注解
Click here 下载完整的示例代码
彩色网格¶
axes.Axes.pcolormesh
允许您生成二维图像样式打印。请注意,它比类似的 pcolor
.
import matplotlib
import matplotlib.pyplot as plt
from matplotlib.colors import BoundaryNorm
from matplotlib.ticker import MaxNLocator
import numpy as np
基本颜色¶
我们通常通过定义四边形的边和四边形的值来指定pcolormesh。注意这里 x 和 y 在各自的维度中,每个元素都比Z多出一个元素。
np.random.seed(19680801)
Z = np.random.rand(6, 10)
x = np.arange(-0.5, 10, 1) # len = 11
y = np.arange(4.5, 11, 1) # len = 7
fig, ax = plt.subplots()
ax.pcolormesh(x, y, Z)
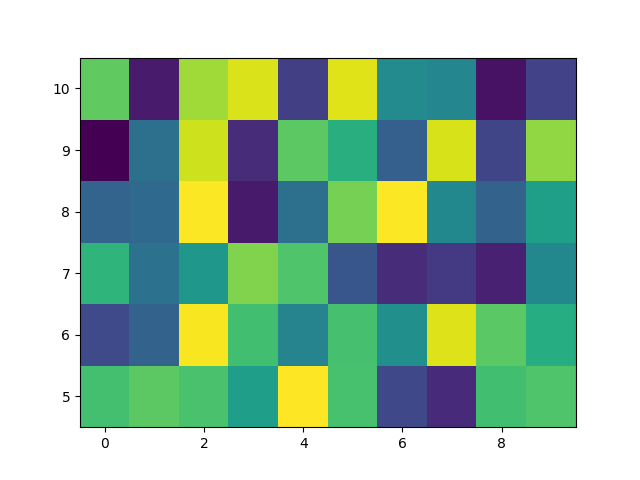
出:
<matplotlib.collections.QuadMesh object at 0x7fa9fc8e17b8>
居中坐标¶
通常用户想要通过 X 和 Y 尺寸与 Z 到 axes.Axes.pcolormesh
. 这也是允许的,如果 shading='auto'
已通过(默认设置为 rcParams["pcolor.shading"]
(default: 'flat'
) ). 前Matplotlib 3.3, shading='flat'
将删除 Z ;虽然出于向后兼容性的目的仍然允许这样做,但会引发一个DeprecationWarning。
x = np.arange(10) # len = 10
y = np.arange(6) # len = 6
X, Y = np.meshgrid(x, y)
fig, axs = plt.subplots(2, 1, sharex=True, sharey=True)
axs[0].pcolormesh(X, Y, Z, vmin=np.min(Z), vmax=np.max(Z), shading='auto')
axs[0].set_title("shading='auto' = 'nearest'")
axs[1].pcolormesh(X, Y, Z, vmin=np.min(Z), vmax=np.max(Z), shading='flat')
axs[1].set_title("shading='flat'")
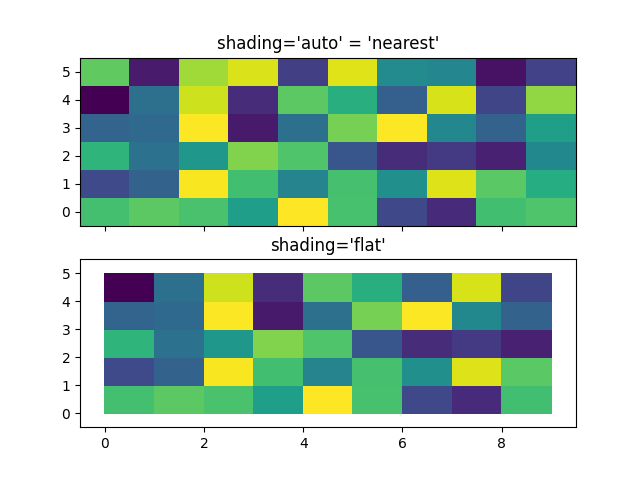
出:
/pb1/rst_repos/git/matplotlib/examples/images_contours_and_fields/pcolormesh_levels.py:67: MatplotlibDeprecationWarning: shading='flat' when X and Y have the same dimensions as C is deprecated since 3.3. Either specify the corners of the quadrilaterals with X and Y, or pass shading='auto', 'nearest' or 'gouraud', or set rcParams['pcolor.shading']. This will become an error two minor releases later.
axs[1].pcolormesh(X, Y, Z, vmin=np.min(Z), vmax=np.max(Z), shading='flat')
Text(0.5, 1.0, "shading='flat'")
使用规范制定等级¶
演示如何将规范化实例和颜色映射实例结合起来绘制 axes.Axes.pcolor
, axes.Axes.pcolormesh
和 axes.Axes.imshow
以类似于轮廓/轮廓的levels关键字参数的方式键入绘图。
# make these smaller to increase the resolution
dx, dy = 0.05, 0.05
# generate 2 2d grids for the x & y bounds
y, x = np.mgrid[slice(1, 5 + dy, dy),
slice(1, 5 + dx, dx)]
z = np.sin(x)**10 + np.cos(10 + y*x) * np.cos(x)
# x and y are bounds, so z should be the value *inside* those bounds.
# Therefore, remove the last value from the z array.
z = z[:-1, :-1]
levels = MaxNLocator(nbins=15).tick_values(z.min(), z.max())
# pick the desired colormap, sensible levels, and define a normalization
# instance which takes data values and translates those into levels.
cmap = plt.get_cmap('PiYG')
norm = BoundaryNorm(levels, ncolors=cmap.N, clip=True)
fig, (ax0, ax1) = plt.subplots(nrows=2)
im = ax0.pcolormesh(x, y, z, cmap=cmap, norm=norm)
fig.colorbar(im, ax=ax0)
ax0.set_title('pcolormesh with levels')
# contours are *point* based plots, so convert our bound into point
# centers
cf = ax1.contourf(x[:-1, :-1] + dx/2.,
y[:-1, :-1] + dy/2., z, levels=levels,
cmap=cmap)
fig.colorbar(cf, ax=ax1)
ax1.set_title('contourf with levels')
# adjust spacing between subplots so `ax1` title and `ax0` tick labels
# don't overlap
fig.tight_layout()
plt.show()
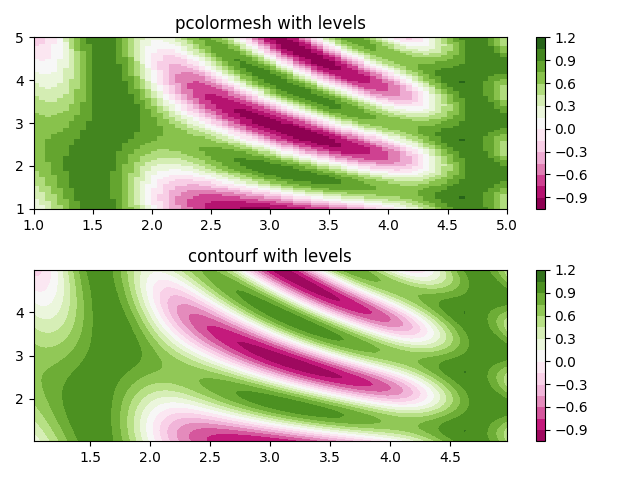
工具书类¶
本例中显示了以下函数和方法的使用:
脚本的总运行时间: (0分1.441秒)
关键词:matplotlib代码示例,codex,python plot,pyplot Gallery generated by Sphinx-Gallery