注解
Click here 下载完整的示例代码
轮廓演示¶
说明简单的轮廓绘制,轮廓的颜色条图像上的轮廓,以及标记的轮廓。
使用默认颜色创建带有标签的简单等高线图。clabel的inline参数将控制标签是否绘制在等高线的线段上,从而删除标签下面的线。
fig, ax = plt.subplots()
CS = ax.contour(X, Y, Z)
ax.clabel(CS, inline=True, fontsize=10)
ax.set_title('Simplest default with labels')
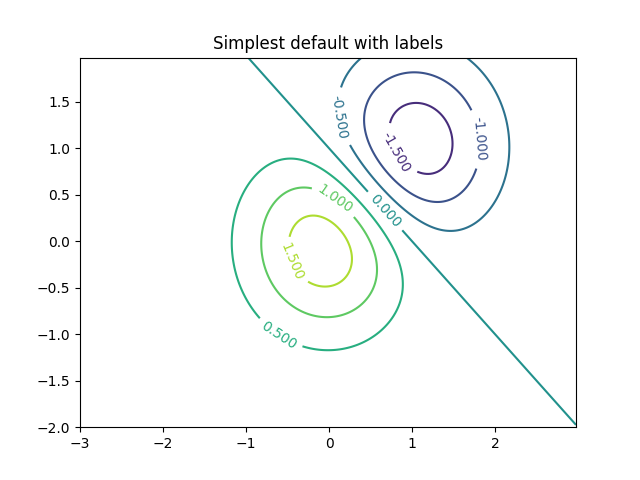
出:
Text(0.5, 1.0, 'Simplest default with labels')
通过提供位置列表(在数据坐标中),可以手动放置等高线标签。看到了吗 交互功能 用于交互式放置。
fig, ax = plt.subplots()
CS = ax.contour(X, Y, Z)
manual_locations = [
(-1, -1.4), (-0.62, -0.7), (-2, 0.5), (1.7, 1.2), (2.0, 1.4), (2.4, 1.7)]
ax.clabel(CS, inline=True, fontsize=10, manual=manual_locations)
ax.set_title('labels at selected locations')
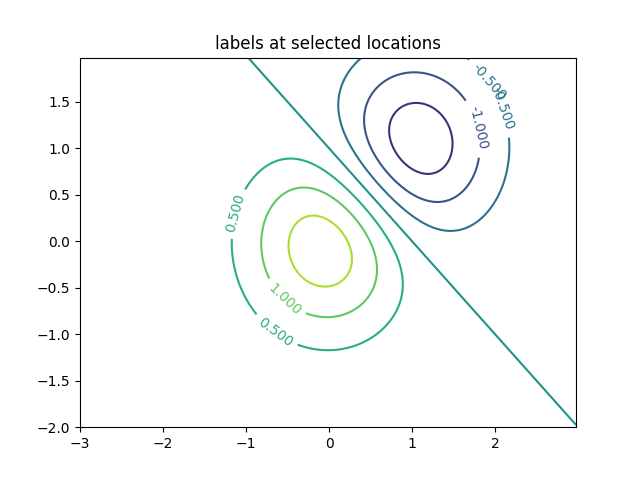
出:
Text(0.5, 1.0, 'labels at selected locations')
您可以强制所有轮廓为相同的颜色。
fig, ax = plt.subplots()
CS = ax.contour(X, Y, Z, 6, colors='k') # Negative contours default to dashed.
ax.clabel(CS, fontsize=9, inline=True)
ax.set_title('Single color - negative contours dashed')
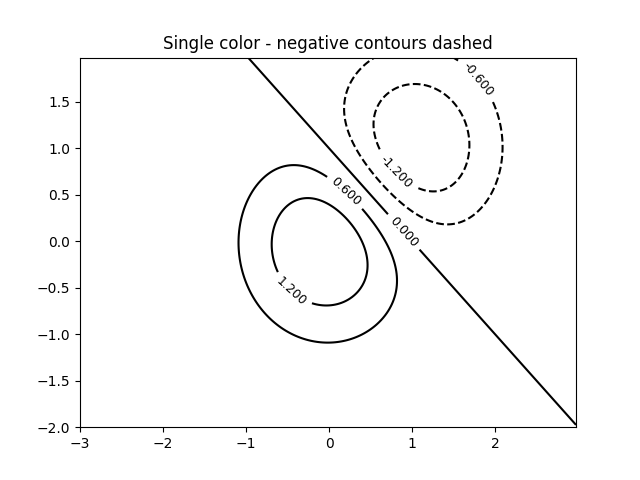
出:
Text(0.5, 1.0, 'Single color - negative contours dashed')
可以将负轮廓设置为实线而不是虚线:
matplotlib.rcParams['contour.negative_linestyle'] = 'solid'
fig, ax = plt.subplots()
CS = ax.contour(X, Y, Z, 6, colors='k') # Negative contours default to dashed.
