纯文本按钮#
有关图形用户界面系统的介绍,请参见 图形用户界面概念 。
这个 arcade.gui.UIFlatButton
是一个带有文本标签的简单按钮。它没有任何三维外观。
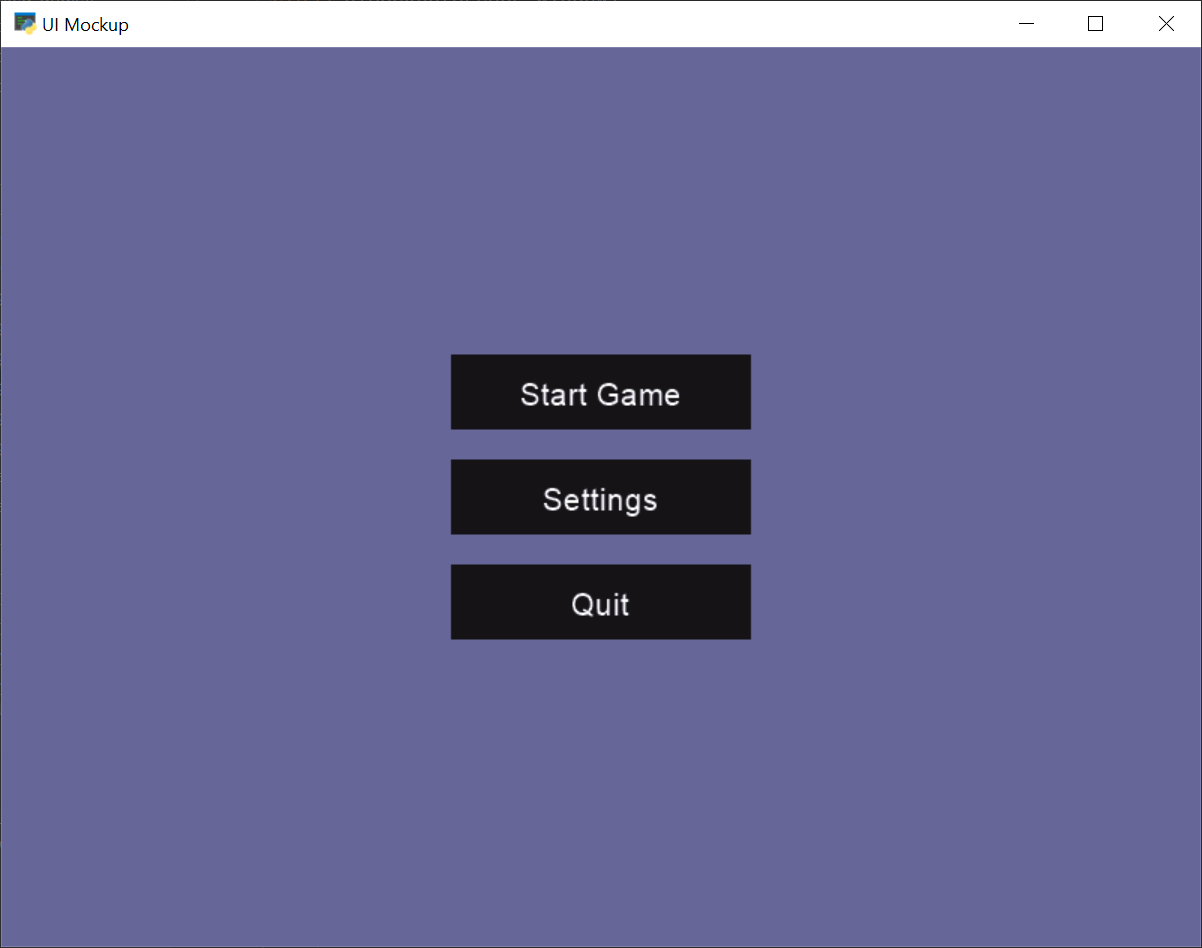
有三种方法可以处理按钮单击事件:
创建一个父类为 arcade.UIFlatButton 并实现一个名为 on_click 。
创建一个按钮,然后将 on_click 属性设置为等于要调用的函数。
创建一个按钮。然后使用修饰符指定在调用 on_click 事件为该按钮发生。
此代码显示上述三种方式中的每一种。代码应该选择三种方法中的一种,并通过程序对其进行标准化。不要编写同时使用这三种方法的代码。
gui_flat_button.py#
1"""
2Example code showing how to create a button,
3and the three ways to process button events.
4
5If Python and Arcade are installed, this example can be run from the command line with:
6python -m arcade.examples.gui_flat_button
7"""
8import arcade
9import arcade.gui
10
11# --- Method 1 for handling click events,
12# Create a child class.
