全屏示例#
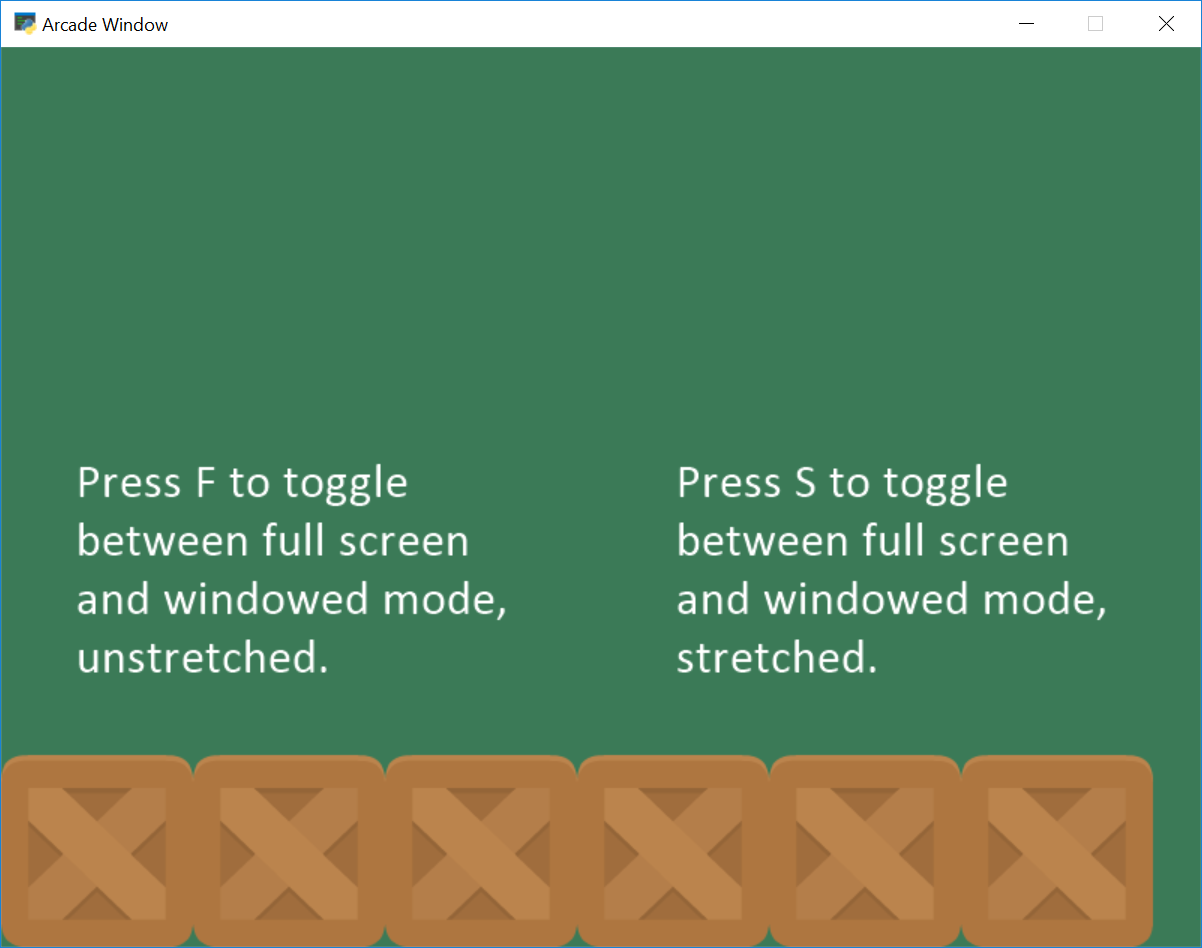
full_screen_example.py#
1"""
2Use sprites to scroll around a large screen.
3
4Simple program to show basic sprite usage.
5
6Artwork from https://kenney.nl
7
8If Python and Arcade are installed, this example can be run from the command line with:
9python -m arcade.examples.full_screen_example
10"""
11
12import arcade
13
14SPRITE_SCALING = 0.5
15
16SCREEN_WIDTH = 800
17SCREEN_HEIGHT = 600
18SCREEN_TITLE = "Full Screen Example"
19
20# How many pixels to keep as a minimum margin between the character
21# and the edge of the screen.
22VIEWPORT_MARGIN = 40
23
24MOVEMENT_SPEED = 5
25
26
27class MyGame(arcade.Window):
28 """ Main application class. """
29
30 def __init__(self):
31 """
32 Initializer
33 """
34 # Open a window in full screen mode. Remove fullscreen=True if
35 # you don't want to start this way.
36 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE, fullscreen=True)
37
38 # This will get the size of the window, and set the viewport to match.
39 # So if the window is 1000x1000, then so will our viewport. If
40 # you want something different, then use those coordinates instead.
41 self.background_color = arcade.color.AMAZON
42 self.example_image = arcade.load_texture(":resources:images/tiles/boxCrate_double.png")
43
44 # The camera used to update the viewport and projection on screen resize.
45 # The position needs to be set to the bottom left corner.
46 self.cam = arcade.camera.Camera2D.from_raw_data(position=(0.0, 0.0))
47
48 def on_draw(self):
49 """
50 Render the screen.
51 """
52
53 self.clear()
54
55 # Get viewport dimensions
56 screen_width, screen_height = int(self.cam.projection_width), int(self.cam.projection_height)
57
58 text_size = 18
59 # Draw text on the screen so the user has an idea of what is happening
60 arcade.draw_text("Press F to toggle between full screen and windowed mode, unstretched.",
61 screen_width // 2, screen_height // 2 - 20,
62 arcade.color.WHITE, text_size, anchor_x="center")
63 arcade.draw_text("Press S to toggle between full screen and windowed mode, stretched.",
64 screen_width // 2, screen_height // 2 + 20,
65 arcade.color.WHITE, text_size, anchor_x="center")
66
67 # Draw some boxes on the bottom so we can see how they change
68 for x in range(64, 800, 128):
69 y = 64
70 width = 128
71 height = 128
72 arcade.draw_texture_rectangle(x, y, width, height, self.example_image)
73
74 def on_key_press(self, key, modifiers):
75 """Called whenever a key is pressed. """
76 if key == arcade.key.F:
77 # User hits f. Flip between full and not full screen.
78 self.set_fullscreen(not self.fullscreen)
79
80 # Get the window coordinates. Match viewport to window coordinates
81 # so there is a one-to-one mapping.
82 self.cam.projection = 0, self.width, 0, self.height
83 self.cam.viewport = 0, 0, self.width, self.height
84 self.cam.use()
85
86 if key == arcade.key.S:
87 # User hits s. Flip between full and not full screen.
88 self.set_fullscreen(not self.fullscreen)
89
90 # Instead of a one-to-one mapping, stretch/squash window to match the
91 # constants. This does NOT respect aspect ratio. You'd need to
92 # do a bit of math for that.
93 self.cam.projection = 0, SCREEN_WIDTH, 0, SCREEN_HEIGHT
94 self.cam.viewport = 0, 0, self.width, self.height
95 self.cam.use()
96
97
98def main():
99 """ Main function """
100 MyGame()
101 arcade.run()
102
103
104if __name__ == "__main__":
105 main()