注解
Click here 下载完整的示例代码
闪电教程¶
“BLIT”是一个 standard technique 在栅格图形中,在Matplotlib的上下文中,可以用来(极大地)提高交互式图形的性能。例如 animation
和 widgets
模块内部使用blitting。在这里,我们将演示如何在这些类之外实现您自己的blitting。
绩效提升的根源就是不必重新做我们不需要做的工作。如果轴的限制没有改变,那么就不需要重新绘制所有的记号和记号标签(特别是因为文本是渲染成本较高的对象之一)。
保存我们工作的程序大致如下:
- 绘制图形,但不包括任何标记为“动画”的艺术家
- 保存RBGA缓冲区的副本
在未来,为了更新“动画”艺术家,我们
- 还原RGBA缓冲区的副本
- 只重绘动画艺术家
- 在屏幕上显示结果图像
从而使我们不必重新绘制所有 _not_ 有生气的。此过程的一个结果是,动画艺术家总是以比静态艺术家更高的z顺序绘制。
不是所有的后端都支持blitting。您可以通过 FigureCanvasBase.supports_blit
财产。
警告
此代码不适用于OSX后端(但适用于mac上的其他GUI后端)。
最小示例¶
我们可以使用 FigureCanvasAgg
方法 copy_from_bbox
和 restore_region
结合设置 animated=True
在我们的艺术家实现一个最小的例子,使用光点加速渲染
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 2 * np.pi, 100)
fig, ax = plt.subplots()
# animated=True tells matplotlib to only draw the artist when we
# explicitly request it
(ln,) = ax.plot(x, np.sin(x), animated=True)
# make sure the window is raised, but the script keeps going
plt.show(block=False)
# stop to admire our empty window axes and ensure it is rendered at
# least once.
#
# We need to fully draw the figure at its final size on the screen
# before we continue on so that :
# a) we have the correctly sized and drawn background to grab
# b) we have a cached renderer so that ``ax.draw_artist`` works
# so we spin the event loop to let the backend process any pending operations
plt.pause(0.1)
# get copy of entire figure (everything inside fig.bbox) sans animated artist
bg = fig.canvas.copy_from_bbox(fig.bbox)
# draw the animated artist, this uses a cached renderer
ax.draw_artist(ln)
# show the result to the screen, this pushes the updated RGBA buffer from the
# renderer to the GUI framework so you can see it
fig.canvas.blit(fig.bbox)
for j in range(100):
# reset the background back in the canvas state, screen unchanged
fig.canvas.restore_region(bg)
# update the artist, neither the canvas state nor the screen have changed
ln.set_ydata(np.sin(x + (j / 100) * np.pi))
# re-render the artist, updating the canvas state, but not the screen
ax.draw_artist(ln)
# copy the image to the GUI state, but screen might not changed yet
fig.canvas.blit(fig.bbox)
# flush any pending GUI events, re-painting the screen if needed
fig.canvas.flush_events()
# you can put a pause in if you want to slow things down
# plt.pause(.1)
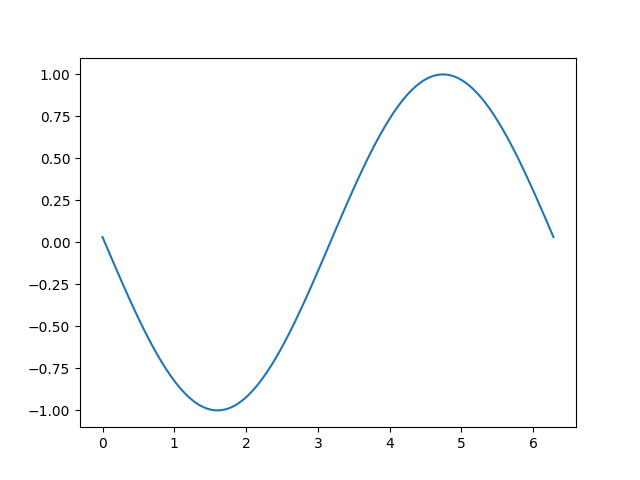
这个例子工作并显示了一个简单的动画,但是,因为我们只抓取一次背景,如果图形的大小(以像素为单位)发生变化(由于图形的大小或dpi发生变化),背景将无效并导致不正确(但有时看起来很酷!)图像。在这个类中,我们建议有一个变量。
基于类的示例¶
我们可以使用一个类来封装样板逻辑和恢复背景的状态,绘制艺术家,然后将结果显示在屏幕上。另外,我们可以使用 'draw_event'
回调以在完全重新绘制时捕获新背景,以正确处理大小调整。
class BlitManager:
def __init__(self, canvas, animated_artists=()):
"""
Parameters
----------
canvas : FigureCanvasAgg
The canvas to work with, this only works for sub-classes of the Agg
canvas which have the `~FigureCanvasAgg.copy_from_bbox` and
`~FigureCanvasAgg.restore_region` methods.
