备注
单击 here 下载完整的示例代码或通过活页夹在浏览器中运行此示例
使灰度滤镜适应RGB图像¶
有许多滤镜是为处理灰度图像而非彩色图像而设计的。为了简化创建能够适应RGB图像的函数的过程,SCRICIT-IMAGE提供了 adapt_rgb
装饰师。
要实际使用 adapt_rgb
装饰者,您必须决定如何调整RGB图像以使用灰度滤镜。有两个预定义的处理程序:
each_channel
将每个RGB通道逐个传递给滤镜,并将结果缝合回RGB图像。
hsv_value
将RGB图像转换为HSV并将值通道传递给滤镜。过滤后的结果被插入回HSV图像中,并转换回RGB。
下面,我们演示如何使用 adapt_rgb
在几个灰度滤镜上:
我们可以像平时一样使用这些函数,但现在它们既可以处理灰度图像,也可以处理彩色图像。让我们用彩色图像绘制结果:
from skimage import data
from skimage.exposure import rescale_intensity
import matplotlib.pyplot as plt
image = data.astronaut()
fig, (ax_each, ax_hsv) = plt.subplots(ncols=2, figsize=(14, 7))
# We use 1 - sobel_each(image) but this won't work if image is not normalized
ax_each.imshow(rescale_intensity(1 - sobel_each(image)))
ax_each.set_xticks([]), ax_each.set_yticks([])
ax_each.set_title("Sobel filter computed\n on individual RGB channels")
# We use 1 - sobel_hsv(image) but this won't work if image is not normalized
ax_hsv.imshow(rescale_intensity(1 - sobel_hsv(image)))
ax_hsv.set_xticks([]), ax_hsv.set_yticks([])
