性能统计信息#
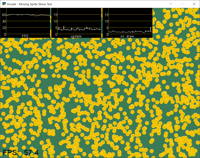
您可以轻松地包括显示FPS的图表,或显示处理调度事件所需的时间。看见 性能信息 。
属性打印出所有已调度事件的计数和平均时间。 arcade.print_timings()
功能。该函数的输出如下所示:
Event Count Average Time
-------------- ----- ------------
on_activate 1 0.0000
on_resize 1 0.0000
on_show 1 0.0000
update 59 0.0030
on_update 59 0.0000
on_expose 1 0.0000
on_draw 59 0.0021
on_mouse_enter 1 0.0000
on_mouse_motion 100 0.0000
performance_statistics.py#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 | """ Performance Statistic Displays Artwork from https://kenney.nl """ import random import arcade # --- Constants --- SPRITE_SCALING_COIN = 0.25 SPRITE_NATIVE_SIZE = 128 SPRITE_SIZE = int(SPRITE_NATIVE_SIZE * SPRITE_SCALING_COIN) SCREEN_WIDTH = 800 SCREEN_HEIGHT = 600 SCREEN_TITLE = "Arcade - Moving Sprite Stress Test" # Size of performance graphs and distance between them GRAPH_WIDTH = 200 GRAPH_HEIGHT = 120 GRAPH_MARGIN = 5 COIN_COUNT = 1500 arcade.enable_timings() class Coin(arcade.Sprite): """ Our coin sprite class """ def update(self): """ Update the sprite. """ # Updating the sprite this way is faster than x and y individually. self.position = (self.position[0] + self.change_x, self.position[1] + self.change_y) # Bounce the coin on the edge if self.position[0] < 0: self.change_x *= -1 elif self.position[0] > SCREEN_WIDTH: self.change_x *= -1 if self.position[1] < 0: self.change_y *= -1 elif self.position[1] > SCREEN_HEIGHT: self.change_y *= -1 class MyGame(arcade.Window): """ Our custom Window Class""" def __init__(self): """ Initializer """ # Call the parent class initializer super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE) # Variables that will hold sprite lists self.coin_list = None self.perf_graph_list = None self.frame_count = 0 arcade.set_background_color(arcade.color.AMAZON) def add_coins(self, amount): # Create the coins for i in range(amount): # Create the coin instance # Coin image from kenney.nl coin = Coin(":resources:images/items/coinGold.png", SPRITE_SCALING_COIN) # Position the coin coin.center_x = random.randrange(SPRITE_SIZE, SCREEN_WIDTH - SPRITE_SIZE) coin.center_y = random.randrange(SPRITE_SIZE, SCREEN_HEIGHT - SPRITE_SIZE) coin.change_x = random.randrange(-3, 4) coin.change_y = random.randrange(-3, 4) # Add the coin to the lists self.coin_list.append(coin) def setup(self): """ Set up the game and initialize the variables. """ # Sprite lists self.coin_list = arcade.SpriteList(use_spatial_hash=False) # Create some coins self.add_coins(COIN_COUNT) # Create a sprite list to put the performance graph into self.perf_graph_list = arcade.SpriteList() # Create the FPS performance graph graph = arcade.PerfGraph(GRAPH_WIDTH, GRAPH_HEIGHT, graph_data="FPS") graph.center_x = GRAPH_WIDTH / 2 graph.center_y = self.height - GRAPH_HEIGHT / 2 self.perf_graph_list.append(graph) # Create the on_update graph graph = arcade.PerfGraph(GRAPH_WIDTH, GRAPH_HEIGHT, graph_data="update") graph.center_x = GRAPH_WIDTH / 2 + (GRAPH_WIDTH + GRAPH_MARGIN) graph.center_y = self.height - GRAPH_HEIGHT / 2 self.perf_graph_list.append(graph) # Create the on_draw graph graph = arcade.PerfGraph(GRAPH_WIDTH, GRAPH_HEIGHT, graph_data="on_draw") graph.center_x = GRAPH_WIDTH / 2 + (GRAPH_WIDTH + GRAPH_MARGIN) * 2 graph.center_y = self.height - GRAPH_HEIGHT / 2 self.perf_graph_list.append(graph) def on_draw(self): """ Draw everything """ # Clear the screen self.clear() # Draw all the sprites self.coin_list.draw() # Draw the graphs self.perf_graph_list.draw() # Get FPS for the last 60 frames text = f"FPS: {arcade.get_fps(60):5.1f}" arcade.draw_text(text, 10, 10, arcade.color.BLACK, 22) def update(self, delta_time): """ Update method """ self.frame_count += 1 # Print and clear timings every 60 frames if self.frame_count % 60 == 0: arcade.print_timings() arcade.clear_timings() # Move the coins self.coin_list.update() def main(): """ Main function """ window = MyGame() window.setup() arcade.run() if __name__ == "__main__": main() |