In [1]: import pandas as pd
In [2]: import matplotlib.pyplot as plt
- Air quality data
在本教程中,空气质量数据关于 \(NO_2\) 由以下人员使用、提供 OpenAQ 并使用 py-openaq 包裹。这个
To raw dataair_quality_no2.csv
数据集提供 \(NO_2\) 测量站的值 FR04014 , BETR801 和 伦敦威斯敏斯特 分别在巴黎、安特卫普和伦敦。In [3]: air_quality = pd.read_csv("data/air_quality_no2.csv", index_col=0, parse_dates=True) In [4]: air_quality.head() Out[4]: station_antwerp station_paris station_london datetime 2019-05-07 02:00:00 NaN NaN 23.0 2019-05-07 03:00:00 50.5 25.0 19.0 2019-05-07 04:00:00 45.0 27.7 19.0 2019-05-07 05:00:00 NaN 50.4 16.0 2019-05-07 06:00:00 NaN 61.9 NaN
备注
的用法
index_col
和parse_dates
的参数read_csv
函数将第一(0)列定义为结果DataFrame
并将列中的日期转换为Timestamp
对象。
如何在Pandas身上创造情节?#
我要对数据进行快速目视检查。
In [5]: air_quality.plot() Out[5]: <AxesSubplot:xlabel='datetime'>
使用一个
DataFrame
,默认情况下,Pandas使用数字数据为每一列创建一条线条图。
我只想用来自巴黎的数据绘制数据表的列。
In [6]: air_quality["station_paris"].plot() Out[6]: <AxesSubplot:xlabel='datetime'>
若要绘制特定列,请使用 subset data tutorial 与
plot()
方法。因此,plot()
方法在两种情况下都有效Series
和DataFrame
。
我想要直观地比较 \(NO_2\) 在伦敦和巴黎测量的价值。
In [7]: air_quality.plot.scatter(x="station_london", y="station_paris", alpha=0.5) Out[7]: <AxesSubplot:xlabel='station_london', ylabel='station_paris'>
除了默认设置之外 line
在使用时绘制 plot
函数时,可以使用多种替代方法来绘制数据。让我们使用一些标准的Python来概述可用的Plot方法:
In [8]: [
...: method_name
...: for method_name in dir(air_quality.plot)
...: if not method_name.startswith("_")
...: ]
...:
Out[8]:
['area',
'bar',
'barh',
'box',
'density',
'hexbin',
'hist',
'kde',
'line',
'pie',
'scatter']
备注
在许多开发环境以及IPython和Jupyter Notebook中,使用TAB按钮可以获得可用方法的概述,例如 air_quality.plot.
+Tab键。
其中一个选项是 DataFrame.plot.box()
,它指的是 boxplot 。这个 box
方法适用于空气质量实例数据:
In [9]: air_quality.plot.box()
Out[9]: <AxesSubplot:>
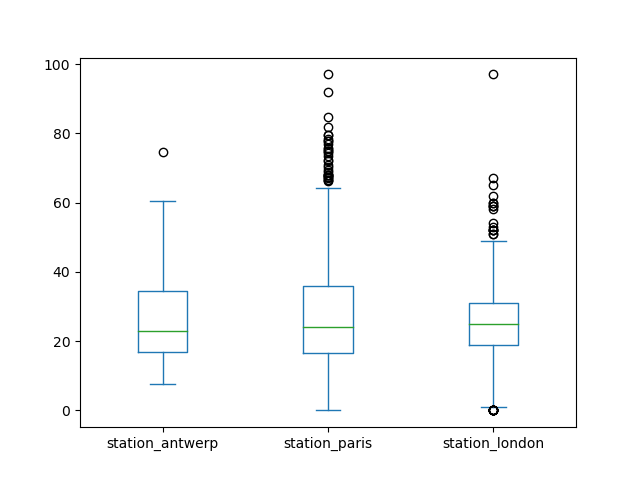
有关默认折线图以外的绘图的介绍,请参阅《用户指南》部分关于 supported plot styles 。
我希望每一列都在一个单独的小情节中。
In [10]: axs = air_quality.plot.area(figsize=(12, 4), subplots=True)
支持每个数据列的单独子图
subplots
的参数plot
功能。每个Pandas绘图功能中提供的内置选项都值得一看。
上的用户指南部分介绍了一些更多的格式选项 plot formatting 。
我想进一步自定义、扩展或保存生成的绘图。
In [11]: fig, axs = plt.subplots(figsize=(12, 4)) In [12]: air_quality.plot.area(ax=axs) Out[12]: <AxesSubplot:xlabel='datetime'> In [13]: axs.set_ylabel("NO$_2$ concentration") Out[13]: Text(0, 0.5, 'NO$_2$ concentration') In [14]: fig.savefig("no2_concentrations.png")
Pandas创建的每个地块对象都是 Matplotlib 对象。由于Matplotlib提供了大量的选项来定制绘图,因此使Pandas和Matplotlib之间的链接显式可使Matplotlib能够将所有功能用于绘图。在前面的示例中应用了此策略:
fig, axs = plt.subplots(figsize=(12, 4)) # Create an empty Matplotlib Figure and Axes
air_quality.plot.area(ax=axs) # Use pandas to put the area plot on the prepared Figure/Axes
axs.set_ylabel("NO$_2$ concentration") # Do any Matplotlib customization you like
fig.savefig("no2_concentrations.png") # Save the Figure/Axes using the existing Matplotlib method.
REMEMBER
这个
.plot.*
方法适用于Series和DataFrame。默认情况下,每一列都绘制为不同的元素(Line、BoxPlot、…)。
Pandas创建的任何地块都是Matplotlib对象。
有关在Pandas中绘制地图的完整概述,请参阅 visualization pages 。