13import arcade.gui.widgets.buttons
14import arcade.gui.widgets.layout
15
16
17class QuitButton(arcade.gui.widgets.buttons.UIFlatButton):
18 def on_click(self, event: arcade.gui.UIOnClickEvent):
19 arcade.exit()
20
21
22class MyView(arcade.View):
23 def __init__(self):
24 super().__init__()
25 # --- Required for all code that uses UI element,
26 # a UIManager to handle the UI.
27 self.ui = arcade.gui.UIManager()
28
29 # Create a vertical BoxGroup to align buttons
30 self.v_box = arcade.gui.widgets.layout.UIBoxLayout(space_between=20)
31
32 # Create the buttons
33 start_button = arcade.gui.widgets.buttons.UIFlatButton(
34 text="Start Game", width=200
35 )
36 self.v_box.add(start_button)
37
38 settings_button = arcade.gui.widgets.buttons.UIFlatButton(
39 text="Settings", width=200
40 )
41 self.v_box.add(settings_button)
42
43 # Again, method 1. Use a child class to handle events.
44 quit_button = QuitButton(text="Quit", width=200)
45 self.v_box.add(quit_button)
46
47 # --- Method 2 for handling click events,
48 # assign self.on_click_start as callback
49 start_button.on_click = self.on_click_start
50
51 # --- Method 3 for handling click events,
52 # use a decorator to handle on_click events
53 @settings_button.event("on_click")
54 def on_click_settings(event):
55 print("Settings:", event)
56
57 # Create a widget to hold the v_box widget, that will center the buttons
58 ui_anchor_layout = arcade.gui.widgets.layout.UIAnchorLayout()
59 ui_anchor_layout.add(child=self.v_box, anchor_x="center_x", anchor_y="center_y")
60
61 self.ui.add(ui_anchor_layout)
62
63 def on_show_view(self):
64 self.window.background_color = arcade.color.DARK_BLUE_GRAY
65 # Enable UIManager when view is shown to catch window events
66 self.ui.enable()
67
68 def on_hide_view(self):
69 # Disable UIManager when view gets inactive
70 self.ui.disable()
71
72 def on_click_start(self, event):
73 print("Start:", event)
74
75 def on_draw(self):
76 self.clear()
77 self.ui.draw()
78
79
80if __name__ == '__main__':
81 window = arcade.Window(800, 600, "UIExample", resizable=True)
82 window.show_view(MyView())
83 window.run()
看见 arcade.gui.UIBoxLayout
和 arcade.gui.UIAnchorLayout
有关定位按钮的详细信息,请参阅。例如,对第31行的更改如下:
self.v_box = arcade.gui.widgets.layout.UIBoxLayout(space_between=20, vertical=False);
和第60行:
ui_anchor_layout.add(child=self.v_box,
anchor_x="left",
anchor_y="bottom",
align_x=10,
align_y=10);
在上面的代码中,将水平对齐按钮,并将它们锚定到窗口的左下角,边距为10px。
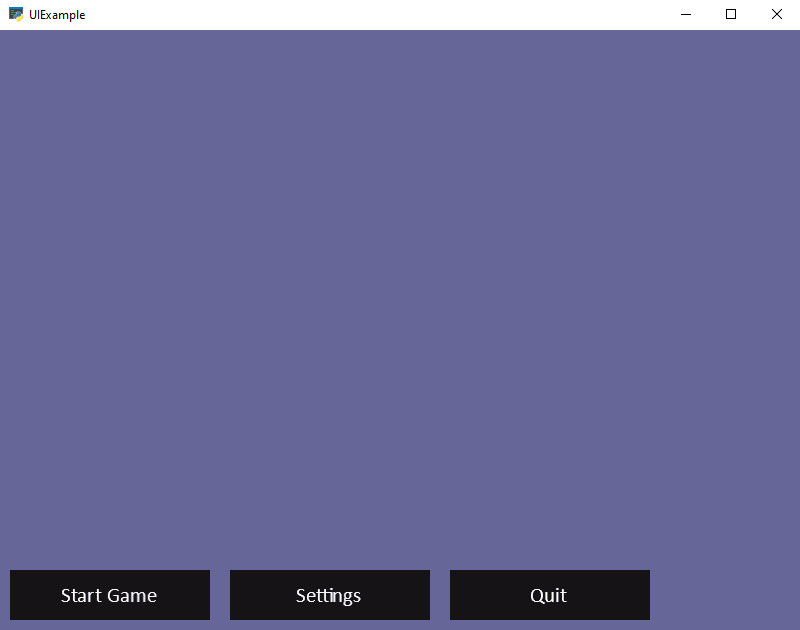