注解
Click here 下载完整的示例代码
使用矩形和多集合构建柱状图¶
使用路径修补程序绘制矩形。在MPL中使用moveto/lineto、closepoly等适当路径之前,实现了使用大量矩形实例的技术,或使用多集合的更快方法。现在我们有了它们,就可以用PathCollection更有效地绘制具有同构属性的规则形状对象的集合。这个例子制作了一个柱状图——在开始时设置顶点数组的工作更多,但是对于大量的对象来说应该更快。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import matplotlib.path as path
fig, ax = plt.subplots()
# Fixing random state for reproducibility
np.random.seed(19680801)
# histogram our data with numpy
data = np.random.randn(1000)
n, bins = np.histogram(data, 50)
# get the corners of the rectangles for the histogram
left = bins[:-1]
right = bins[1:]
bottom = np.zeros(len(left))
top = bottom + n
# we need a (numrects x numsides x 2) numpy array for the path helper
# function to build a compound path
XY = np.array([[left, left, right, right], [bottom, top, top, bottom]]).T
# get the Path object
barpath = path.Path.make_compound_path_from_polys(XY)
# make a patch out of it
patch = patches.PathPatch(barpath)
ax.add_patch(patch)
# update the view limits
ax.set_xlim(left[0], right[-1])
ax.set_ylim(bottom.min(), top.max())
plt.show()
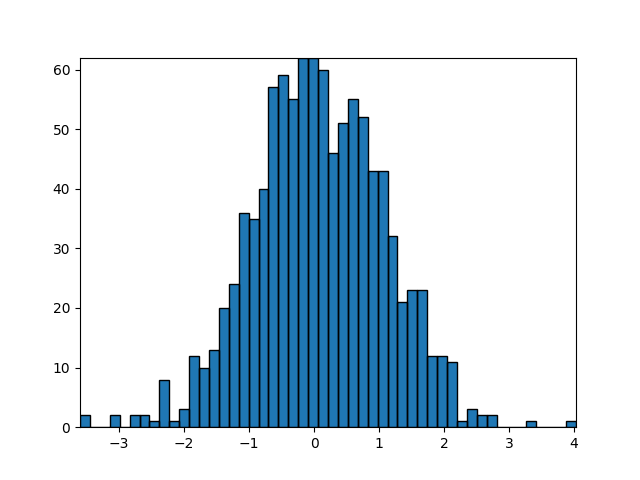
应该注意的是,不要创建三维数组,而是使用 make_compound_path_from_polys
我们还可以直接使用顶点和代码创建复合路径,如下所示
nrects = len(left)
nverts = nrects*(1+3+1)
verts = np.zeros((nverts, 2))
codes = np.ones(nverts, int) * path.Path.LINETO
codes[0::5] = path.Path.MOVETO
codes[4::5] = path.Path.CLOSEPOLY
verts[0::5, 0] = left
verts[0::5, 1] = bottom
verts[1::5, 0] = left
verts[1::5, 1] = top
verts[2::5, 0] = right
verts[2::5, 1] = top
verts[3::5, 0] = right
verts[3::5, 1] = bottom
barpath = path.Path(verts, codes)
工具书类¶
以下函数、方法、类和模块的使用如本例所示:
import matplotlib
matplotlib.patches
matplotlib.patches.PathPatch
matplotlib.path
matplotlib.path.Path
matplotlib.path.Path.make_compound_path_from_polys
matplotlib.axes.Axes.add_patch
matplotlib.collections.PathCollection
# This example shows an alternative to
matplotlib.collections.PolyCollection
matplotlib.axes.Axes.hist
出:
<function Axes.hist at 0x7faa00dc7f28>
关键词:matplotlib代码示例,codex,python plot,pyplot Gallery generated by Sphinx-Gallery