animated_artists : Iterable[Artist]
List of the artists to manage
"""
self.canvas = canvas
self._bg = None
self._artists = []
for a in animated_artists:
self.add_artist(a)
# grab the background on every draw
self.cid = canvas.mpl_connect("draw_event", self.on_draw)
def on_draw(self, event):
"""Callback to register with 'draw_event'."""
cv = self.canvas
if event is not None:
if event.canvas != cv:
raise RuntimeError
self._bg = cv.copy_from_bbox(cv.figure.bbox)
self._draw_animated()
def add_artist(self, art):
"""
Add an artist to be managed.
Parameters
----------
art : Artist
The artist to be added. Will be set to 'animated' (just
to be safe). *art* must be in the figure associated with
the canvas this class is managing.
"""
if art.figure != self.canvas.figure:
raise RuntimeError
art.set_animated(True)
self._artists.append(art)
def _draw_animated(self):
"""Draw all of the animated artists."""
fig = self.canvas.figure
for a in self._artists:
fig.draw_artist(a)
def update(self):
"""Update the screen with animated artists."""
cv = self.canvas
fig = cv.figure
# paranoia in case we missed the draw event,
if self._bg is None:
self.on_draw(None)
else:
# restore the background
cv.restore_region(self._bg)
# draw all of the animated artists
self._draw_animated()
# update the GUI state
cv.blit(fig.bbox)
# let the GUI event loop process anything it has to do
cv.flush_events()
下面是我们如何使用我们的课堂。这是一个比第一种情况稍微复杂的例子,因为我们还添加了一个文本框计数器。
# make a new figure
fig, ax = plt.subplots()
# add a line
(ln,) = ax.plot(x, np.sin(x), animated=True)
# add a frame number
fr_number = ax.annotate(
"0",
(0, 1),
xycoords="axes fraction",
xytext=(10, -10),
textcoords="offset points",
ha="left",
va="top",
animated=True,
)
bm = BlitManager(fig.canvas, [ln, fr_number])
# make sure our window is on the screen and drawn
plt.show(block=False)
plt.pause(.1)
for j in range(100):
# update the artists
ln.set_ydata(np.sin(x + (j / 100) * np.pi))
fr_number.set_text("frame: {j}".format(j=j))
# tell the blitting manager to do it's thing
bm.update()
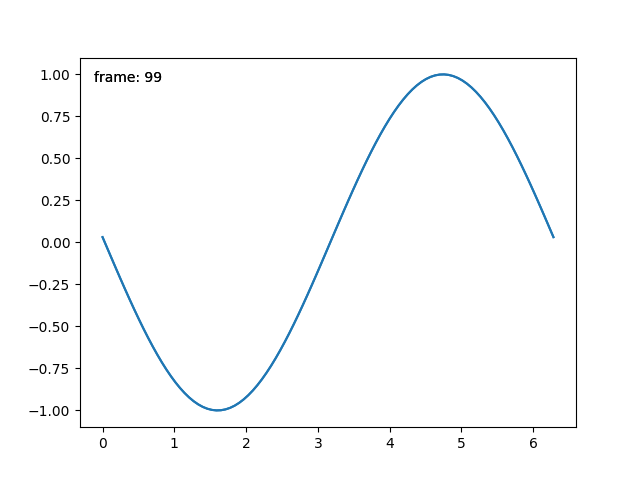
该类不依赖于 pyplot
适合嵌入到更大的GUI应用程序中。
脚本的总运行时间: (0分1.285秒)
关键词:matplotlib代码示例,codex,python plot,pyplot Gallery generated by Sphinx-Gallery