纯文本按钮#
有关图形用户界面系统的介绍,请参见 图形用户界面概念 。
这个 arcade.gui.UIFlatButton
是一个带有文本标签的简单按钮。它没有任何三维外观。
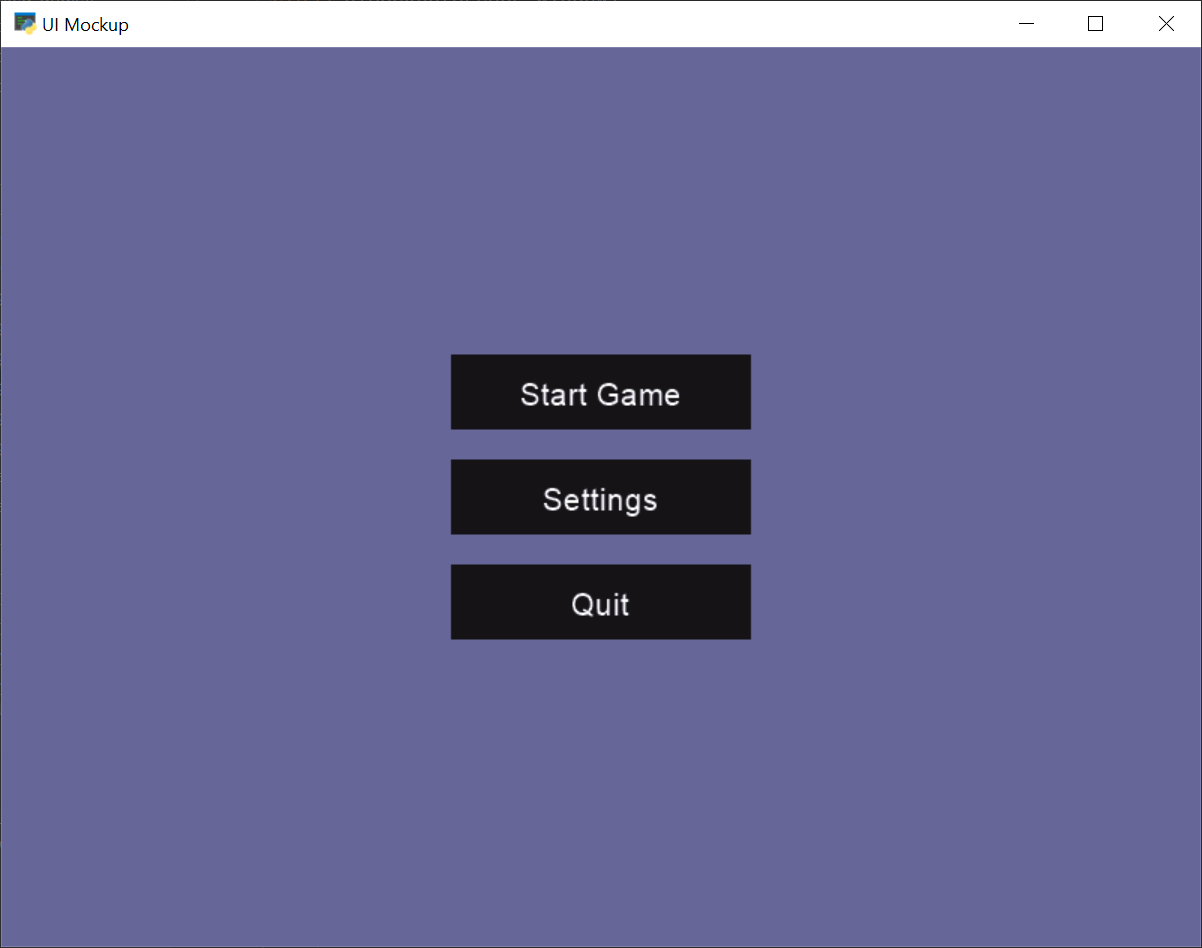
有三种方法可以处理按钮单击事件:
创建一个父类为 arcade.UIFlatButton 并实现一个名为 on_click 。
创建一个按钮,然后将 on_click 属性设置为等于要调用的函数。
创建一个按钮。然后使用修饰符指定在调用 on_click 事件为该按钮发生。
此代码显示上述三种方式中的每一种。代码应该选择三种方法中的一种,并通过程序对其进行标准化。不要编写同时使用这三种方法的代码。
gui_flat_button.py#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | """ Example code showing how to create a button, and the three ways to process button events. """ import arcade import arcade.gui # --- Method 1 for handling click events, # Create a child class. class QuitButton(arcade.gui.UIFlatButton): def on_click(self, event: arcade.gui.UIOnClickEvent): arcade.exit() class MyWindow(arcade.Window): def __init__(self): super().__init__(800, 600, "UIFlatButton Example", resizable=True) # --- Required for all code that uses UI element, # a UIManager to handle the UI. self.manager = arcade.gui.UIManager() self.manager.enable() # Set background color arcade.set_background_color(arcade.color.DARK_BLUE_GRAY) # Create a vertical BoxGroup to align buttons self.v_box = arcade.gui.UIBoxLayout() # Create the buttons start_button = arcade.gui.UIFlatButton(text="Start Game", width=200) self.v_box.add(start_button.with_space_around(bottom=20)) settings_button = arcade.gui.UIFlatButton(text="Settings", width=200) self.v_box.add(settings_button.with_space_around(bottom=20)) # Again, method 1. Use a child class to handle events. quit_button = QuitButton(text="Quit", width=200) self.v_box.add(quit_button) # --- Method 2 for handling click events, # assign self.on_click_start as callback start_button.on_click = self.on_click_start # --- Method 3 for handling click events, # use a decorator to handle on_click events @settings_button.event("on_click") def on_click_settings(event): print("Settings:", event) # Create a widget to hold the v_box widget, that will center the buttons self.manager.add( arcade.gui.UIAnchorWidget( anchor_x="center_x", anchor_y="center_y", child=self.v_box) ) def on_click_start(self, event): print("Start:", event) def on_draw(self): self.clear() self.manager.draw() window = MyWindow() arcade.run() |