项目创建¶
DPG可以分解为 项目 , 用户界面项目 , 货柜 。
项目¶
在DPG中创建的所有内容都是 item 。可以通过调用各种 add_* ** * or * draw_** **功能。这些命令返回一个唯一的标识符,可用于以后引用该项目。* 用户界面项目 和 集装箱 也是 项目 ** - but not every ** 项目 必然是一种 用户界面项目 ** or ** 容器**。
所有项目都有以下可选参数: 标签 , tag , user_data ,以及 use_internal_label 。这个 tag 是自动生成的,也可以指定。一个 标签 用作项的显示名称。 user_data 可以是任何值,并且经常用于 回调 。
备注
事件 处理程序 , 注册处 , 组 ,以及 主题 也是物品。这些都是用于定制应用程序的功能、流程和整体外观的底层项目。
货柜¶
集装箱 可以包含其他(允许)项目的项目。
除了通过调用相应的 *add_* ** *函数,也可以通过调用相应的上下文管理器来创建它们。
备注
容器在用作上下文管理器时更有用(也是推荐的)。
下面是创建两个新的 窗户 项使用其上下文管理器并启动应用程序:
import dearpygui.dearpygui as dpg
dpg.create_context()
with dpg.window(label="Window1", pos=(0,0)):
pass
with dpg.window(label="Window2", pos=(100,0)):
pass
dpg.create_viewport(title='Custom Title', width=600, height=200)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()
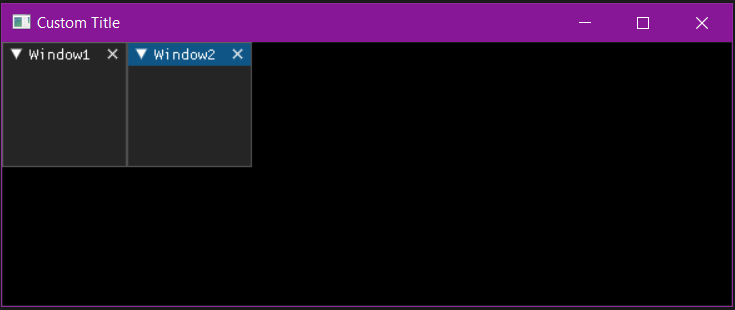
用户界面项目¶
用户界面项目 是被认为是用户界面中的可视且通常可交互的元素的项。
这些措施包括 纽扣 , 滑块 , 输入 ,甚至其他容器,如 窗口 和 树节点 。
下面是一个创建 窗户 包含其他几个项目的容器:
import dearpygui.dearpygui as dpg
dpg.create_context()
with dpg.window(label="Tutorial") as window:
# When creating items within the scope of the context
# manager, they are automatically "parented" by the
# container created in the initial call. So, "window"
# will be the parent for all of these items.
button1 = dpg.add_button(label="Press Me!")
slider_int = dpg.add_slider_int(label="Slide to the left!", width=100)
slider_float = dpg.add_slider_float(label="Slide to the right!", width=100)
# An item's unique identifier (tag) is returned when
# creating items.
print(f"Printing item tag's: {window}, {button1}, {slider_int}, {slider_float}")
# If you want to add an item to an existing container, you
# can specify it by passing the container's tag as the
# "parent" parameter.
button2 = dpg.add_button(label="Don't forget me!", parent=window)
dpg.create_viewport(title='Custom Title', width=600, height=200)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()
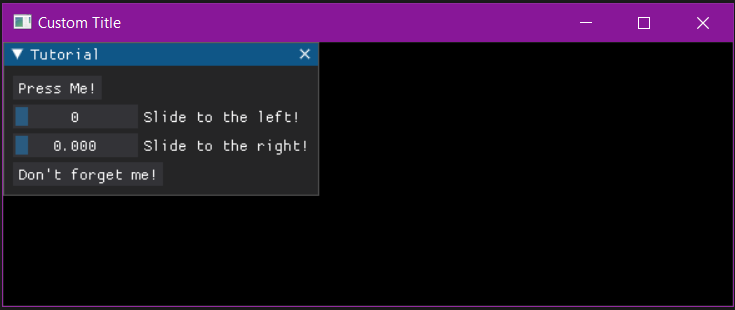
运行时添加和删除¶
使用DPG,您可以在运行时动态添加、删除和移动项目。
这可以通过使用回调来运行所需项的 *add_* ** *命令,并指定该项目将属于的父项。
通过使用 在此之前 关键字添加项时,您可以控制新项将出现在父项之前。默认情况下,会将新小部件放在末尾。
下面是一个演示在运行时添加和删除项目的示例:
import dearpygui.dearpygui as dpg
dpg.create_context()
def add_buttons():
global new_button1, new_button2
new_button1 = dpg.add_button(label="New Button", before="delete_button", tag="new_button1")
new_button2 = dpg.add_button(label="New Button 2", parent="secondary_window", tag="new_button2")
def delete_buttons():
dpg.delete_item("new_button1")
dpg.delete_item("new_button2")
with dpg.window(label="Tutorial", pos=(200, 200)):
dpg.add_button(label="Add Buttons", callback=add_buttons)
dpg.add_button(label="Delete Buttons", callback=delete_buttons, tag="delete_button")
with dpg.window(label="Secondary Window", tag="secondary_window", pos=(100, 100)):
pass
dpg.create_viewport(title='Custom Title', width=600, height=400)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()
提示
删除容器时,默认情况下会删除该容器及其子项,除非关键字 children_only 设置为True,即:
import dearpygui.dearpygui as dpg
dpg.create_context()
def delete_children():
dpg.delete_item("window", children_only=True)
with dpg.window(label="Tutorial", pos=(200, 200), tag="window"):
dpg.add_button(label="Delete Children", callback=delete_children)
dpg.add_button(label="Button_1")
dpg.add_button(label="Button_2")
dpg.add_button(label="Button_3")
dpg.create_viewport(title='Custom Title', width=600, height=400)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()