 self.wall_list = arcade.SpriteList()
74 self.bomb_list = arcade.SpriteList()
75
76 # Set up the player
77 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
78 scale=0.4)
79 self.player_sprite.center_x = 512
80 self.player_sprite.center_y = 512
81 self.player_list.append(self.player_sprite)
82
83 # -- Set up several columns of walls
84 for x in range(200, PLAYING_FIELD_WIDTH, 210):
85 for y in range(0, PLAYING_FIELD_HEIGHT, 64):
86 # Randomly skip a box so the player can find a way through
87 if random.randrange(5) > 0:
88 wall = arcade.Sprite(":resources:images/tiles/grassCenter.png", scale=SPRITE_SCALING)
89 wall.center_x = x
90 wall.center_y = y
91 self.wall_list.append(wall)
92
93 for i in range(BOMB_COUNT):
94 bomb = arcade.Sprite(":resources:images/tiles/bomb.png", scale=0.25)
95 placed = False
96 while not placed:
97 bomb.center_x = random.randrange(PLAYING_FIELD_WIDTH)
98 bomb.center_y = random.randrange(PLAYING_FIELD_HEIGHT)
99 if not arcade.check_for_collision_with_list(bomb, self.wall_list):
100 placed = True
101 self.bomb_list.append(bomb)
102
103 self.physics_engine = arcade.PhysicsEngineSimple(self.player_sprite, self.wall_list)
104
105 # Set the background color
106 self.background_color = arcade.color.AMAZON
107
108 def on_draw(self):
109 """
110 Render the screen.
111 """
112
113 # This command has to happen before we start drawing
114 self.clear()
115
116 # Select the camera we'll use to draw all our sprites
117 self.camera_shake.update_camera()
118 self.camera_sprites.use()
119
120 # Draw all the sprites.
121 self.wall_list.draw()
122 self.bomb_list.draw()
123 self.player_list.draw()
124
125 # Readjust the camera's screen_shake
126 self.camera_shake.readjust_camera()
127
128 def on_key_press(self, key, modifiers):
129 """Called whenever a key is pressed. """
130
131 if key == arcade.key.UP:
132 self.player_sprite.change_y = PLAYER_MOVEMENT_SPEED
133 elif key == arcade.key.DOWN:
134 self.player_sprite.change_y = -PLAYER_MOVEMENT_SPEED
135 elif key == arcade.key.LEFT:
136 self.player_sprite.change_x = -PLAYER_MOVEMENT_SPEED
137 elif key == arcade.key.RIGHT:
138 self.player_sprite.change_x = PLAYER_MOVEMENT_SPEED
139
140 def on_key_release(self, key, modifiers):
141 """Called when the user releases a key. """
142
143 if key == arcade.key.UP or key == arcade.key.DOWN:
144 self.player_sprite.change_y = 0
145 elif key == arcade.key.LEFT or key == arcade.key.RIGHT:
146 self.player_sprite.change_x = 0
147
148 def on_update(self, delta_time):
149 """ Movement and game logic """
150
151 # Call update on all sprites (The sprites don't do much in this
152 # example though.)
153 self.physics_engine.update()
154 self.camera_shake.update(delta_time)
155
156 # Scroll the screen to the player
157 self.scroll_to_player()
158
159 hit_list = arcade.check_for_collision_with_list(self.player_sprite, self.bomb_list)
160 for bomb in hit_list:
161 # Remove the bomb and go 'boom'
162 bomb.remove_from_sprite_lists()
163 self.explosion_sound.play()
164
165 self.camera_shake.start()
166
167 def scroll_to_player(self):
168 """
169 Scroll the window to the player.
170
171 if CAMERA_SPEED is 1, the camera will immediately move to the desired position.
172 Anything between 0 and 1 will have the camera move to the location with a smoother
173 pan.
174 """
175
176 position = (
177 self.player_sprite.center_x,
178 self.player_sprite.center_y
179 )
180 self.camera_sprites.position = arcade.math.lerp_2d(self.camera_sprites.position, position, CAMERA_SPEED)
181
182 def on_resize(self, width: int, height: int):
183 """
184 Resize window
185 Handle the user grabbing the edge and resizing the window.
186 """
187 super().on_resize(width, height)
188 self.camera_sprites.match_screen(and_projection=True)
189 self.camera_gui.match_screen(and_projection=True)
190
191
192def main():
193 """ Main function """
194 window = MyGame(DEFAULT_SCREEN_WIDTH, DEFAULT_SCREEN_HEIGHT, SCREEN_TITLE)
195 window.setup()
196 arcade.run()
197
198
199if __name__ == "__main__":
200 main()