ax_hsv.set_title("Sobel filter computed\n on (V)alue converted image (HSV)")
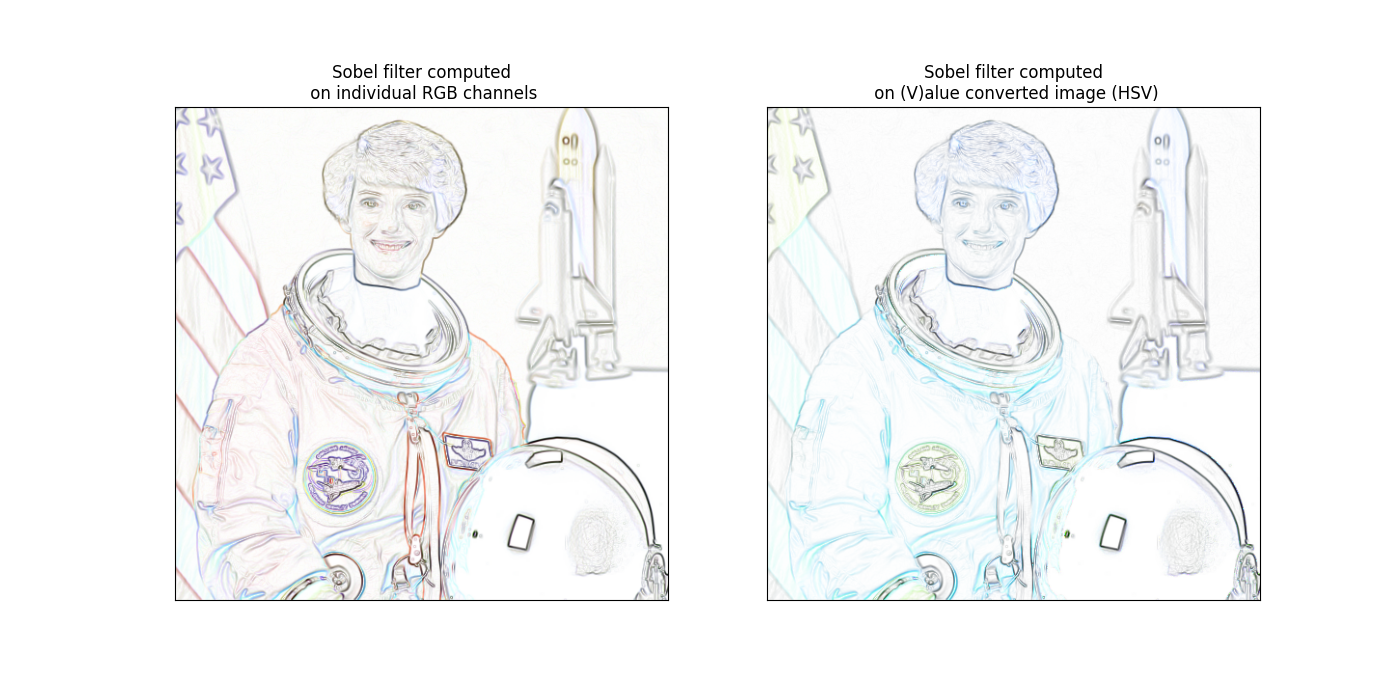
输出:
Text(0.5, 1.0, 'Sobel filter computed\n on (V)alue converted image (HSV)')
请注意,值过滤图像的结果保留了原始图像的颜色,但通道过滤图像以更令人惊讶的方式组合在一起。在其他常见情况下,例如平滑,通道过滤图像将产生比值过滤图像更好的结果。
您还可以为创建自己的处理程序函数 adapt_rgb
。为此,只需创建一个具有以下签名的函数:
请注意, adapt_rgb
处理程序是为图像是第一个参数的筛选器编写的。
作为一个非常简单的示例,我们只需将任何RGB图像转换为灰度,然后返回过滤结果:
创建使用以下内容的签名非常重要 *args
和 **kwargs
将参数传递给筛选器,以便允许修饰函数具有任意数量的位置参数和关键字参数。
最后,我们可以将该处理程序用于 adapt_rgb
就像以前一样:
@adapt_rgb(as_gray)
def sobel_gray(image):
return filters.sobel(image)
fig, ax = plt.subplots(ncols=1, nrows=1, figsize=(7, 7))
# We use 1 - sobel_gray(image) but this won't work if image is not normalized
ax.imshow(rescale_intensity(1 - sobel_gray(image)), cmap=plt.cm.gray)
ax.set_xticks([]), ax.set_yticks([])
ax.set_title("Sobel filter computed\n on the converted grayscale image")
plt.show()
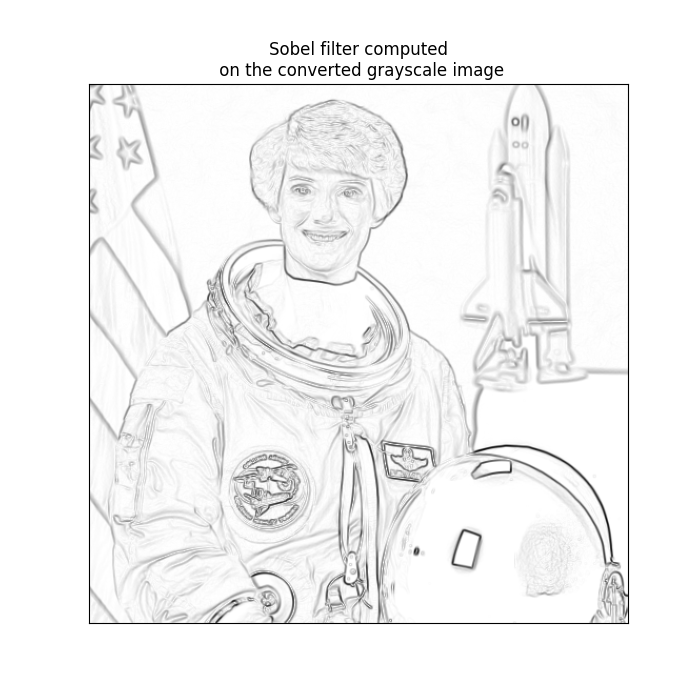
备注
对阵列形状的非常简单的检查用于检测RGB图像,因此 adapt_rgb
不建议用于支持非RGB空间中的3D体积或彩色图像的功能。
脚本的总运行时间: (0分0.693秒)