XML解析¶
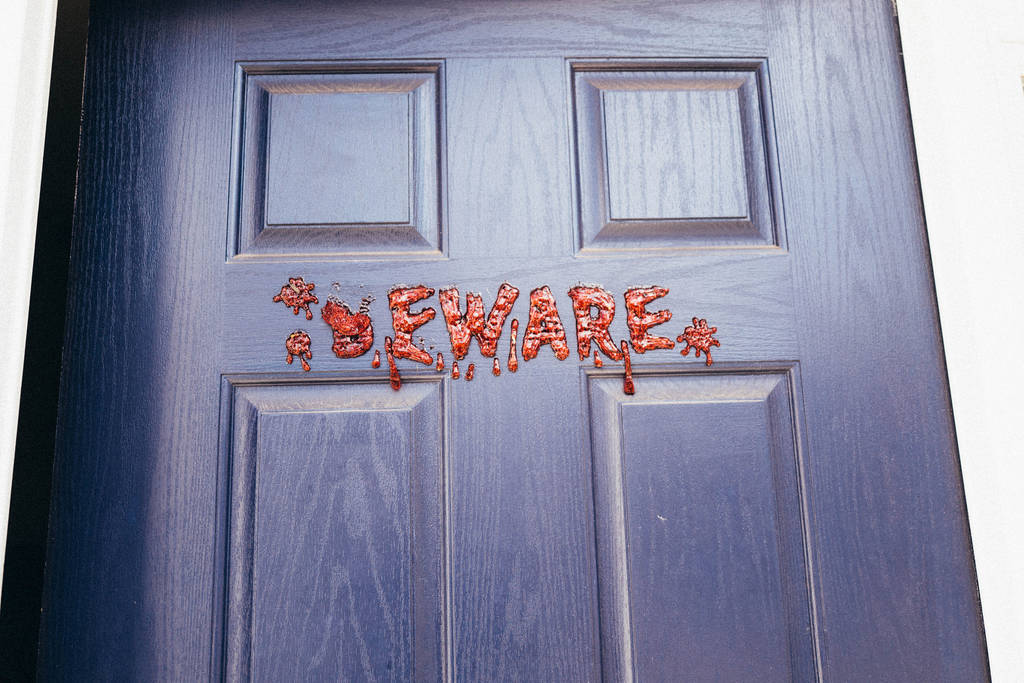
untangle¶
untangle 是一个简单的库,它接受一个XML文档并返回一个反映其结构中节点和属性的python对象。
例如,这样的XML文件:
<?xml version="1.0"?>
<root>
<child name="child1">
</root>
可以这样加载:
import untangle
obj = untangle.parse('path/to/file.xml')
然后您可以像这样获得子元素的name属性:
obj.root.child['name']
untangle还支持从字符串或URL加载XML。
xmltodict¶
xmltodict 是另一个简单的库,旨在让XML看起来像是在使用JSON。
这样的XML文件:
<mydocument has="an attribute">
<and>
<many>elements</many>
<many>more elements</many>
</and>
<plus a="complex">
element as well
</plus>
</mydocument>
可以这样加载到Python dict中:
import xmltodict
with open('path/to/file.xml') as fd:
doc = xmltodict.parse(fd.read())
然后您可以访问元素、属性和值,如下所示:
doc['mydocument']['@has'] # == u'an attribute'
doc['mydocument']['and']['many'] # == [u'elements', u'more elements']
doc['mydocument']['plus']['@a'] # == u'complex'
doc['mydocument']['plus']['#text'] # == u'element as well'
xmltodict还允许您使用unparse函数往返于XML,具有适合处理内存中不适合的文件的流模式,并支持XML命名空间。
XMLSchema¶
xmlschema 提供对在Python中使用XSD架构的支持。与其他XML库不同,自动类型解析是可用的,因此F.E.如果架构将元素定义为 int
,被解析的 dict
还将包含一个 int
该元素的值。此外,该库支持根据模式自动和显式地验证XML文档。
from xmlschema import XMLSchema, etree_tostring
# load a XSD schema file
schema = XMLSchema("your_schema.xsd")
# validate against the schema
schema.validate("your_file.xml")
# or
schema.is_valid("your_file.xml")
# decode a file
data = schmema.decode("your_file.xml")
# encode to string
s = etree_tostring(schema.encode(data))