IO、处理程序、州轮询¶
处理程序¶
处理程序是在项的指定状态更改时提交回调的项。
处理程序可以通过显示或隐藏来激活或停用。
需要将处理程序添加到处理程序注册表。
项目处理程序¶
项处理程序侦听与特定项相关的状态。
- 活动:
已激活
活动的
已点击
停用
编辑后停用
焦点
悬停
调整尺寸
已切换
可见
项处理程序需要添加到项处理程序注册表中。
项处理程序注册表可以绑定到项。它们可以绑定到多个项,以避免必须为每个项复制处理程序。
import dearpygui.dearpygui as dpg
dpg.create_context()
def change_text(sender, app_data):
dpg.set_value("text item", f"Mouse Button ID: {app_data}")
def visible_call(sender, app_data):
print("I'm visible")
with dpg.item_handler_registry(tag="widget handler") as handler:
dpg.add_item_clicked_handler(callback=change_text)
dpg.add_item_visible_handler(callback=visible_call)
with dpg.window(width=500, height=300):
dpg.add_text("Click me with any mouse button", tag="text item")
dpg.add_text("Close window with arrow to change visible state printing to console", tag="text item 2")
# bind item handler registry to item
dpg.bind_item_handler_registry("text item", "widget handler")
dpg.bind_item_handler_registry("text item 2", "widget handler")
dpg.create_viewport(title='Custom Title', width=800, height=600)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()
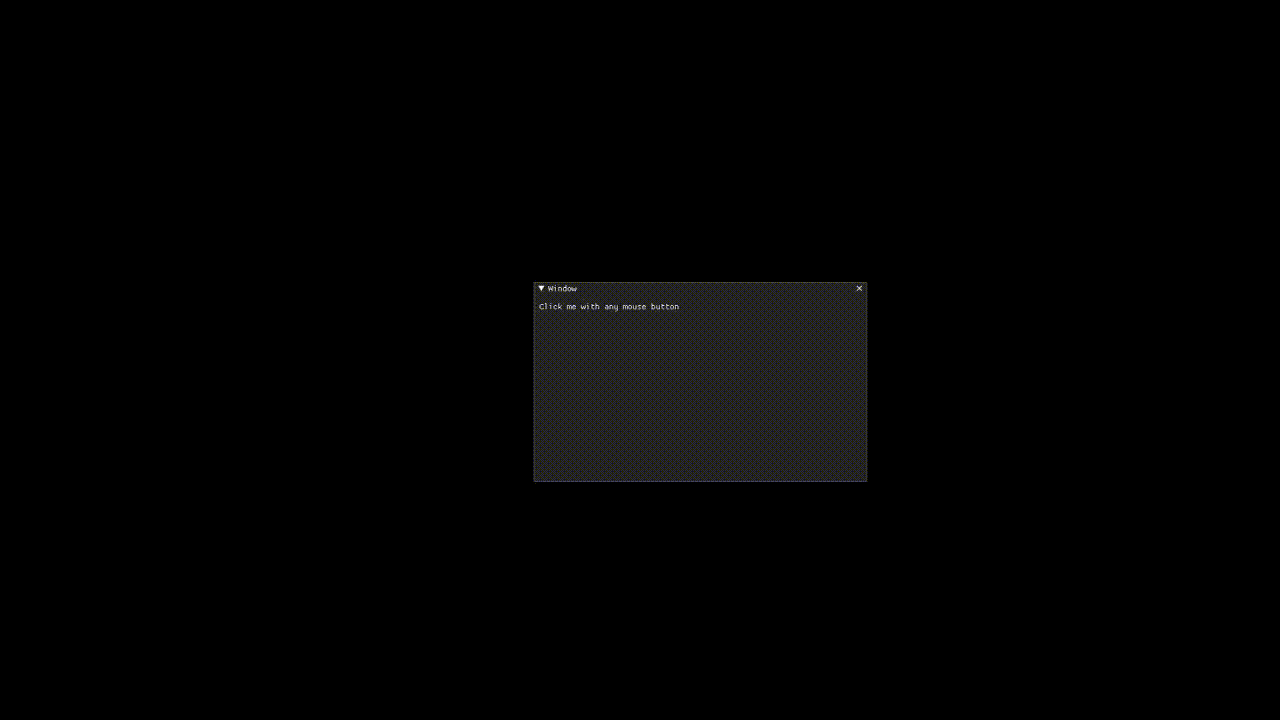
全局处理程序(IO输入)¶
全局处理程序侦听未绑定到特定项的操作:
- 键:
降下来
按下
发布
- 鼠标:
单击
双击
降下来
拖曳
移动
发布
车轮
