绘图基本体#
此示例说明如何使用绘图命令。对于新入门的程序员来说,这是一个很好的起点,因为他们在绘制图像之前不需要知道如何定义函数或类。
看见 喜笑颜开 以获取操作中的绘制基元的示例。为简单起见,此示例没有许多通常在 使用窗口类启动模板 。
备注
这里显示的画法很简单,但速度很慢。现代图形通常是一批绘制的,这种技术在本例中没有显示。
要查找所有可用命令,请在 API指数 。
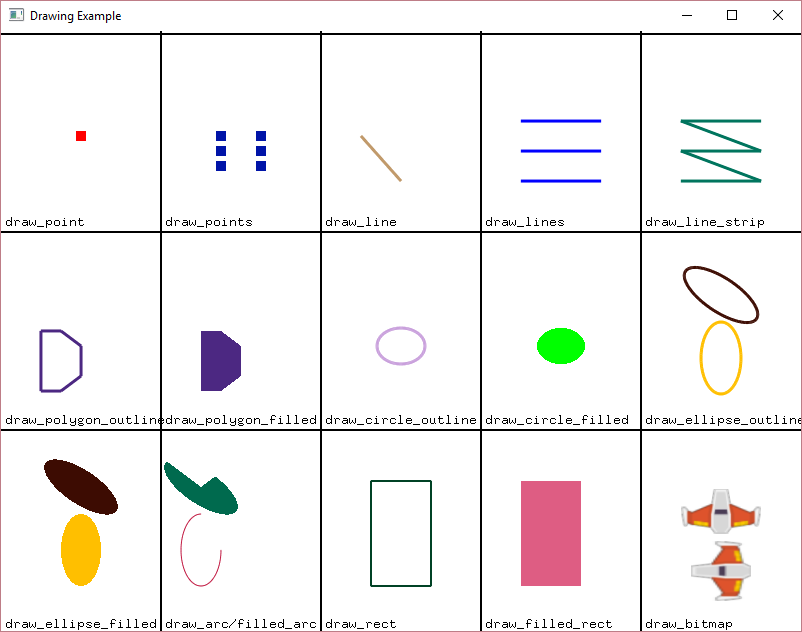
drawing_primitives.py#
1"""
2Example "Arcade" library code.
3
4This example shows the drawing primitives and how they are used.
5It does not assume the programmer knows how to define functions or classes
6yet.
7
8API documentation for the draw commands can be found here:
9https://api.arcade.academy/en/latest/quick_index.html
10
11A video explaining this example can be found here:
12https://vimeo.com/167158158
13
14If Python and Arcade are installed, this example can be run from the command line with:
15python -m arcade.examples.drawing_primitives
16"""
17
18# Import the Arcade library. If this fails, then try following the instructions
19# for how to install arcade:
20# https://api.arcade.academy/en/latest/install/index.html
21import arcade
22
23# Open the window. Set the window title and dimensions (width and height)
24arcade.open_window(600, 600, "Drawing Primitives Example")
25
26# Set the background color to white
27# For a list of named colors see
28# https://api.arcade.academy/en/latest/arcade.color.html
29# Colors can also be specified in (red, green, blue) format and
30# (red, green, blue, alpha) format.
31arcade.set_background_color(arcade.color.WHITE)
32
33# Start the render process. This must be done before any drawing commands.
