Mahotas:用Python语言实现计算机视觉¶
备注
如果您在科学出版物中使用mahotas,请引用:
科埃略,L.P.,2013年。Mahotas:用于可编写脚本的计算机视觉的开源软件。开放研究软件杂志1(1):E3,DOI:https://dx.doi.org/10.5334/jors.ac
Mahotas是一个用于Python的计算机视觉和图像处理库。
它包括许多用C++实现的算法,以提高在Numy数组中操作时的速度,并具有非常干净的Python接口。
Mahotas目前拥有100多个用于图像处理和计算机视觉的功能,而且还在不断增长。Mahotas功能的一些示例:
凸点计算。
泽尼克和哈拉里克, local binary patterns 和TAS功能。
卷积。
Sobel边缘检测。
发布计划大约是每隔几个月发布一个版本,每个版本都会带来新的功能和改进的性能。不过,界面非常稳定,使用多年前的mahotas版本编写的代码在当前版本中运行得很好,只是速度会更快(一些界面已被弃用,几年后将被移除,但在此期间,你只会收到警告)。
带有测试用例的错误报告通常在24小时内得到修复。
参见
mahotas-imread 是一个附带项目,包括读取图像/将图像写入文件的代码
实例¶
这是一个加载文件(名为 test.jpeg )和呼叫 watershed 使用高于阈值的区域作为种子(我们使用最大类间方差来定义阈值)。
import numpy as np
import mahotas
import pylab
img = mahotas.imread('test.jpeg')
T_otsu = mahotas.thresholding.otsu(img)
seeds,_ = mahotas.label(img > T_otsu)
labeled = mahotas.cwatershed(img.max() - img, seeds)
pylab.imshow(labeled)
计算距离变换也很容易:
import pylab as p
import numpy as np
import mahotas
f = np.ones((256,256), bool)
f[200:,240:] = False
f[128:144,32:48] = False
# f is basically True with the exception of two islands: one in the lower-right
# corner, another, middle-left
dmap = mahotas.distance(f)
p.imshow(dmap)
p.show()
(Source code
, png
, hires.png
, pdf
)
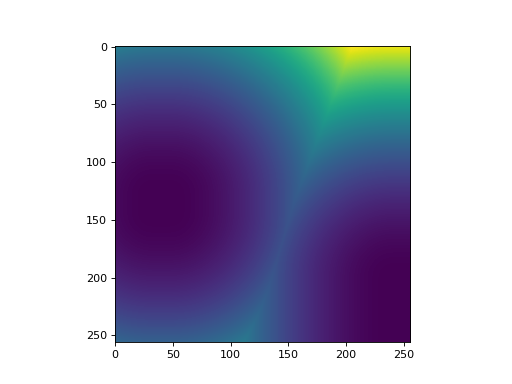
完整的文档内容¶
跳至详细信息 API Documentation
- 如何安装Mahotas
- 寻找沃利
- 带标记的图像函数
- 阈值设置
- 小波变换
- 距离变换
- 多边形实用程序
- 功能
- 局部二进制模式
- 加速的健壮特征
- 用Mahotas实现SURF-REF
- 形态运算符
- 色彩空间转换
- 使用Mahotas的输入/输出
- 教程:使用Mahotas进行分类
- 教程:扩展景深
- Mahotas-features.py
- 常见问题解答
- Mahotas内部结构
- 产生mahotas的原因
- 贡献
- 可能的任务
- 历史
- 版本1.4.13(2022年6月28日)
- 版本1.4.12(2021年10月14日)
- 版本1.4.11(2020年8月16日)
- 版本1.4.10(2020年6月11日)
- 版本1.4.9(2019年11月12日)
- 版本1.4.8(2019年10月11日)
- 版本1.4.7(2019年7月10日)
- 版本1.4.6(2019年7月10日)
- 版本1.4.5(2018年10月20日)
- 版本1.4.4(2017年11月5日)
- 版本1.4.3(2016年10月3日)
- 版本1.4.2(2016年10月2日)
- 版本1.4.1(2015年12月20日)
- 版本1.4.0(2015年7月8日)
- 版本1.3.0(2015年4月28日)
- 版本1.2.4(2014年12月23日)
- 版本1.2.3(2014年11月8日)
- 版本1.2.2(2014年10月19日)
- 版本1.2.1(2014年7月21日)
- 1.2版(2014年7月17日)
- 版本1.1.1(2014年7月4日)
- 1.1.0(2014年2月12日)
- 1.0.4(2013-12-15)
- 1.0.3(2013-10-06)
- 1.0.2(2013年7月10日)
- 1.0.1(2013年7月9日)
- 1.0(2013年5月21日)
- 0.99(2013年5月4日)
- 0.9.8(2013年4月22日)
- 0.9.7(2013年2月3日)
- 0.9.6(2012年12月2日)
- 0.9.5(2012年11月5日)
- 0.9.4(2012年10月10日)
- 0.9.3(2012年10月9日)
- 0.9.2(2012年9月1日)
- 0.9.1(2012年8月28日)
- 0.9(2012年7月16日)
- 0.8.1(2012年6月6日)
- 0.8(2012年5月7日)
- 0.7.3(2012年3月14日)
- 0.7.2(2012年2月13日)
- 0.7.1(2012年1月6日)
- 版本.6.6(2011年8月8日)
- 对于版本 0.6.5
- 完整的API文档
- 主要特征
as_rgb()
bbox()
border()
borders()
bwperim()
cdilate()
center_of_mass()
cerode()
close()
close_holes()
convolve()
convolve1d()
croptobbox()
cwatershed()
daubechies()
dilate()
disk()
distance()
dog()
erode()
euler()
find()
fullhistogram()
gaussian_filter()
gaussian_filter1d()
get_structuring_elem()
haar()
hitmiss()
idaubechies()
ihaar()
imread()
imresize()
imsave()
label()
labeled_sum()
laplacian_2D()
locmax()
locmin()
majority_filter()
mean_filter()
median_filter()
moments()
open()
otsu()
overlay()
rank_filter()
rc()
regmax()
regmin()
sobel()
stretch()
stretch_rgb()
template_match()
thin()
wavelet_center()
wavelet_decenter()
eccentricity()
ellipse_axes()
haralick()
lbp()
pftas()
roundness()
tas()
zernike()
zernike_moments()
rgb2gray()
rgb2grey()
rgb2lab()
rgb2sepia()
rgb2xyz()
xyz2lab()
xyz2rgb()