需要将全局处理程序添加到处理程序注册表。
注册表为处理程序提供了分组方面,允许通过输入设备进行分离。它们还提供打开和关闭整个注册表的功能。
例如,这可以允许鼠标输入注册表或键盘输入注册表。注册表还允许通过简单地隐藏注册表来停用其所有子处理程序。
import dearpygui.dearpygui as dpg
dpg.create_context()
def change_text(sender, app_data):
dpg.set_value("text_item", f"Mouse Button: {app_data[0]}, Down Time: {app_data[1]} seconds")
with dpg.handler_registry():
dpg.add_mouse_down_handler(callback=change_text)
with dpg.window(width=500, height=300):
dpg.add_text("Press any mouse button", tag="text_item")
dpg.create_viewport(title='Custom Title', width=800, height=600)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()
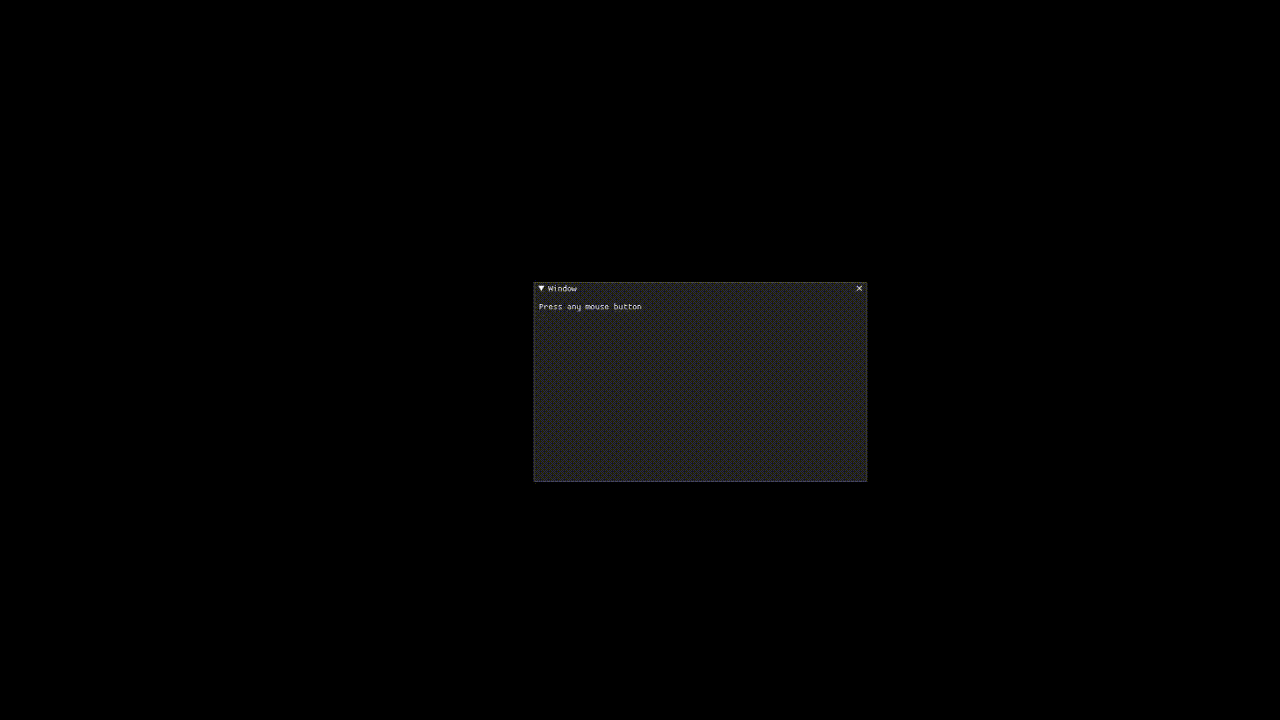
轮询项目状态¶
轮询项状态可通过 get_item_state
。当与如下所示的处理程序结合使用时,这些功能可能非常强大。
import dearpygui.dearpygui as dpg
dpg.create_context()
def change_text(sender, app_data):
if dpg.is_item_hovered("text item"):
dpg.set_value("text item", f"Stop Hovering Me, Go away!!")
else:
dpg.set_value("text item", f"Hover Me!")
with dpg.handler_registry():
dpg.add_mouse_move_handler(callback=change_text)
with dpg.window(width=500, height=300):
dpg.add_text("Hover Me!", tag="text item")
dpg.create_viewport(title='Custom Title', width=800, height=600)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()