ax.clabel(CS, fontsize=9, inline=True)
ax.set_title('Single color - negative contours solid')
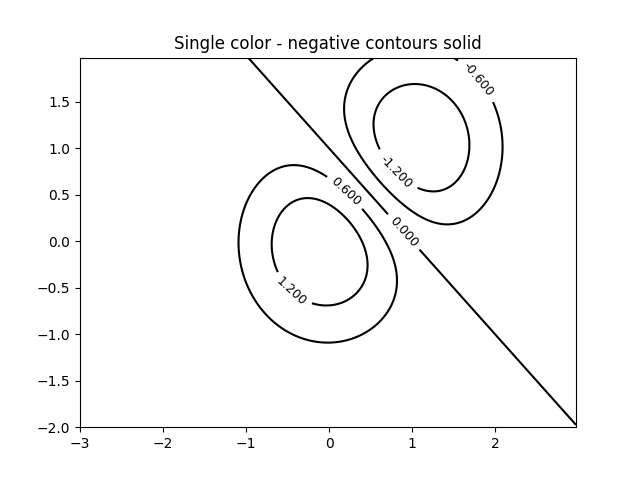
出:
Text(0.5, 1.0, 'Single color - negative contours solid')
您可以手动指定轮廓的颜色
fig, ax = plt.subplots()
CS = ax.contour(X, Y, Z, 6,
linewidths=np.arange(.5, 4, .5),
colors=('r', 'green', 'blue', (1, 1, 0), '#afeeee', '0.5'),
)
ax.clabel(CS, fontsize=9, inline=True)
ax.set_title('Crazy lines')
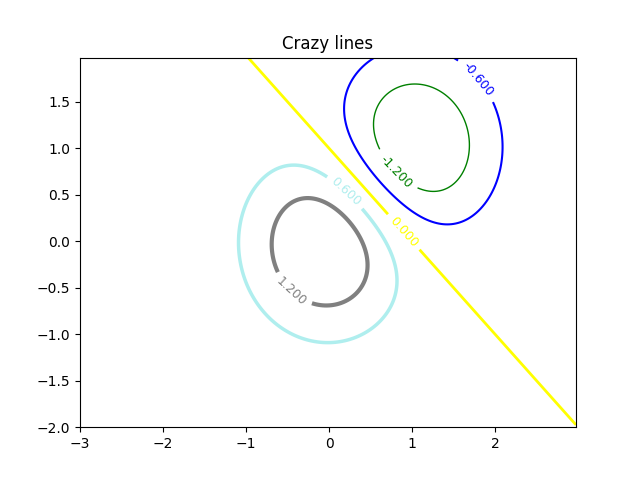
出:
Text(0.5, 1.0, 'Crazy lines')
或者可以使用颜色映射来指定颜色;默认颜色映射将用于轮廓线。
fig, ax = plt.subplots()
im = ax.imshow(Z, interpolation='bilinear', origin='lower',
cmap=cm.gray, extent=(-3, 3, -2, 2))
levels = np.arange(-1.2, 1.6, 0.2)
CS = ax.contour(Z, levels, origin='lower', cmap='flag', extend='both',
linewidths=2, extent=(-3, 3, -2, 2))
# Thicken the zero contour.
zc = CS.collections[6]
plt.setp(zc, linewidth=4)
ax.clabel(CS, levels[1::2], # label every second level
inline=True, fmt='%1.1f', fontsize=14)
# make a colorbar for the contour lines
CB = fig.colorbar(CS, shrink=0.8)
ax.set_title('Lines with colorbar')
# We can still add a colorbar for the image, too.
CBI = fig.colorbar(im, orientation='horizontal', shrink=0.8)
# This makes the original colorbar look a bit out of place,
# so let's improve its position.
l, b, w, h = ax.get_position().bounds
ll, bb, ww, hh = CB.ax.get_position().bounds
CB.ax.set_position([ll, b + 0.1*h, ww, h*0.8])
plt.show()
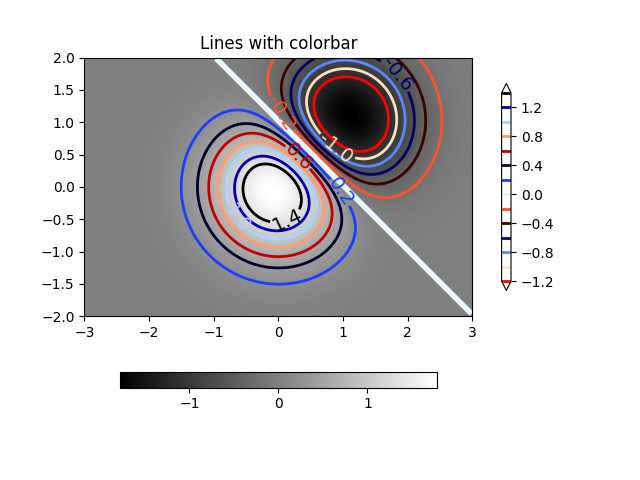
工具书类¶
本例中显示了以下函数和方法的使用:
出:
<function _AxesBase.get_position at 0x7faa00da27b8>
脚本的总运行时间: (0分3.005秒)
关键词:matplotlib代码示例,codex,python plot,pyplot Gallery generated by Sphinx-Gallery