通常,我们首先学习如何输出东西(想想Hello World!),学习如何输入东西总是第二位的。为什么?因为输入不是某些程序的要求,而不是输出,所以每个程序都需要输入。(这就是程序的定义。大于或等于0输入,大于或等于1输出。)然而,每一场比赛都需要输入。这就是为什么我们说“我喜欢玩游戏”。玩耍意味着移动你身体的一部分(可能是你的手指)。无论如何,让我们添加输入逻辑,使这个项目成为真正的游戏。
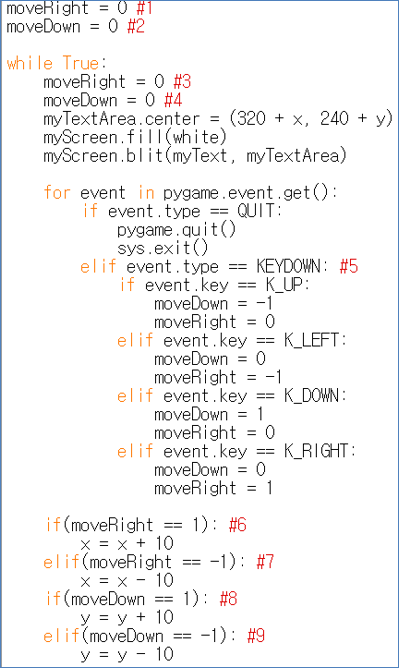
1import sys, pygame
2pygame.init()
3
4size = width, height = 220, 140
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("Bagic-INPUT-sourcecode.png")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
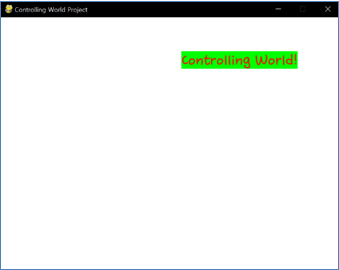
1import sys, pygame
2pygame.init()
3
4size = width, height = 220, 140
5speed = [2, 2]
6black = 0, 0, 0
7
8screen = pygame.display.set_mode(size)
9
10ball = pygame.image.load("Bagic-INPUT-resultscreen.png")
11ballrect = ball.get_rect()
12
13while True:
14 for event in pygame.event.get():
15 if event.type == pygame.QUIT: sys.exit()
16
17 ballrect = ballrect.move(speed)
18 if ballrect.left < 0 or ballrect.right > width:
19 speed[0] = -speed[0]
20 if ballrect.top < 0 or ballrect.bottom > height:
21 speed[1] = -speed[1]
22
23 screen.fill(black)
24 screen.blit(ball, ballrect)
25 pygame.display.flip()
(控制World Project的源代码及其结果屏幕)
(不是控制World Project的全部源代码,而是部分)
(控制世界!当玩家按下键盘的四个方向键中的一个时移动)
There are 2 big difference in comparison to before project. First big difference is line #5, which adds checking KEYDOWN
event is triggered or not. Other lines are just changing previous algorithm to act differently. We know that same command can make big difference in entire program when it is executed before Event statement of after Event statement. Pay attention that process about changing location appear after Event statement. (Update after set. That is second big difference). Variable event.key
means latest pressed key on keyboard. Look at the specific key name. K_UP, K_LEFT, K_DOWN, K_RIGHT. Very intuitive K_ series. (Given by pygame.locals which we added at the Header) Furthermore, there are other key named K_8, K_a, K_L, K_LCTRL, K_DELETE, or K_F4. We can understand meaning of these keys without extra explanation. Full key list can be found in
https://www.pygame.org/docs/ref/key.html#pygame.key.name.
注意,KEYDOWN的意思是“这个键以前没有按过,但是 现在是按下的 “和”的涵义 “暂挂”不包括在内 这里。在保持的情况下,关于检查的新事件处理 KEYUP
(意思是以前按了这个键,现在没有按)需要一些处理(需要额外的变量和算法)。这将在高级部分提到。
添加输入很容易,因为它只是添加带有特定事件参数的if阶段。现在游戏项目完成了,因为项目有输出,有过程,有输入!真的?不是的。这个项目不能被称为游戏,因为没有 互动 至少在两个人之间 游戏对象 ,否 rule 对于播放此游戏(既不限制(例如惠普,时间)也没有得分)。大多数情况下,不是 赏心悦目 (没有动机,没有各种各样的输入和输出,没有吸引人的内容)首先,我们必须学习更高级的输入(例如,鼠标状态的处理)、过程(例如,功能化的想法)和输出(例如,打印图像/声音或可视化内部数据),以繁荣游戏界面/系统。不要停留在打印常量文本或输入单键的级别。当然,这种水平的经验对高级水平肯定是有帮助的。那么,让我们进入更高的层次吧!
<参考代码>::
import pygame, sys
from pygame.locals import*
white = (255,255,255)
red = (255,0,0)
green = (0,255,0)
pygame.init()
pygame.display.set_caption("Controlling World Project")
myScreen = pygame.display.set_mode((640, 480))
myTextFont = pygame.font.Font("HoonWhitecatR.ttf", 32)
myText = myTextFont.render("Controlling World!", True, red, green)
myTextArea = myText.get_rect()
myTextArea.center = (320, 240)
fpsClock = pygame.time.Clock()
x = 0
y = 0
moveRight = 0 #1
moveDown = 0 #2
while True:
moveRight = 0 #3
moveDown = 0 #4
myTextArea.center = (320 + x, 240 + y)
myScreen.fill(white)
myScreen.blit(myText, myTextArea)
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == KEYDOWN: #5
if event.key == K_UP:
moveDown = -1
moveRight = 0
elif event.key == K_LEFT:
moveDown = 0
moveRight = -1
elif event.key == K_DOWN:
moveDown = 1
moveRight = 0
elif event.key == K_RIGHT:
moveDown = 0
moveRight = 1
if(moveRight == 1): #6
x = x + 10
elif(moveRight == -1): #7
x = x - 10
if(moveDown == 1): #8
y = y + 10
elif(moveDown == -1): #9
y = y - 10
pygame.display.update()
Edit on GitHub