34arcade.start_render()
35
36# Draw a grid
37# Draw vertical lines every 120 pixels
38for x in range(0, 601, 120):
39 arcade.draw_line(x, 0, x, 600, arcade.color.BLACK, 2)
40
41# Draw horizontal lines every 200 pixels
42for y in range(0, 601, 200):
43 arcade.draw_line(0, y, 800, y, arcade.color.BLACK, 2)
44
45# Draw a point
46arcade.draw_text("draw_point", 3, 405, arcade.color.BLACK, 12)
47arcade.draw_point(60, 495, arcade.color.RED, 10)
48
49# Draw a set of points
50arcade.draw_text("draw_points", 123, 405, arcade.color.BLACK, 12)
51point_list = ((165, 495),
52 (165, 480),
53 (165, 465),
54 (195, 495),
55 (195, 480),
56 (195, 465))
57arcade.draw_points(point_list, arcade.color.ZAFFRE, 10)
58
59# Draw a line
60arcade.draw_text("draw_line", 243, 405, arcade.color.BLACK, 12)
61arcade.draw_line(270, 495, 300, 450, arcade.color.WOOD_BROWN, 3)
62
63# Draw a set of lines
64arcade.draw_text("draw_lines", 363, 405, arcade.color.BLACK, 12)
65point_list = ((390, 450),
66 (450, 450),
67 (390, 480),
68 (450, 480),
69 (390, 510),
70 (450, 510)
71 )
72arcade.draw_lines(point_list, arcade.color.BLUE, 3)
73
74# Draw a line strip
75arcade.draw_text("draw_line_strip", 483, 405, arcade.color.BLACK, 12)
76point_list = ((510, 450),
77 (570, 450),
78 (510, 480),
79 (570, 480),
80 (510, 510),
81 (570, 510)
82 )
83arcade.draw_line_strip(point_list, arcade.color.TROPICAL_RAIN_FOREST, 3)
84
85# Draw a polygon
86arcade.draw_text("draw_polygon_outline", 3, 207, arcade.color.BLACK, 9)
87point_list = ((30, 240),
88 (45, 240),
89 (60, 255),
90 (60, 285),
91 (45, 300),
92 (30, 300))
93arcade.draw_polygon_outline(point_list, arcade.color.SPANISH_VIOLET, 3)
94
95# Draw a filled in polygon
96arcade.draw_text("draw_polygon_filled", 123, 207, arcade.color.BLACK, 9)
97point_list = ((150, 240),
98 (165, 240),
99 (180, 255),
100 (180, 285),
101 (165, 300),
102 (150, 300))
103arcade.draw_polygon_filled(point_list, arcade.color.SPANISH_VIOLET)
104
105# Draw an outline of a circle
106arcade.draw_text("draw_circle_outline", 243, 207, arcade.color.BLACK, 10)
107arcade.draw_circle_outline(300, 285, 18, arcade.color.WISTERIA, 3)
108
109# Draw a filled in circle
110arcade.draw_text("draw_circle_filled", 363, 207, arcade.color.BLACK, 10)
111arcade.draw_circle_filled(420, 285, 18, arcade.color.GREEN)
112
113# Draw an ellipse outline, and another one rotated
114arcade.draw_text("draw_ellipse_outline", 483, 207, arcade.color.BLACK, 10)
115arcade.draw_ellipse_outline(540, 273, 15, 36, arcade.color.AMBER, 3)
116arcade.draw_ellipse_outline(540, 336, 15, 36,
117 arcade.color.BLACK_BEAN, 3, 45)
118
119# Draw a filled ellipse, and another one rotated
120arcade.draw_text("draw_ellipse_filled", 3, 3, arcade.color.BLACK, 10)
121arcade.draw_ellipse_filled(60, 81, 15, 36, arcade.color.AMBER)
122arcade.draw_ellipse_filled(60, 144, 15, 36,
123 arcade.color.BLACK_BEAN, 45)
124
125# Draw an arc, and another one rotated
126arcade.draw_text("draw_arc/filled_arc", 123, 3, arcade.color.BLACK, 10)
127arcade.draw_arc_outline(150, 81, 15, 36,
128 arcade.color.BRIGHT_MAROON, 90, 360)
129arcade.draw_arc_filled(150, 144, 15, 36,
130 arcade.color.BOTTLE_GREEN, 90, 360, 45)
131
132# Draw an rectangle outline
133arcade.draw_text("draw_rect", 243, 3, arcade.color.BLACK, 10)
134arcade.draw_rectangle_outline(295, 100, 45, 65,
135 arcade.color.BRITISH_RACING_GREEN)
136arcade.draw_rectangle_outline(295, 160, 20, 45,
137 arcade.color.BRITISH_RACING_GREEN, 3, 45)
138
139# Draw a filled in rectangle
140arcade.draw_text("draw_filled_rect", 363, 3, arcade.color.BLACK, 10)
141arcade.draw_rectangle_filled(420, 100, 45, 65, arcade.color.BLUSH)
142arcade.draw_rectangle_filled(420, 160, 20, 40, arcade.color.BLUSH, 45)
143
144# Load and draw an image to the screen
145# Image from kenney.nl asset pack #1
146arcade.draw_text("draw_bitmap", 483, 3, arcade.color.BLACK, 12)
147texture = arcade.load_texture(":resources:images/space_shooter/playerShip1_orange.png")
148scale = .6
149arcade.draw_scaled_texture_rectangle(540, 120, texture, scale, 0)
150arcade.draw_scaled_texture_rectangle(540, 60, texture, scale, 45)
151
152# Finish the render.
153# Nothing will be drawn without this.
154# Must happen after all draw commands
155arcade.finish_render()
156
157# Keep the window up until someone closes it.
158arcade.run()