우선,텍스트가아닌,도형을시각화하겠다.HP바는어떤가?만약HP의최대값이고정되어있고오직현재HP값만이변한다면,이두데이터를출력하는가장쉬운방법은무엇인가?
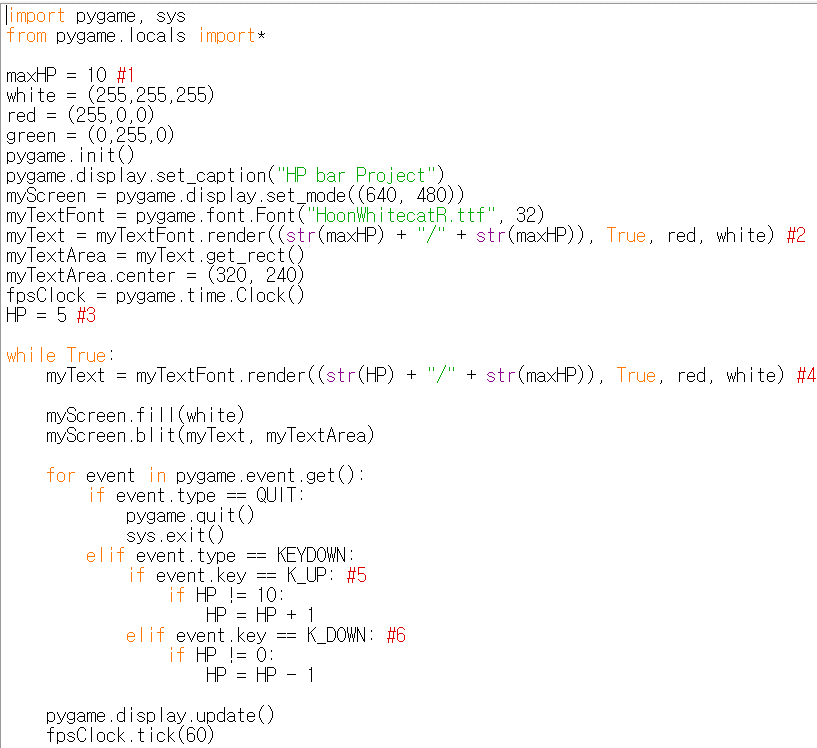
1import sys, pygame
2pygame.init()
3
4size = width, height = 320, 240
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("AdvancedOutputProcess1.gif")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
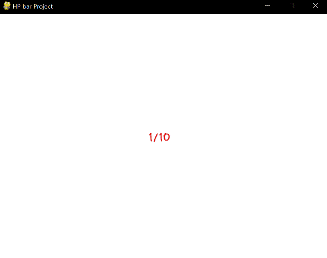
1import sys, pygame
2pygame.init()
3
4size = width, height = 320, 240
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("AdvancedOutputProcess2.gif")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
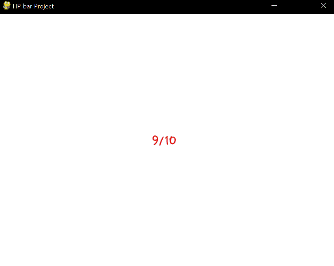
1import sys, pygame
2pygame.init()
3
4size = width, height = 320, 240
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("AdvancedOutputProcess3.gif")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
변수의값이변할때마다텍스트를다시렌더링하기만하면된다.변수의값은어떻게바꾸는가?그것은Event문에서이루어진다。(키보드위또는아래를눌러HP를조절하게하였다。)이전과동일한방법이다.하지만,이것들은여전히텍스트이다.아직충분히시각화되지않는다.이데이터들을어떻게더상세하게시각화할까?총탄창에서아이디어를따올수있다.HP는정수값이고,불연속적값을가지므로,아래와같이출력될수있다。
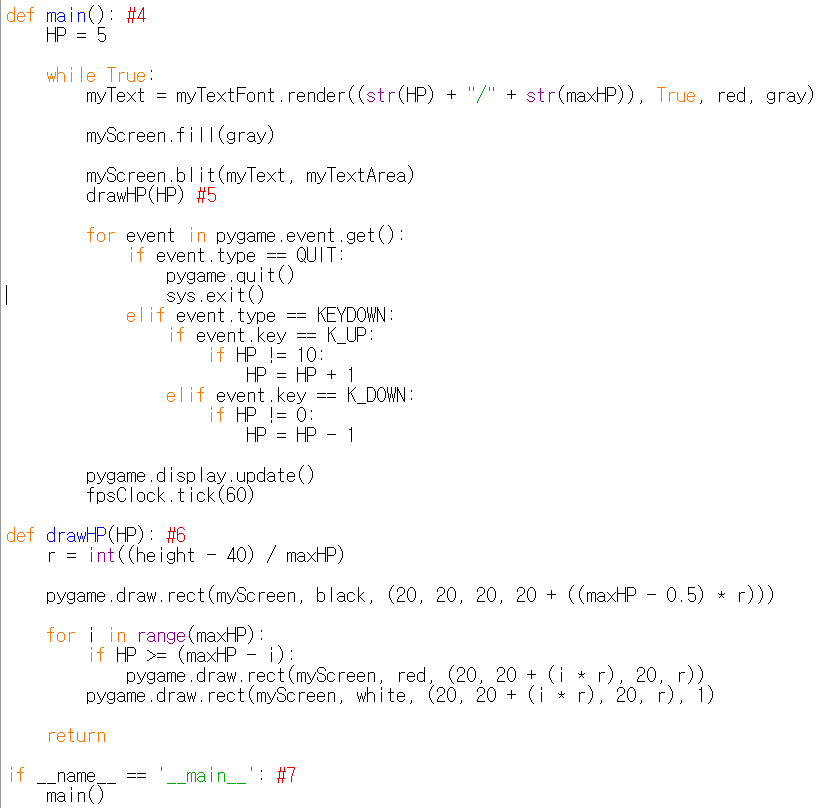
1import sys, pygame
2pygame.init()
3
4size = width, height = 320, 240
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("AdvancedOutputProcess4.gif")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
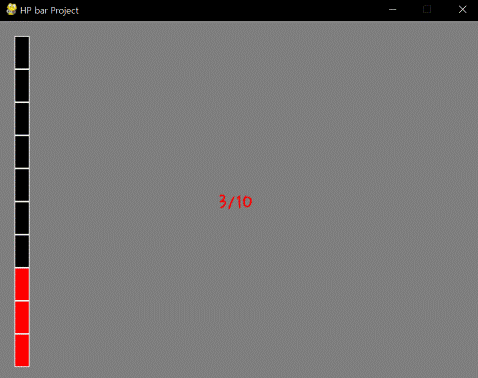
1import sys, pygame
2pygame.init()
3
4size = width, height = 320, 240
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("AdvancedOutputProcess5.gif")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
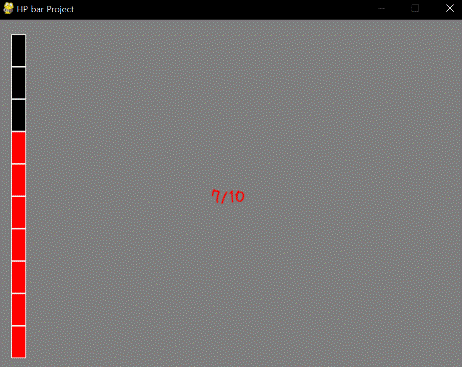
1import sys, pygame
2pygame.init()
3
4size = width, height = 320, 240
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("AdvancedOutputProcess6.gif")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
