更好地通过键盘移动#
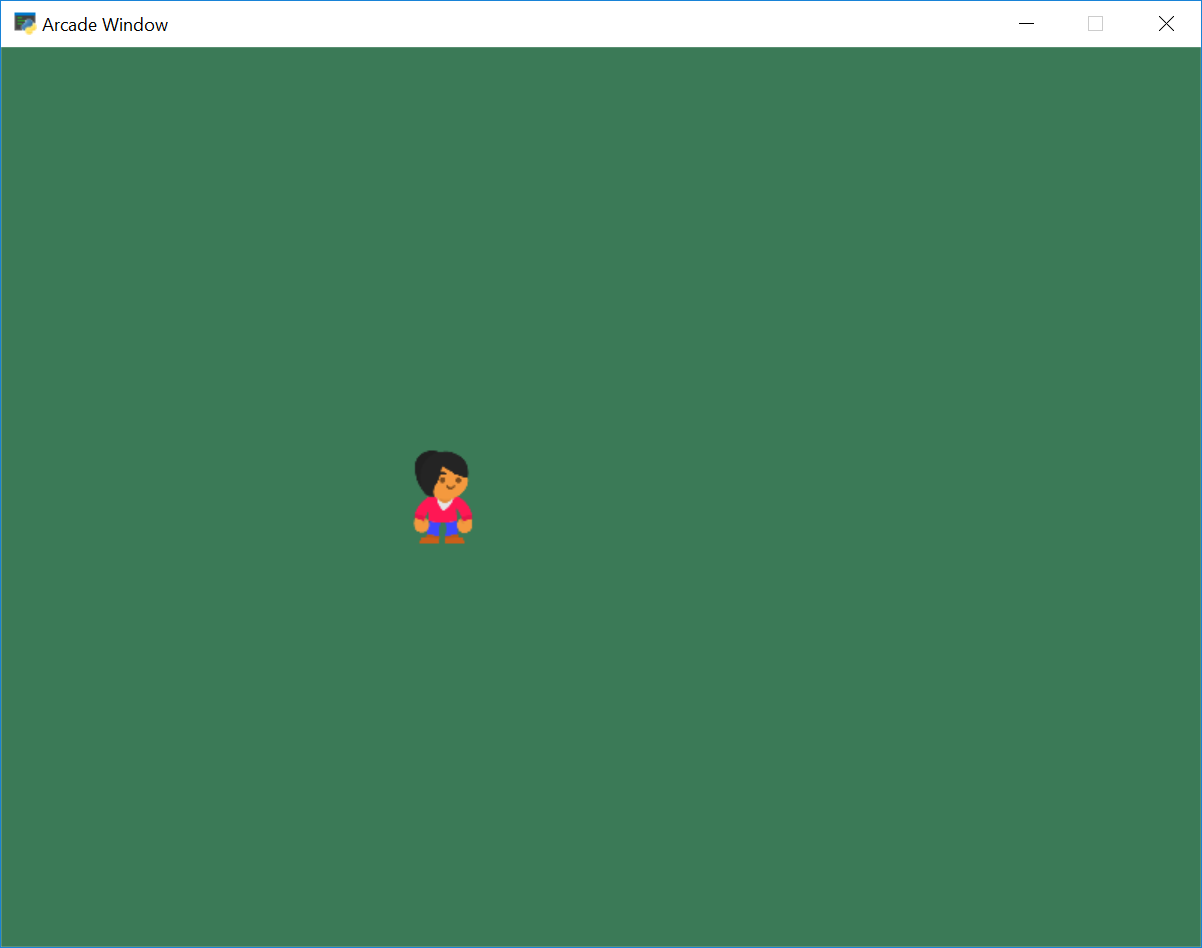
如果玩家按下左键,精灵应该向左移动。如果球员同时左击和右击,球员应该停下来。如果玩家松开左键,保持右键不动,玩家就应该向右移动。
处理击键的较简单方法将无法正确处理此问题。此代码跟踪哪个键是按下的还是向上的,并正确处理它。
请参见高亮显示的部分。
sprite_move_keyboard_better.py#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 | """ Better Move Sprite With Keyboard Simple program to show moving a sprite with the keyboard. This is slightly better than sprite_move_keyboard.py example in how it works, but also slightly more complex. Artwork from https://kenney.nl If Python and Arcade are installed, this example can be run from the command line with: python -m arcade.examples.sprite_move_keyboard_better """ import arcade SPRITE_SCALING = 0.5 SCREEN_WIDTH = 800 SCREEN_HEIGHT = 600 SCREEN_TITLE = "Better Move Sprite with Keyboard Example" MOVEMENT_SPEED = 5 class Player(arcade.Sprite): def update(self): """ Move the player """ # Move player. # Remove these lines if physics engine is moving player. self.center_x += self.change_x self.center_y += self.change_y # Check for out-of-bounds if self.left < 0: self.left = 0 elif self.right > SCREEN_WIDTH - 1: self.right = SCREEN_WIDTH - 1 if self.bottom < 0: self.bottom = 0 elif self.top > SCREEN_HEIGHT - 1: self.top = SCREEN_HEIGHT - 1 class MyGame(arcade.Window): """ Main application class. """ def __init__(self, width, height, title): """ Initializer """ # Call the parent class initializer super().__init__(width, height, title) # Variables that will hold sprite lists self.player_list = None # Set up the player info self.player_sprite = None # Track the current state of what key is pressed self.left_pressed = False self.right_pressed = False self.up_pressed = False self.down_pressed = False # Set the background color arcade.set_background_color(arcade.color.AMAZON) def setup(self): """ Set up the game and initialize the variables. """ # Sprite lists self.player_list = arcade.SpriteList() # Set up the player self.player_sprite = Player(":resources:images/animated_characters/female_person/femalePerson_idle.png", SPRITE_SCALING) self.player_sprite.center_x = 50 self.player_sprite.center_y = 50 self.player_list.append(self.player_sprite) def on_draw(self): """ Render the screen. """ # Clear the screen self.clear() # Draw all the sprites. self.player_list.draw() def update_player_speed(self): # Calculate speed based on the keys pressed self.player_sprite.change_x = 0 self.player_sprite.change_y = 0 if self.up_pressed and not self.down_pressed: self.player_sprite.change_y = MOVEMENT_SPEED elif self.down_pressed and not self.up_pressed: self.player_sprite.change_y = -MOVEMENT_SPEED if self.left_pressed and not self.right_pressed: self.player_sprite.change_x = -MOVEMENT_SPEED elif self.right_pressed and not self.left_pressed: self.player_sprite.change_x = MOVEMENT_SPEED def on_update(self, delta_time): """ Movement and game logic """ # Call update to move the sprite # If using a physics engine, call update player to rely on physics engine # for movement, and call physics engine here. self.player_list.update() def on_key_press(self, key, modifiers): """Called whenever a key is pressed. """ if key == arcade.key.UP: self.up_pressed = True self.update_player_speed() elif key == arcade.key.DOWN: self.down_pressed = True self.update_player_speed() elif key == arcade.key.LEFT: self.left_pressed = True self.update_player_speed() elif key == arcade.key.RIGHT: self.right_pressed = True self.update_player_speed() def on_key_release(self, key, modifiers): """Called when the user releases a key. """ if key == arcade.key.UP: self.up_pressed = False self.update_player_speed() elif key == arcade.key.DOWN: self.down_pressed = False self.update_player_speed() elif key == arcade.key.LEFT: self.left_pressed = False self.update_player_speed() elif key == arcade.key.RIGHT: self.right_pressed = False self.update_player_speed() def main(): """ Main function """ window = MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE) window.setup() arcade.run() if __name__ == "__main__": main() |