훨씬나아졌다.그리는로직은#6을확인하면된다。로직은단순하다.먼저,큰검은색직사각형을그린다.그다음,현재HP을따져서작은빨간색직사각형들을0개또는최대개수만큼그린다。마지막으로,작은직사각형들에하얀테두리를그린다.Pygame.Draw.rect함수에선위치변수로4개의매개변수가사용된것을확인할수있는데(첫번째변수는캔버스변수이고,두번째변수는색상변수,네번째변수는두께변수이다。)이4개의매개변수의용도가무엇인지는직접설명하는것보다,직접값을변경하면서확인하는것이가장쉽게이해할수있다.3개의값이20일때하나의값만을10또는30으로바꿔보아라!
그리고,이제는본격적으로함수화를해야한다.Always문과Event문을Main함수에담았는데,이경우Main함수를프로그램이찾을수있도록#7에서추가적인처리를해야한다。그다음Drag HP라는새로운함수를만들었다。게임에서의함수화아이디어는图形用户界面프로그램을만들때의함수화아이디어와크게다르지않다。예를들면,하나의변수를출력하는하나의출력함수를각각만들어두는것이좋을것이다.물론,각각의변수가출력될좌표를정하는것은화면전체를디자인할때선행되어야할것이다.
<참고코드>::
import pygame, sys
from pygame.locals import*
maxHP = 10
white = (255,255,255)
gray = (127,127,127)
black = (0,0,0)
red = (255,0,0)
green = (0,255,0)
blue = (0,0,255)
pygame.init()
pygame.display.set_caption("HP bar Project")
width = 640 #1
height = 480 #2
myScreen = pygame.display.set_mode((width, height))
myTextFont = pygame.font.Font("HoonWhitecatR.ttf", 32)
myText = myTextFont.render((str(maxHP) + "/" + str(maxHP)), True, red, gray)
myTextArea = myText.get_rect()
myTextArea.center = (width/2, height/2) #3
fpsClock = pygame.time.Clock()
def main(): #4
HP = 5
while True:
myText = myTextFont.render((str(HP) + "/" + str(maxHP)), True, red, gray)
myScreen.fill(gray)
myScreen.blit(myText, myTextArea)
drawHP(HP) #5
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == KEYDOWN:
if event.key == K_UP:
if HP != 10:
HP = HP + 1
elif event.key == K_DOWN:
if HP != 0:
HP = HP - 1
pygame.display.update()
fpsClock.tick(60)
def drawHP(HP): #6
r = int((height - 40) / maxHP)
pygame.draw.rect(myScreen, black, (20, 20, 20, 20 + ((maxHP - 0.5) * r)))
for i in range(maxHP):
if HP >= (maxHP - i):
pygame.draw.rect(myScreen, red, (20, 20 + (i * r), 20, r))
pygame.draw.rect(myScreen, white, (20, 20 + (i * r), 20, r), 1)
return
if __name__ == '__main__': #7
main()
Edit on GitHub